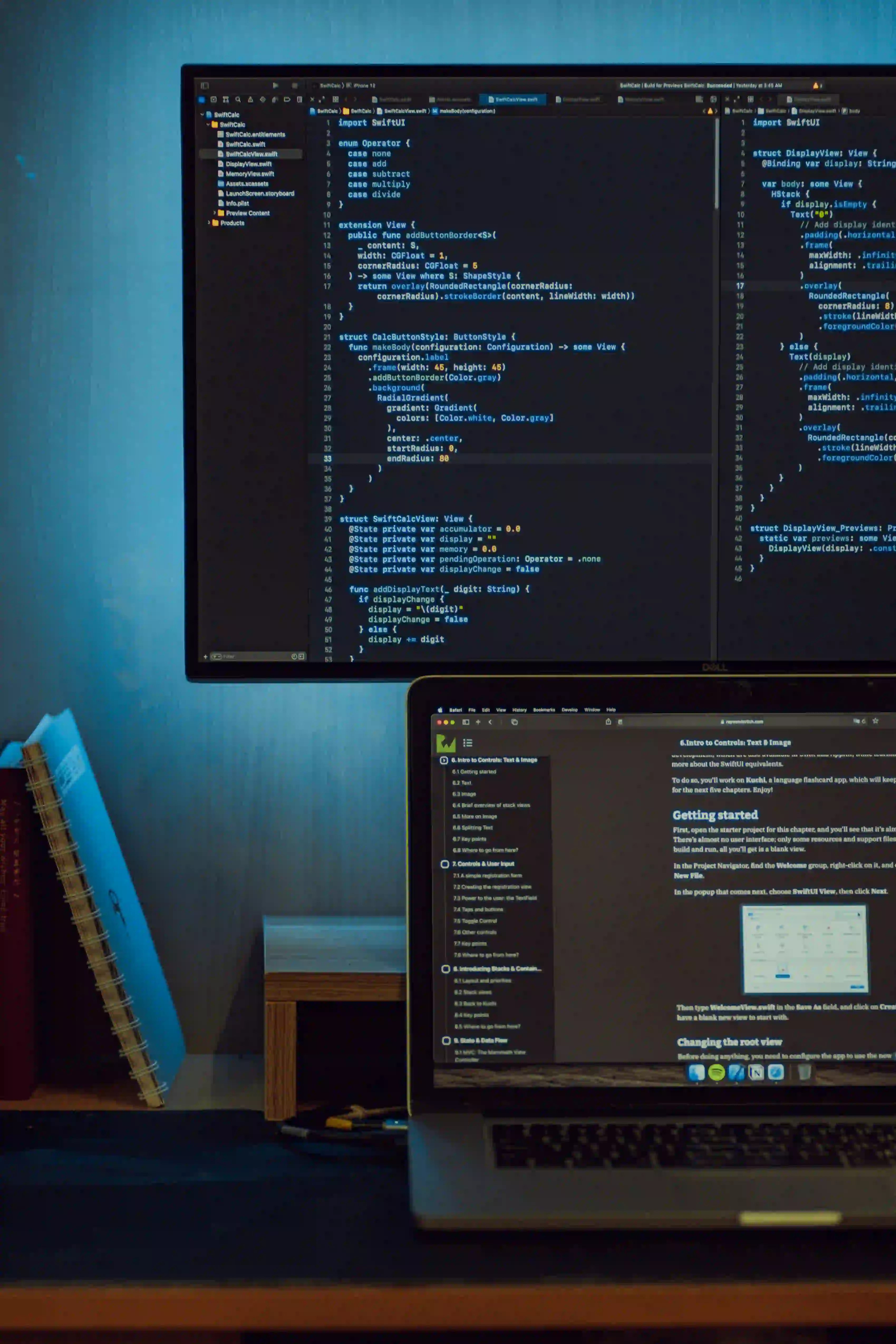
Maximizing Efficiency: Streamlining Java EE and Spring Integration
In the world of enterprise application development, Java EE and Spring Framework are two pillars that provide robust, scalable, and efficient solutions. Java Enterprise Edition (Java EE) offers a set of specifications, APIs, and runtime environments for building large-scale, multi-tiered, scalable, reliable, and secure enterprise applications. On the other hand, the Spring Framework provides a comprehensive programming and configuration model for modern Java-based enterprise applications.
