Maximizing Efficiency in Automation Testing Pipelines
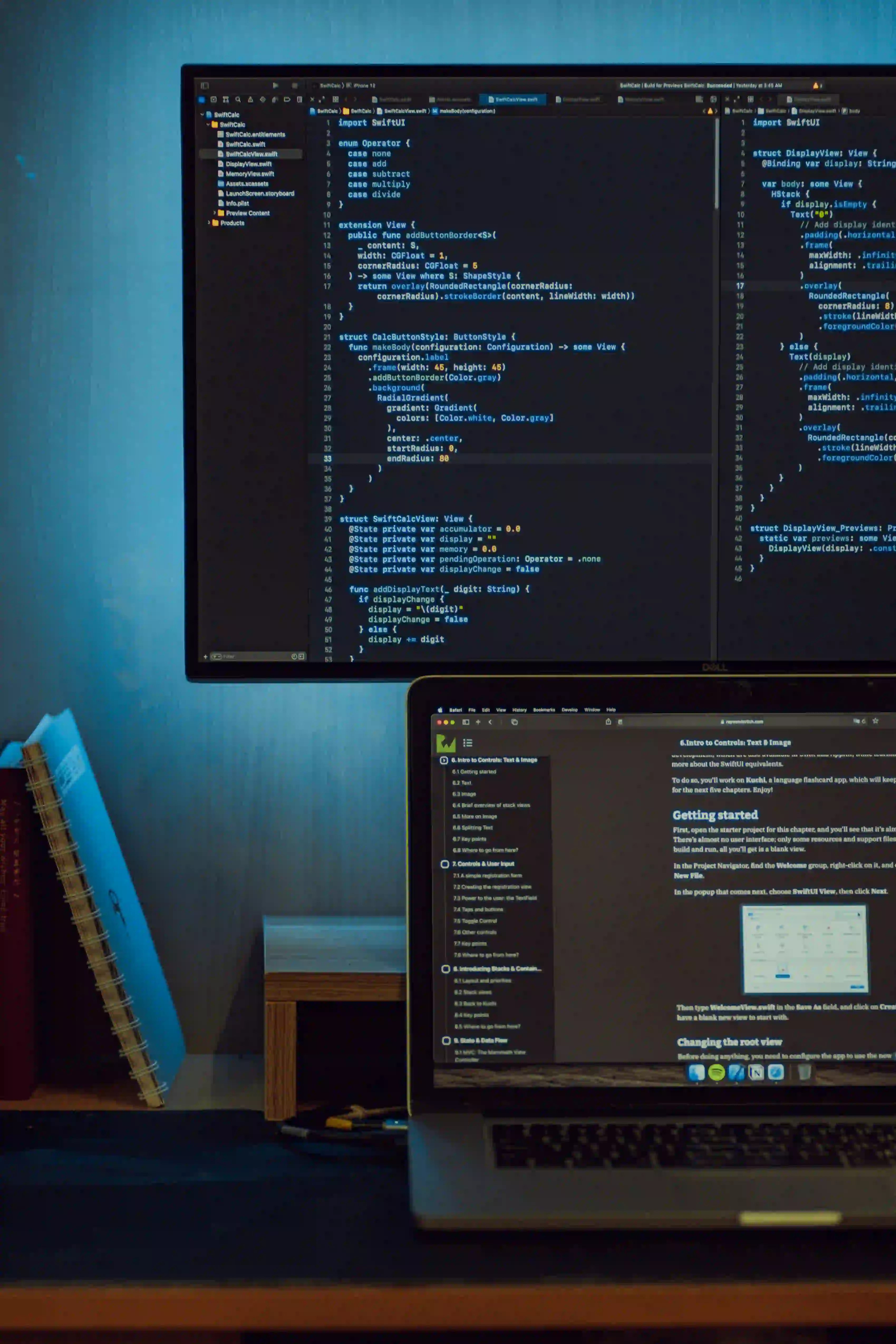
Understanding the Importance of Automation Testing in Software Development
In today's dynamic software development landscape, the need for automated testing is more critical than ever. Automation testing has become an integral part of the software development lifecycle, ensuring the quality, reliability, and efficiency of software applications. With the rapid adoption of Agile and DevOps practices, the demand for continuous integration and deployment has skyrocketed, making automation testing an indispensable component of the development process.
