Troubleshooting Async Method Invocation with Future in Spring
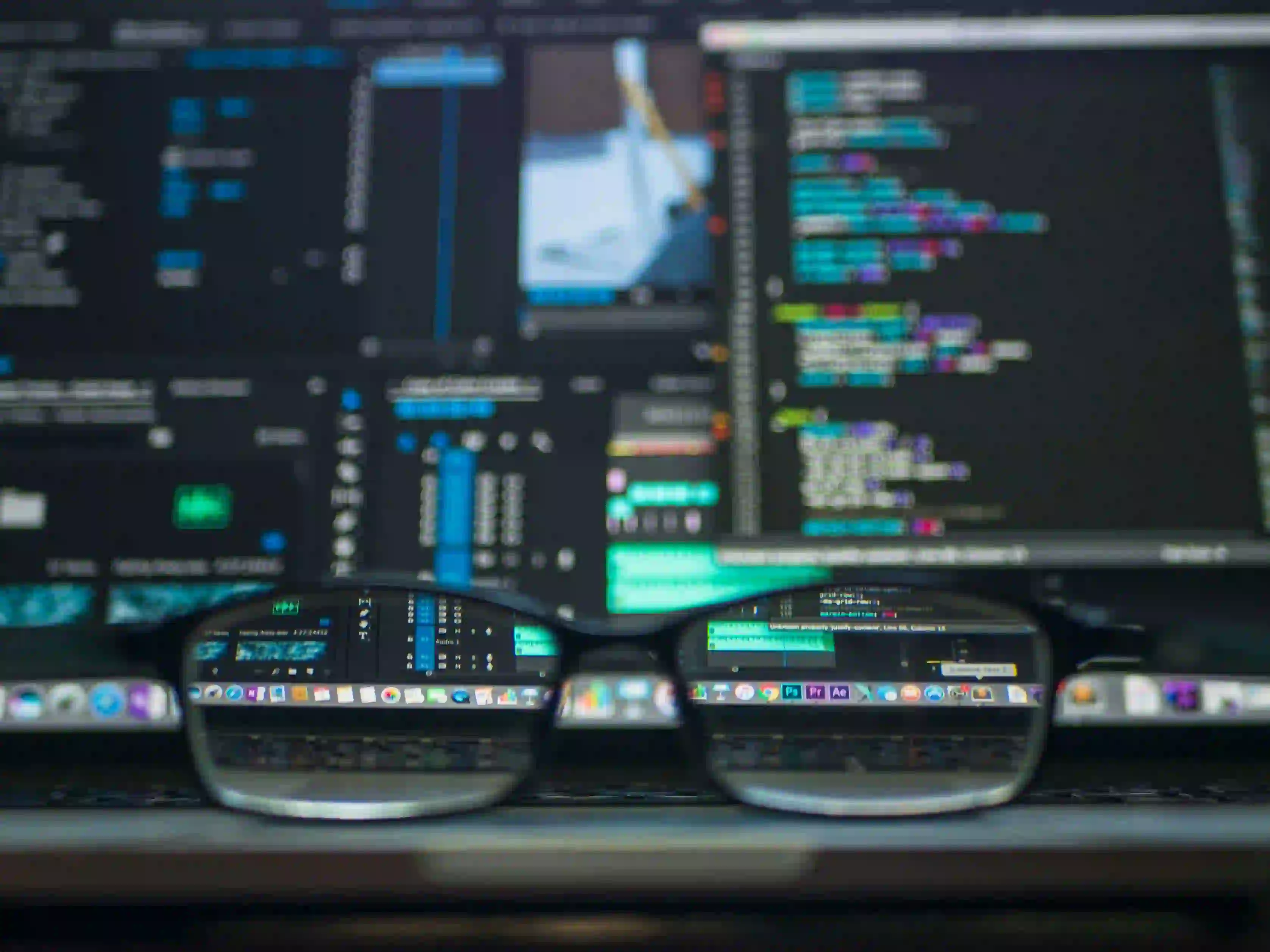
Troubleshooting Async Method Invocation with Future in Spring
Asynchronous programming is a vital part of modern software development, allowing developers to improve performance and responsiveness by executing non-blocking operations. In Java, the Future
interface has long been used to represent the result of an asynchronous computation. When working with Spring Framework, developers often leverage the @Async
annotation to mark a method as asynchronous, allowing it to be executed in a separate thread.
However, troubleshooting issues that arise from async method invocation with Future
in Spring can be challenging. This article aims to explore common problems and their solutions, equipping you with the knowledge to navigate and resolve such issues effectively.
Understanding Async Method Invocation in Spring
Before delving into troubleshooting, it's crucial to understand the basics of async method invocation in Spring. When you annotate a method with @Async
, Spring creates a proxy that is capable of intercepting the method call and executing it in a separate thread. The method returns a Future
object, which allows the caller to obtain the result or handle potential exceptions once the asynchronous task is completed.
Consider the following example, where a service method is annotated with @Async
:
@Service
public class MyService {
@Async
public Future<String> performAsyncTask() {
// Perform asynchronous task
return new AsyncResult<>("Task completed successfully");
}
}
In this scenario, the performAsyncTask
method is executed asynchronously, and a Future
containing the result of the task is returned to the caller.
Troubleshooting Common Issues
1. Missing @EnableAsync Annotation
When using the @Async
annotation in Spring, it's essential to ensure that the @EnableAsync
annotation is present in the configuration. This annotation enables the detection of @Async
annotations and allows for the creation of the necessary proxies.
@Configuration
@EnableAsync
public class AppConfig {
// Configuration beans and other settings
}
Without the @EnableAsync
annotation, the @Async
annotation will be ignored, and the method will be executed synchronously, leading to unexpected behavior.
2. Uncaught Exception Handling
Handling exceptions from asynchronous methods is crucial, as failing to do so can result in silent failures and unprocessed errors. When using Future
to obtain the result of an asynchronous task, it's essential to handle exceptions that may occur during the task's execution.
@Service
public class MyService {
@Async
public Future<String> performAsyncTask() {
try {
// Perform asynchronous task
return new AsyncResult<>("Task completed successfully");
} catch (Exception e) {
return new AsyncResult<>("Task failed: " + e.getMessage());
}
}
}
In this example, an exception within the asynchronous task is caught, and a relevant error message is returned within the AsyncResult
object.
3. Using CompletableFuture Instead of Future
While the Future
interface provides basic functionality for asynchronous computation, Java 8 introduced the CompletableFuture
class, offering more advanced features for handling asynchronous operations. Consider using CompletableFuture
in place of Future
to take advantage of its extensive capabilities, such as combining multiple asynchronous tasks and handling timeouts.
@Service
public class MyService {
@Async
public CompletableFuture<String> performAsyncTask() {
// Perform asynchronous task
return CompletableFuture.completedFuture("Task completed successfully");
}
}
By utilizing CompletableFuture
, you can enhance the management and composition of asynchronous tasks within your Spring application.
Key Takeaways
In conclusion, troubleshooting async method invocation with Future
in Spring involves understanding the nuances of asynchronous programming and addressing common pitfalls. By ensuring the presence of @EnableAsync
, handling exceptions effectively, and considering the use of CompletableFuture
, you can enhance the reliability and performance of asynchronous tasks in your Spring applications.
Remember, thorough understanding of the underlying principles, combined with diligent troubleshooting, is key to effectively resolving issues related to async method invocation with Future
in Spring.
For further insights into asynchronous programming with Spring, refer to the official documentation provided by the Spring team.
I hope this guide has been beneficial in improving your troubleshooting skills for async method invocation in Spring!