Maintaining Workflow Continuity with Effective Error Handling
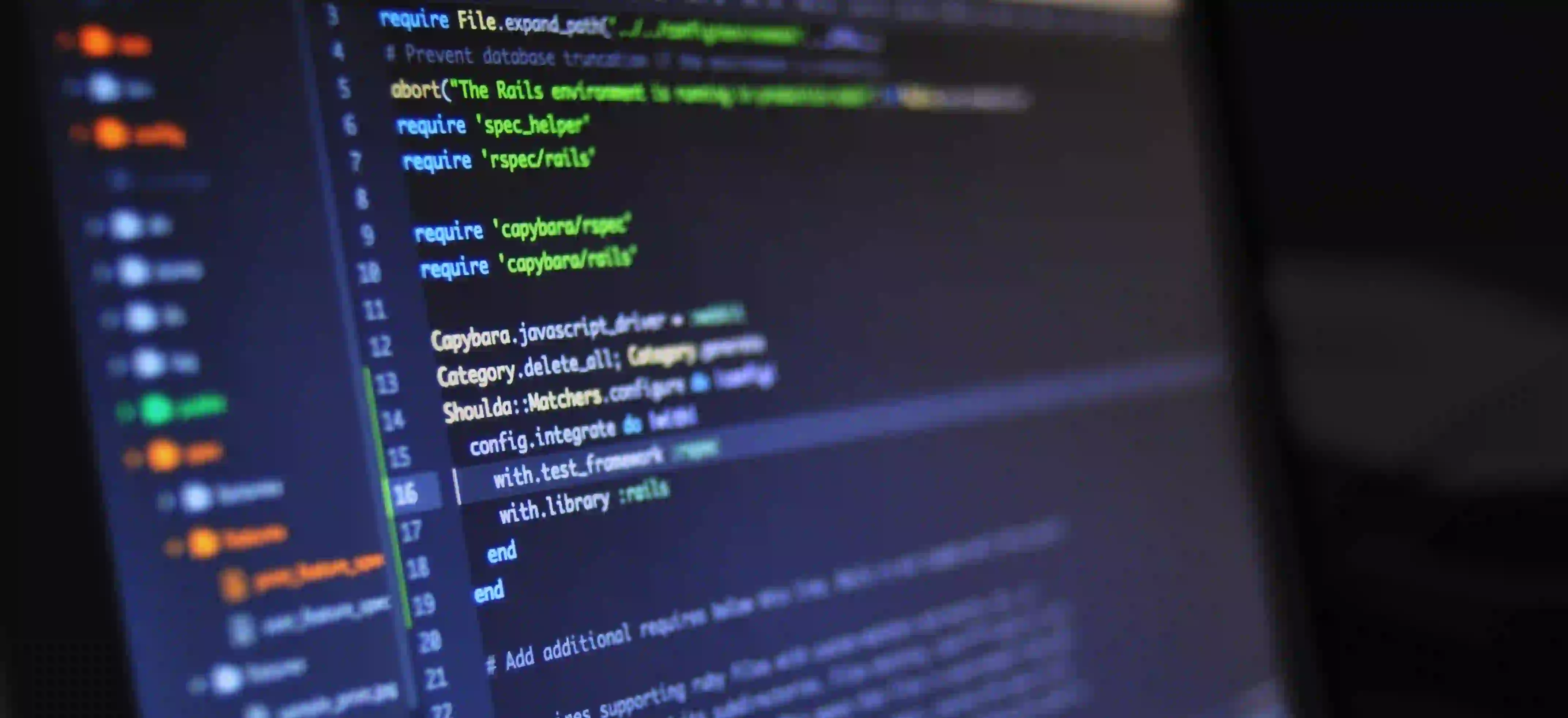
Maintaining Workflow Continuity with Effective Error Handling
Error handling is an indispensable aspect of Java programming. It ensures that applications continue to function despite encountering unexpected issues or invalid input. In this post, we'll delve into the significance of effective error handling in Java, exploring best practices, common pitfalls, and strategies for maintaining workflow continuity.
Understanding the Importance of Error Handling
In the realm of software development, errors are inevitable. They can stem from various sources, including user input, network issues, or software bugs. Failing to address these errors robustly can lead to application instability, data loss, and ultimately, a poor user experience.
Best Practices for Error Handling in Java
1. Use of Exceptions
Java employs the concept of exceptions to handle errors. When an error occurs, an exception object is thrown, which can be caught and handled appropriately. This mechanism allows for a clear separation between normal program flow and error handling logic.
Code Example:
try {
// Code that may throw an exception
} catch (SpecificException ex) {
// Handle the specific type of exception
} catch (AnotherException ex) {
// Handle another type of exception
} catch (Exception ex) {
// Catch all other exceptions
// Log the exception for debugging purposes
}
In the above code snippet, the program attempts to execute the code within the try
block. If an exception is thrown, it is caught by the corresponding catch
block, thereby enabling targeted error handling.
2. Avoid Overuse of Generic Exception Handling
While catching a generic Exception
provides a safety net for unexpected errors, it should be used sparingly. Overusing generic exception handling can mask specific issues and impede debugging efforts. It is preferable to catch specific exceptions whenever possible, thus promoting precise error resolution.
3. Resource Management with Try-With-Resources
Java 7 introduced the try-with-resources statement, which simplifies the management of resources such as file streams or database connections. With this construct, resources are automatically closed at the end of the block, mitigating the risk of resource leaks and bolstering robust error handling.
Code Example:
try (FileInputStream fileStream = new FileInputStream("file.txt")) {
// Code that utilizes the fileStream
} catch (IOException e) {
// Handle the exception
}
In the above example, the fileStream
is automatically closed at the end of the try block, regardless of whether an exception is thrown.
Common Error Handling Pitfalls in Java
1. Swallowing Exceptions
One prevalent mistake in error handling is swallowing exceptions, wherein an exception is caught but not appropriately handled or logged. This practice can obscure underlying issues, rendering them difficult to diagnose and rectify.
2. Neglecting Logging and Monitoring
Effective error handling encompasses more than just catching and handling exceptions; it also entails robust logging and monitoring. Logging the details of caught exceptions can facilitate postmortem analysis and aid in identifying recurring issues. Furthermore, integrating monitoring systems enables real-time detection of errors, empowering swift remediation.
Strategies for Maintaining Workflow Continuity
In a production environment, workflow continuity is paramount, necessitating a proactive approach towards error handling.
1. Implementing Circuit Breaker Pattern
The Circuit Breaker pattern is a fault-tolerance mechanism that enhances the resilience of distributed systems. By monitoring for failures, the circuit breaker can "trip," thereby preventing cascading failures and allowing the system to gracefully degrade when under duress.
2. Retrying Operations
For transient errors, such as network timeouts or database unavailability, implementing automatic retry mechanisms can bolster workflow continuity. By reattempting failed operations with backoff strategies, the system can often mitigate transient errors without human intervention.
My Closing Thoughts on the Matter
In the realm of Java programming, effective error handling is vital for maintaining workflow continuity and ensuring a seamless user experience. By adhering to best practices, avoiding common pitfalls, and embracing resilience strategies, developers can fortify their applications against unforeseen errors, thereby fostering robustness and reliability.
For further insights into Java error handling, consider exploring Oracle's official documentation and Baeldung's comprehensive guide.