Seamless Integration of Java EE and Spring Framework
When it comes to enterprise application development, integrating Java EE and Spring Framework can offer a powerful combination of features and capabilities. Java EE provides a set of standardized APIs, including servlets, JPA (Java Persistence API), JMS (Java Message Service), and JTA (Java Transaction API), while the Spring Framework simplifies the development of enterprise applications through its dependency injection, AOP (Aspect-oriented Programming), and declarative transaction management.
Leveraging the Power of CDI (Contexts and Dependency Injection)
CDI is a powerful component model that is part of the Java EE platform, which can be seamlessly integrated with the Spring Framework to benefit from the features offered by both technologies. By using CDI alongside Spring, developers can take advantage of features such as type-safe dependency injection, interceptors, decorators, events, and scopes, while also benefiting from Spring's extensive support for AspectJ aspects, declarative transaction management, and AOP.
Example of CDI and Spring Integration
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.enterprise.inject.Produces;
import javax.inject.Inject;
@Configuration
public class CDIAndSpringIntegrationConfig {
@Inject
private SomeCdiBean someCdiBean;
@Autowired
private SomeSpringBean someSpringBean;
@Bean
public SomeSpringBean someSpringBean() {
return new SomeSpringBean();
}
@Produces
public SomeCdiBean someCdiBean() {
return new SomeCdiBean();
}
}
In this example, we are demonstrating the integration of CDI and Spring through a configuration class where we are injecting a CDI bean into a Spring bean and vice versa. This seamless integration enables the utilization of both CDI and Spring beans within the same application, consolidating the best features of both technologies.
Utilizing Spring Data with JPA
The integration of Spring Data with JPA, a key component of Java EE, provides a powerful way to simplify the implementation of data access layers and repositories. Spring Data JPA offers the ability to automatically generate repository implementations at runtime from a repository interface. This eliminates the need for boilerplate code traditionally associated with the development of data access layers and repositories.
Example of Spring Data JPA Integration
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
List<User> findByRole(Role role);
// Additional custom queries and operations can be defined here
}
In this example, we are showcasing the simplicity of defining a repository interface by extending the JpaRepository
interface provided by Spring Data JPA. Additionally, custom query methods can be defined within the repository interface, allowing developers to leverage the power of JPA while benefiting from the simplifications offered by Spring Data.
Effective Transactions Management
Java EE's JTA (Java Transaction API) and Spring's transaction management capabilities can be seamlessly integrated to ensure effective management of transactions within enterprise applications. By leveraging the strengths of both Java EE and Spring Framework for transaction management, developers can ensure the reliability and consistency of their applications' data operations.
Example of Transactions Management with Spring and JTA
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
@PersistenceContext
private EntityManager entityManager;
@Transactional(rollbackFor = Exception.class)
public User updateUser(User user) {
User managedUser = entityManager.merge(user);
// Additional business logic and data operations
return managedUser;
}
}
In this example, we are demonstrating the usage of the @Transactional
annotation provided by the Spring Framework to manage transactions, while also utilizing the @PersistenceContext
annotation to inject the entity manager provided by Java EE. This seamless integration ensures that data operations performed within the updateUser
method are managed within a transactional context, guaranteeing the consistency and reliability of the application's data.
A Final Look
Integrating Java EE and Spring Framework can provide a synergistic approach to enterprise application development, leveraging the strengths of both technologies to maximize efficiency and productivity. By seamlessly integrating Java EE's powerful APIs with Spring's comprehensive programming and configuration model, developers can build robust, scalable, and maintainable enterprise applications.
Additionally, the advent of microservices and cloud-native architectures has further underscored the importance of efficient integration between Java EE and Spring Framework, allowing enterprises to build modern, cloud-native applications while maintaining compatibility with established Java EE standards.
In conclusion, the seamless integration of Java EE and Spring Framework presents a compelling proposition for developers and enterprises looking to maximize efficiency and streamline their enterprise application development processes.
References:
- Java EE: https://javaee.github.io/javaee-spec/
- Spring Framework: https://spring.io/projects/spring-framework
- CDI (Contexts and Dependency Injection): https://javaee.github.io/cdi/
- Spring Data JPA: https://spring.io/projects/spring-data-jpa
- JTA (Java Transaction API): https://javaee.github.io/javaee-spec/javadocs/javax/transaction/Transactional.html