Improving Performance of Play 2 Modules
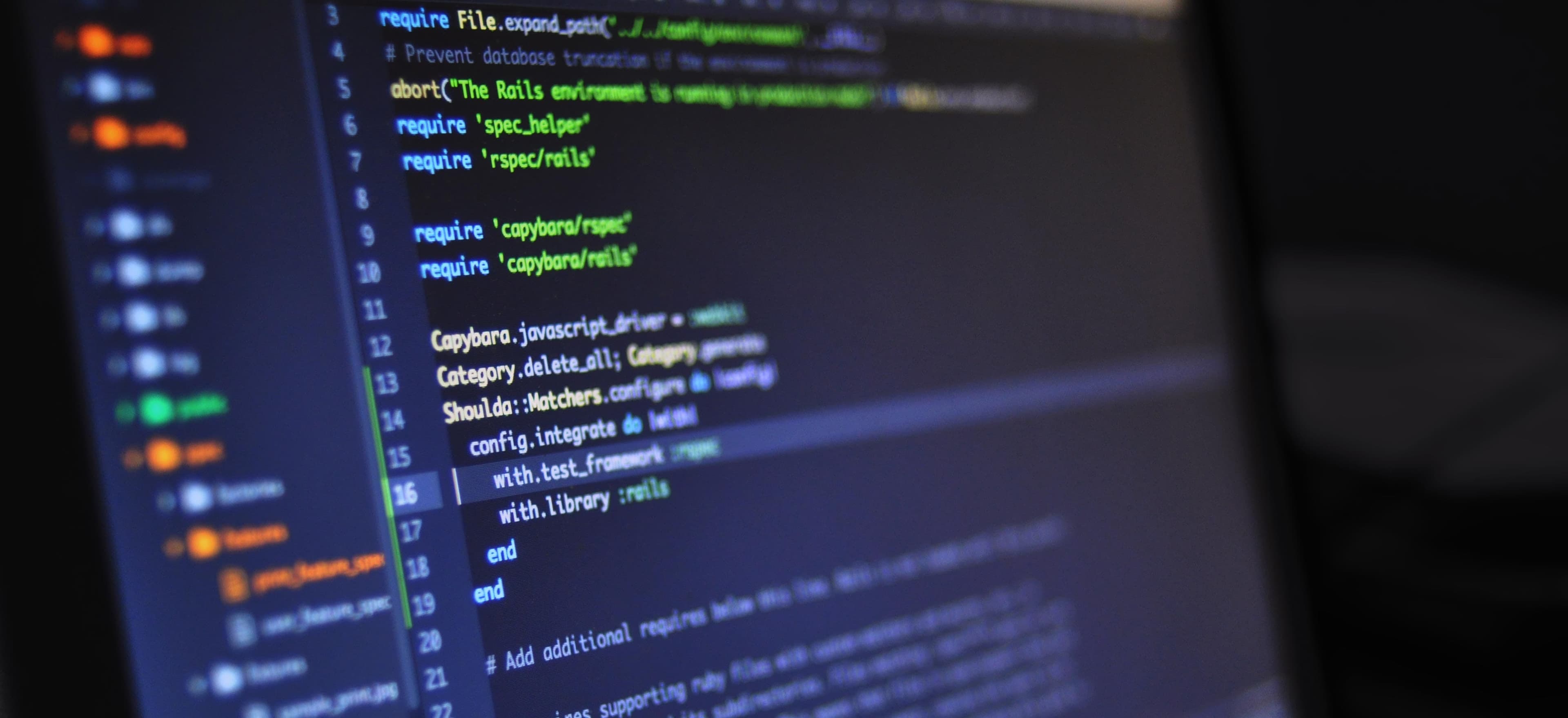
- Published on
Maximizing Performance in Play 2 Modules
Play Framework has established itself as a robust and efficient web framework for Java and Scala developers. However, when working with Play 2 modules, ensuring optimal performance can be a challenging task.
In this post, we will explore some best practices and techniques to maximize the performance of Play 2 modules. We will delve into areas such as caching, asynchronous processing, and optimizing database interactions to ensure your modules perform at their best.
Caching for Improved Response Times
Caching is a crucial aspect of performance optimization in Play 2 modules. By caching frequently accessed data, you can reduce the load on your application and improve response times.
Play Framework provides a powerful caching API that allows you to cache the results of expensive computations or database queries. Let's take a look at an example of how caching can be utilized in a Play 2 module.
public Result index() {
String cachedData = cache.getOrElse("cachedData", () -> {
// Expensive computation or database query
return fetchDataFromDatabase();
});
return ok(cachedData);
}
In this example, the cache.getOrElse
method is used to retrieve data from the cache. If the data is not found, the provided function is executed to fetch the data from the database, which is then stored in the cache for future requests.
By incorporating caching in this manner, you can significantly improve the response times of your Play 2 modules.
Leveraging Asynchronous Processing
Asynchronous processing is another technique that can greatly enhance the performance of Play 2 modules, especially when dealing with I/O operations. With Play's built-in support for asynchronous programming, you can leverage non-blocking, event-driven code to handle concurrent requests efficiently.
Let's consider an example of using asynchronous processing to perform parallel database queries in a Play 2 module.
public CompletionStage<Result> getUsers() {
CompletionStage<List<User>> usersFuture = userRepository.getUsers();
CompletionStage<List<Role>> rolesFuture = roleRepository.getRoles();
return usersFuture.thenCombineAsync(rolesFuture, (users, roles) -> {
// Process and combine the results
return ok("Users and roles fetched successfully");
});
}
In this example, the getUsers
method fetches users and roles from the database asynchronously using the userRepository
and roleRepository
. The thenCombineAsync
method is then used to process the results of both queries and combine them before returning the response.
By embracing asynchronous processing, you can effectively utilize the resources of your Play 2 modules and cater to a larger number of concurrent requests without compromising performance.
Optimizing Database Interactions
Efficient interaction with databases is crucial for the overall performance of Play 2 modules. By employing techniques such as connection pooling, query optimization, and proper indexing, you can significantly enhance the responsiveness of your modules.
Play Framework offers seamless integration with popular database libraries such as Slick or Anorm, allowing you to optimize database interactions at various levels. Let's examine a succinct example of how connection pooling can be configured in a Play 2 module.
db.default.hikaricp.connectionTimeout = 30 seconds
db.default.hikaricp.maximumPoolSize = 10
In this configuration, the HikariCP connection pool settings are customized to define the connection timeout and maximum pool size. This allows for better utilization of database connections and efficient management of resources, ultimately contributing to improved performance.
Furthermore, utilizing database-specific features such as query optimizations and index utilization can also significantly enhance the efficiency of database interactions in Play 2 modules.
Final Thoughts
In conclusion, maximizing the performance of Play 2 modules necessitates a comprehensive approach that encompasses caching, asynchronous processing, and optimized database interactions. By strategically incorporating these techniques, you can ensure that your modules deliver optimal performance and responsiveness to meet the demands of modern web applications.
For further insights into Play Framework and performance optimization, consider exploring Play Framework's official documentation and best practices for Java web development to expand your knowledge and proficiency in building high-performance applications.
Optimizing the performance of Play 2 modules is an ongoing endeavor, and staying abreast of best practices and advancements in web development is essential to consistently deliver efficient and scalable solutions.