The Ultimate Guide to Implementing ListViews with Adapters in Android
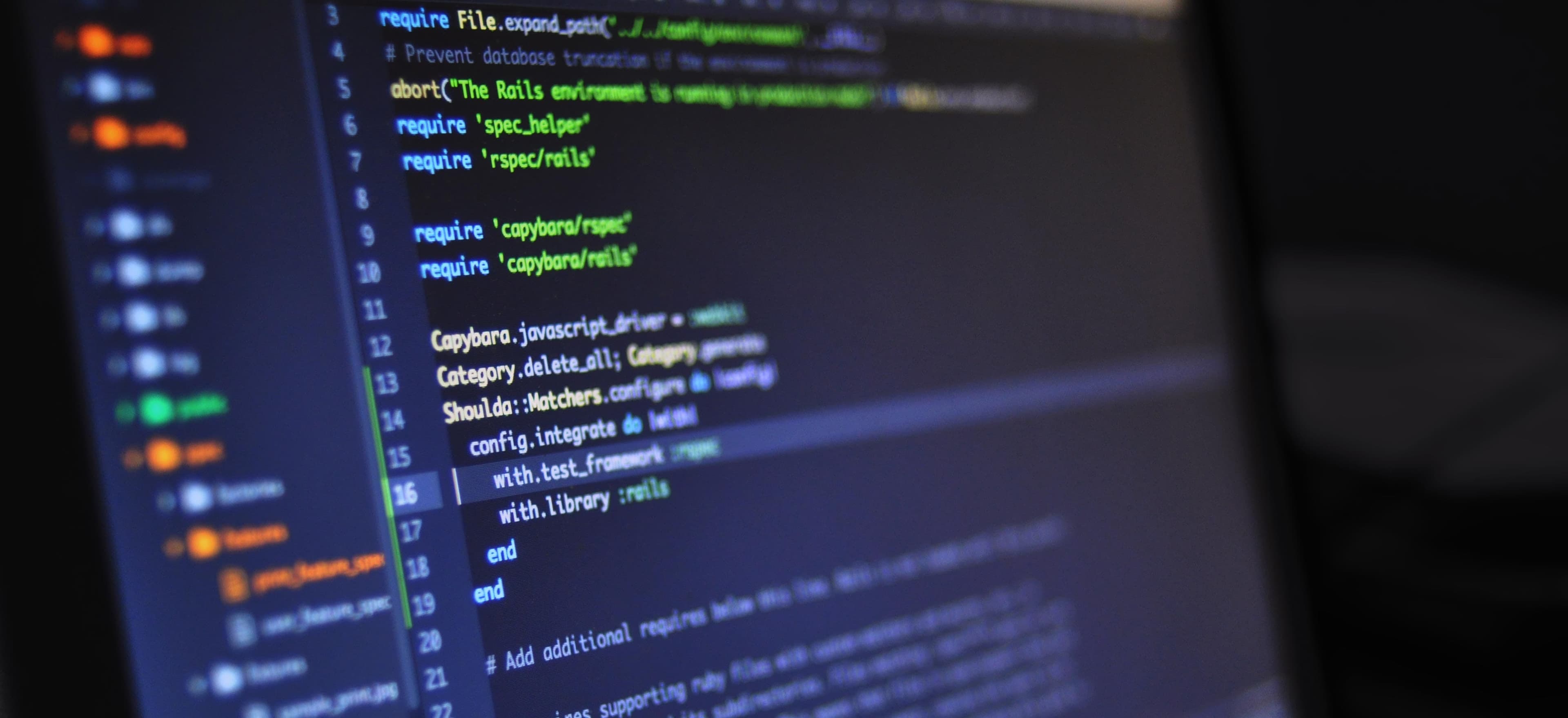
- Published on
The Ultimate Guide to Implementing ListViews with Adapters in Android
If you're a mobile app developer working with Java and Android, you've probably encountered the need to display a list of items in your app. The ListView, along with the Adapter pattern, is a powerful combination for achieving this. In this guide, we'll delve into the ins and outs of implementing ListViews with Adapters in Android.
Understanding ListViews and Adapters
A ListView is a view group that displays a list of scrollable items. However, to populate the ListView with data, we need to use an Adapter. An Adapter acts as a bridge between the data source and the ListView, providing the necessary view for each item in the data set.
Setting up the ListView in XML
Let's start by setting up a ListView in our layout XML file:
<ListView
android:id="@+id/listView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
Creating the Custom Adapter
In order to customize the appearance and behavior of items within the ListView, we need to create a custom Adapter by extending the BaseAdapter
class. Let's go through an example of how to achieve this:
public class CustomAdapter extends BaseAdapter {
private List<String> dataList;
private LayoutInflater inflater;
public CustomAdapter(Context context, List<String> dataList) {
this.dataList = dataList;
this.inflater = LayoutInflater.from(context);
}
@Override
public int getCount() {
return dataList.size();
}
@Override
public String getItem(int position) {
return dataList.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
if (convertView == null) {
convertView = inflater.inflate(R.layout.list_item, parent, false);
}
TextView textView = convertView.findViewById(R.id.textView);
textView.setText(getItem(position));
return convertView;
}
}
In the above example, we've created a CustomAdapter
that takes a list of strings as its data source. We override the necessary methods such as getCount
, getItem
, getItemId
, and getView
to customize the behavior and appearance of the ListView's items.
Using the Custom Adapter with the ListView
Now that we have our custom Adapter set up, let's use it to populate the ListView in our MainActivity.
public class MainActivity extends AppCompatActivity {
private ListView listView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView = findViewById(R.id.listView);
List<String> dataList = Arrays.asList("Item 1", "Item 2", "Item 3");
CustomAdapter customAdapter = new CustomAdapter(this, dataList);
listView.setAdapter(customAdapter);
}
}
In the onCreate
method of our MainActivity, we initialize the ListView and create an instance of our CustomAdapter
, passing in the data list. We then set the customAdapter to the ListView using the setAdapter
method.
Benefits of Using Adapters
By implementing ListViews with Adapters in Android, we gain the following benefits:
- Efficient use of memory by recycling views as the user scrolls through the list.
- Simplified data population and presentation within the ListView.
- Easy customization of item appearance and behavior by creating custom Adapters.
The Bottom Line
In this guide, we've explored the implementation of ListViews with Adapters in Android using Java. Understanding the role of Adapters in populating ListViews and creating custom Adapters is essential for building responsive and dynamic user interfaces in Android apps.
By following the steps outlined in this guide, you can effectively utilize ListViews and Adapters to seamlessly display lists of items in your Android applications, providing a smooth and engaging user experience.
Implementing ListViews with Adapters in Android is a fundamental skill for any Android developer, and mastering this concept will empower you to create rich, interactive, and user-friendly apps.
Now that you've grasped the fundamentals of ListViews and Adapters, you're well-equipped to take your Android development skills to the next level!
Keep coding and building amazing Android apps!
Check out these resources for further learning:
Happy coding!