Troubleshooting 404 Error in Spring 4: Handling Stack Overflow Voted Questions
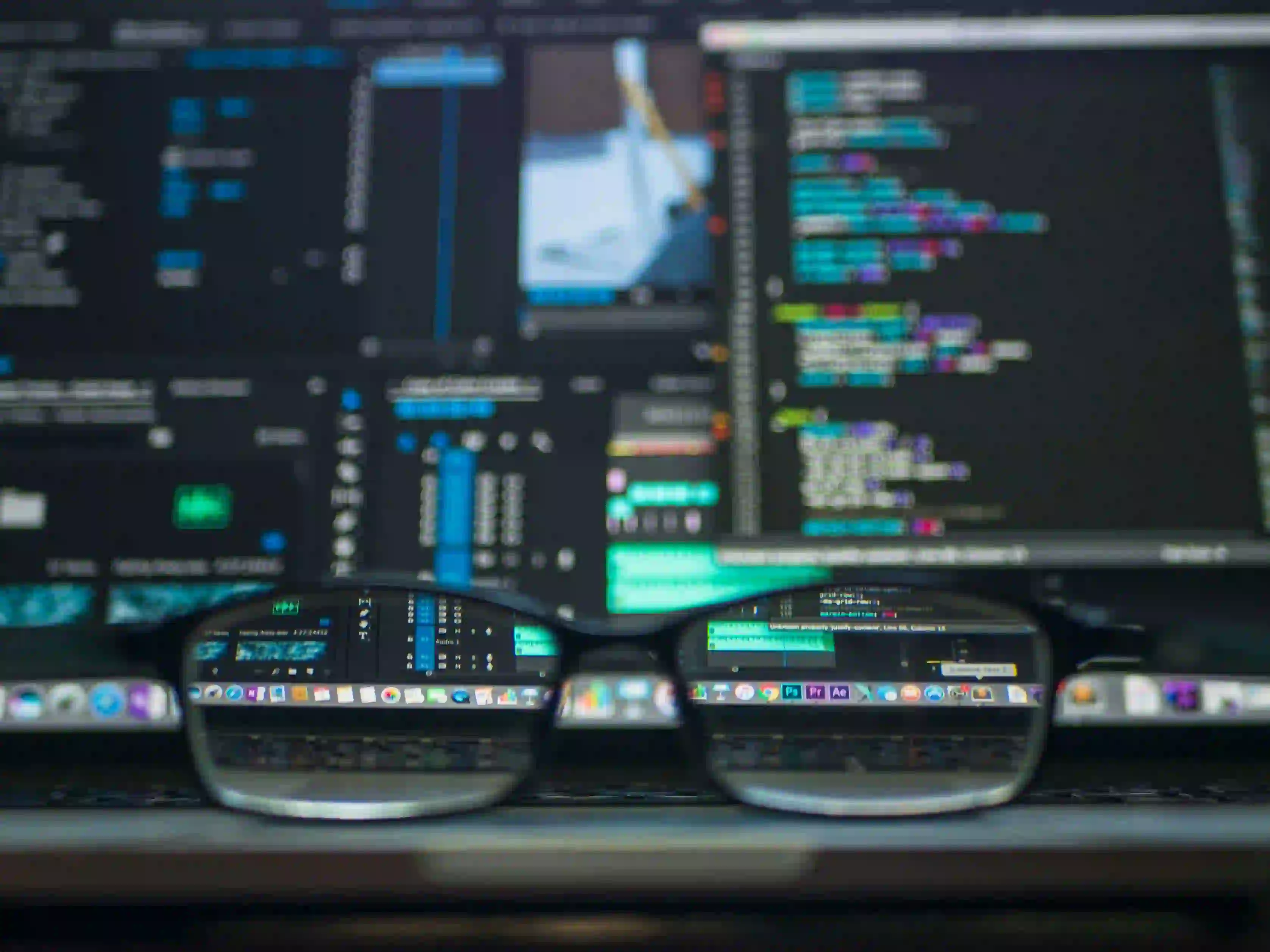
Troubleshooting 404 Error in Spring 4: Handling Stack Overflow Voted Questions
If you're encountering a 404 error in your Spring 4 application, fear not. You're not alone. Many developers, including experienced ones, have faced this issue. In this post, we'll delve into the common causes of this error and how to address them. We'll also look into some highly upvoted Stack Overflow questions related to this topic, as understanding real-world scenarios can often provide valuable insights.
Understanding the 404 Error
The 404 error, or "Not Found" error, indicates that the server could not find the requested resources. In the context of a Spring application, this typically means that the requested endpoint mapping is not found or that the application cannot handle the incoming request properly.
Common Causes
Let's explore some common reasons behind the 404 error in a Spring 4 application:
-
Incorrect Request Mapping: Check if the URL mapping in the controller aligns with the request being made. Any mismatch here can result in a 404 error.
