Mastering Mockito: Common Pitfalls and How to Avoid Them
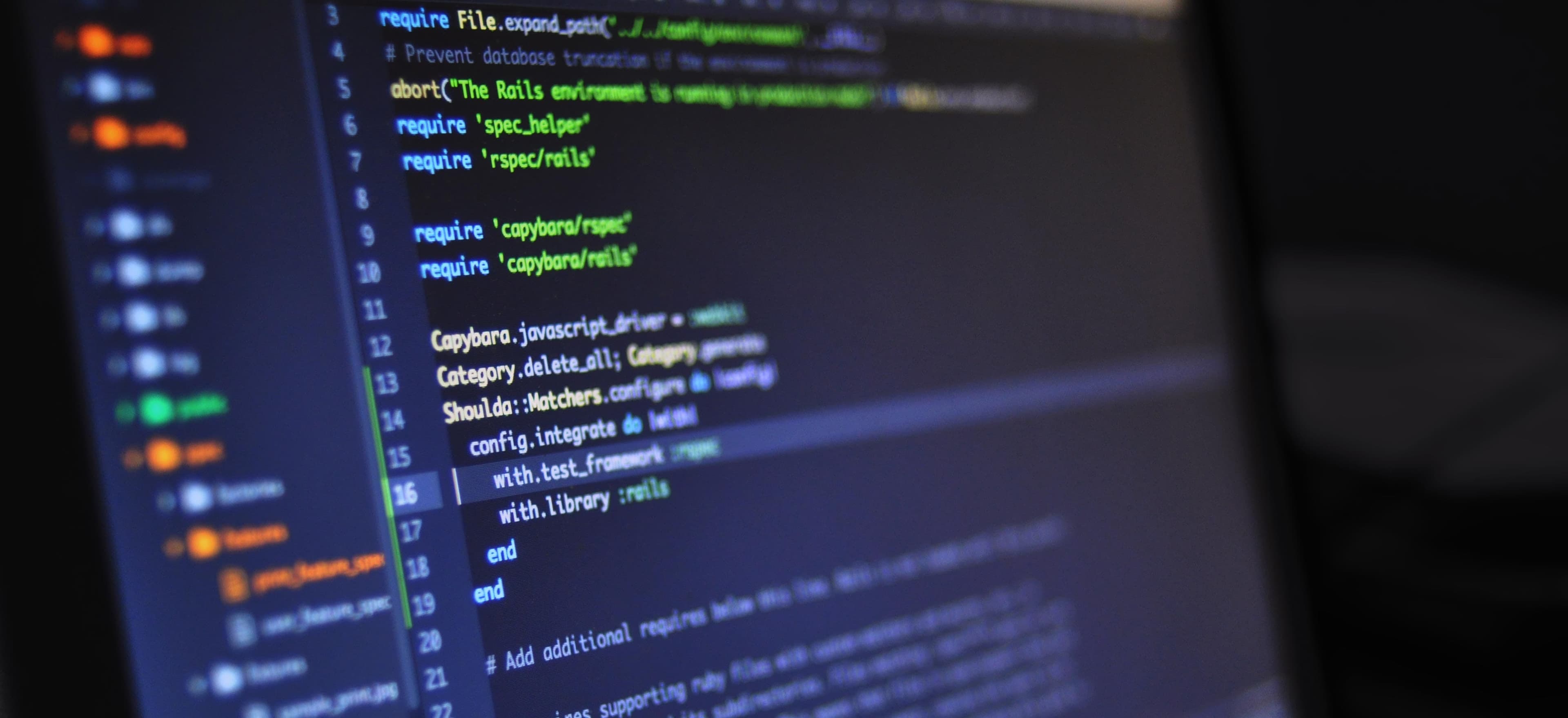
- Published on
Mastering Mockito: Common Pitfalls and How to Avoid Them
As developers, we often rely on testing frameworks to ensure our code behaves as expected. Mockito is one of the most popular frameworks for unit testing in Java. Its ability to create mock objects makes it easier to test components in isolation. However, using Mockito correctly requires a solid understanding of its nuances. In this blog post, we will discuss common pitfalls associated with Mockito and offer best practices to help you avoid them.