Embracing Automation Testing in Java
Java has emerged as a leading programming language for automation testing due to its versatility, robustness, and rich ecosystem of testing frameworks and libraries. Leveraging Java for automation testing allows developers to create scalable, maintainable, and efficient test suites that can seamlessly integrate with existing development pipelines. In this article, we'll explore tactics and best practices for maximizing efficiency in automation testing pipelines using Java.
Choosing the Right Testing Framework
Selecting the appropriate testing framework is pivotal in ensuring the effectiveness and efficiency of automation testing pipelines. In the Java ecosystem, several testing frameworks such as JUnit, TestNG, and Spock offer diverse features and capabilities to cater to different testing needs.
Let's consider a simple example using JUnit, a widely adopted testing framework in the Java community. Below is a basic JUnit test class for testing a hypothetical Calculator
class:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In the above code snippet, we define a test method testAddition()
that verifies the functionality of the add
method in the Calculator
class. The assertEquals
assertion compares the expected result with the actual result, ensuring the correctness of the operation. This simplistic yet powerful example illustrates the elegance of JUnit for writing concise and effective test cases.
Implementing Page Object Model Design Pattern
When it comes to automated UI testing, the Page Object Model (POM) design pattern plays a pivotal role in enhancing the maintainability and reusability of test code. By encapsulating the web page elements and their interactions within dedicated page classes, POM promotes clean and organized test code, reducing duplication and improving the robustness of UI automation tests.
Let's delve into a simplified POM implementation using Selenium, a popular automation tool for web application testing. Below is an example of a page class representing a login page:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
public class LoginPage {
private WebDriver driver;
private By usernameInput = By.id("username");
private By passwordInput = By.id("password");
private By loginButton = By.id("loginButton");
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
driver.findElement(usernameInput).sendKeys(username);
}
public void enterPassword(String password) {
driver.findElement(passwordInput).sendKeys(password);
}
public void clickLoginButton() {
driver.findElement(loginButton).click();
}
}
The LoginPage
class encapsulates the web elements and their corresponding interactions, providing a clean and cohesive abstraction for the login page. This enables the testers to write concise and readable test scripts, promoting maintainability and scalability in UI automation testing.
Leveraging Parallel Execution
To expedite the execution of test suites and minimize the overall testing time, parallel execution is an indispensable strategy. By running test cases in parallel, automation testing pipelines can significantly reduce the time required for comprehensive test coverage, accelerating the feedback loop and enhancing the agility of the development process.
In Java, TestNG provides robust support for parallel execution, allowing test classes, test methods, or even individual test instances to run concurrently, leveraging the available computing resources effectively.
Below is an example of configuring parallel execution in TestNG:
<suite name="MyTestSuite" parallel="tests">
<test name="RegressionTests">
<classes>
<class name="com.example.testcase1"/>
<class name="com.example.testcase2"/>
</classes>
</test>
</suite>
By specifying the parallel
attribute in the TestNG suite XML file, we can orchestrate parallel execution at different levels of granularity, paving the way for expedited test runs and improved testing efficiency.
Incorporating Continuous Integration (CI) and Continuous Deployment (CD) Pipelines
Integrating automation testing seamlessly into CI/CD pipelines is paramount for enforcing the principles of continuous integration, continuous deployment, and DevOps. Tools like Jenkins, Travis CI, and CircleCI offer robust capabilities for automating the execution of test suites, ensuring that every code change undergoes rigorous testing before deployment.
By leveraging Jenkins, a popular CI/CD tool, developers and testers can orchestrate the execution of automation test scripts as part of the build and deployment process, fostering a culture of continuous quality assurance and validation.
My Closing Thoughts on the Matter
In essence, Java serves as an exceptional platform for building and optimizing automation testing pipelines, equipping developers and testers with a plethora of tools, frameworks, and best practices to maximize efficiency and effectiveness. By embracing the right testing framework, adopting proven design patterns, harnessing parallel execution, and integrating with CI/CD pipelines, organizations can cultivate a robust testing culture that accelerates the delivery of high-quality software products.
By adhering to these principles and instilling a mindset of continuous improvement, software teams can champion automation testing as a catalyst for innovation, reliability, and customer satisfaction in the ever-evolving realm of software development.