-
Missing Controller or Handler: Ensure that the controller or handler method exists to process the incoming request. If not, the application will not be able to find the appropriate handler for the request, resulting in a 404 error.
-
Missing Configuration: Verify if the necessary configurations, such as
@EnableWebMvc
or correct component scanning, are in place. Without them, the application might struggle to locate the appropriate components to handle the request. -
Issues with @RequestMapping and @RestController: If not used correctly, these annotations can lead to 404 errors. Ensure that they are applied appropriately to avoid this issue.
Troubleshooting with Highly Upvoted Stack Overflow Questions
Now, let's take a look at some highly upvoted Stack Overflow questions that shed light on resolving the 404 error in Spring 4 applications:
1. Spring MVC @PathVariable not working
In this popular question, developers discuss various scenarios where @PathVariable
mappings may fail to work as expected. The insightful answers and discussions provide valuable tips for resolving mapping issues that could lead to 404 errors.
2. Spring MVC @RestController doesn't work and returns 404 error
Here, developers share their experiences with @RestController
and delve into how misusing this annotation can result in 404 errors. Understanding their experiences can help you prevent similar issues in your application.
3. Spring Boot app doesn't map using @RequestMapping
In this thread, developers explore various reasons why @RequestMapping
may fail to map requests properly, leading to 404 errors. The discussions provide insights into troubleshooting mappings effectively.
Considering these highly upvoted questions, it's evident that mapping issues, especially related to annotations such as @PathVariable
, @RestController
, and @RequestMapping
, are key culprits behind the 404 errors in Spring 4 applications.
Resolving the Issue
Now that we've identified potential causes and explored relevant Stack Overflow questions, let's delve into fixing the 404 error in Spring 4.
1. Verifying Request Mapping
Check the request mappings in your controller classes. Ensure that the request mappings are correctly defined and that there are no conflicts or discrepancies in the URL patterns.
@Controller
@RequestMapping("/products")
public class ProductController {
@RequestMapping(value = "/{id}", method = RequestMethod.GET)
public ResponseEntity<Product> getProductById(@PathVariable("id") Long id) {
// Logic to fetch product by ID
}
}
The @RequestMapping
annotation defines the URL pattern for handling requests. Ensure that it aligns with the incoming request URLs. Misalignment here could lead to a 404 error.
2. Reviewing Component Scan Configuration
Confirm that the component scan is set up correctly to scan the packages where your controllers and other components reside.
@Configuration
@EnableWebMvc
@ComponentScan(basePackages = "com.example")
public class AppConfig {
// Configuration settings
}
Without proper component scanning, Spring might not be able to locate the controllers and handlers, resulting in 404 errors.
3. Understanding @RestController Usage
If you're using @RestController
, ensure that it's used appropriately to handle RESTful requests.
@RestController
@RequestMapping("/api/products")
public class ProductRestController {
@GetMapping("/{id}")
public ResponseEntity<Product> getProductById(@PathVariable("id") Long id) {
// Logic to fetch product by ID
}
}
The combination of @RestController
and @RequestMapping
for each handler method must align with the desired endpoint mappings. Any mismatch might lead to 404 errors.
4. Checking Dispatcher Servlet Configuration
Verify the configuration of the Dispatcher Servlet in your web.xml
or through Servlet 3.0+ configuration. The Dispatcher Servlet plays a crucial role in handling incoming requests.
<servlet>
<servlet-name>dispatcher</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/dispatcher-servlet.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup
</servlet>
<servlet-mapping>
<servlet-name>dispatcher</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
Ensure that the DispatcherServlet
is set up correctly to handle incoming requests and route them to the appropriate controllers based on the defined mappings.
5. Checking Server Context Path
In some cases, especially when deploying to a specific context path within a server, the context path might need to be considered in the request mappings.
@RestController
@RequestMapping("${app.contextPath}/api/products")
public class ProductRestController {
// Handler methods
}
By referencing the context path dynamically, you can ensure that the application adapts to different deployment environments without hardcoding the context path.
Closing Remarks
Encountering a 404 error in a Spring 4 application can be frustrating, but understanding the underlying causes and the proven solutions is the key to resolving it effectively. By thoroughly reviewing the request mappings, configuration settings, and proper usage of annotations, you can mitigate the occurrences of 404 errors.
Remember, while troubleshooting, referring to highly upvoted Stack Overflow questions can provide valuable insights from the experiences of other developers facing similar issues. Using the collective knowledge of the community can significantly expedite the resolution process, saving you time and effort.
In the realm of Spring 4 development, encountering challenges like the 404 error is not uncommon. Embrace the learning opportunities they present, and continue honing your skills to become a more proficient developer.