What is Mockito?
Mockito is a mocking framework for Java that lets you create and configure mock objects. It helps you write clean and reliable tests by allowing you to simulate complex behaviors without requiring the actual implementation of some classes. This means you can focus on testing a specific unit of code instead of dealing with dependencies.
Why Use Mockito?
- Isolation of Tests: Mockito allows you to isolate the unit of work, ensuring your tests are not affected by other parts of your application.
- Readable Tests: Tests written with Mockito can be easier to read and understand.
- Flexible Mocking: You can easily configure the behavior of mock objects to suit your test conditions.
Common Pitfalls of Using Mockito
While Mockito is powerful, there are several common pitfalls that developers often encounter. Let’s explore these pitfalls and provide solutions to avoid them.
1. Not Using Annotations Correctly
Pitfall
One common mistake is not using the right annotations or misusing them, especially when initializing mocks.
Solution
Use @Mock
, @InjectMocks
, and @Before
annotations correctly. Here’s how you can set it up:
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.mockito.junit.MockitoJUnitRunner;
@RunWith(MockitoJUnitRunner.class)
public class ExampleTest {
@Mock
private DependencyClass dependency;
@InjectMocks
private ClassUnderTest classUnderTest;
@Before
public void setUp() {
MockitoAnnotations.openMocks(this);
}
@Test
public void testMethod() {
// Your test code
}
}
Why: Using annotations simplifies your test setup and makes your tests easier to manage.
2. Ignoring Argument Matchers
Pitfall
Many developers forget that argument matchers such as any()
, eq()
, and isNull()
need to be used consistently with the when
and verify
methods.
Solution
Always use argument matchers in a consistent manner. For instance:
when(dependency.method(anyString())).thenReturn(someValue);
// Later in the test
verify(dependency).method("specificValue");
Why: Mixing matchers with explicit values can lead to unexpected results and confusing failures in your tests.
3. Stubbing Void Methods Incorrectly
Pitfall
Stubbing void methods can be tricky. Forgetting to use doNothing()
or using when()
on a void method results in an InvalidUseOfMatchersException
.
Solution
Use doNothing()
combined with when()
for stubbing void methods:
doNothing().when(dependency).voidMethod();
4. Verifying Behavior Incorrectly
Pitfall
Failing to specify the right number of invocations during verification can lead to misleading test results.
Solution
Explicitly state the expected invocations:
verify(dependency, times(1)).methodCalled();
Why: This ensures your tests are accurate and that the component under test behaves as expected during the test.
5. Using Mockito in Non-Test Classes
Pitfall
Some developers mistakenly use Mockito to mock classes in non-test classes, leading to unintended consequences and poor design.
Solution
Mockito should only be used within your test classes. Avoid any approach that uses Mockito objects in the production code.
6. Not Cleaning Up After Tests
Pitfall
Neglecting to reset mocks can result in side effects between tests, leading to false positives or negatives.
Solution
Use @After
annotations and Mockito.reset(mock)
to clean up:
@After
public void tearDown() {
Mockito.reset(mock);
}
Why: This ensures that each test runs in isolation without any lingering state from previous tests.
7. Over-Mocking
Pitfall
Over-mocking occurs when developers create overly complex mock setups that can confuse test results.
Solution
Keep mocks simple. Focus on what needs to be tested. Avoid mocking everything in sight. Ideally, mock only what is necessary to isolate the unit of work.
Key Takeaways
Mockito is an essential tool for Java developers for writing effective tests. However, pitfalls can lead to confusion and misdirection. By being aware of these common issues and implementing best practices, your experience with Mockito can be both productive and enjoyable.
Further Reading
To enhance your understanding of Mockito, consider reading the following resources:
- Mockito Official Documentation
- JUnit 5 User Guide
- Effective Unit Testing: A guide for Java Developers
With practice and knowledge, you will master Mockito and significantly improve your unit testing skills in Java. Happy testing!
This blog post encapsulates the key aspects of using Mockito effectively while keeping it informative and engaging. Start applying these tips and watch your confidence in writing unit tests grow!
Checkout our other articles