Maximizing Performance with Asynchronous Grails Controllers
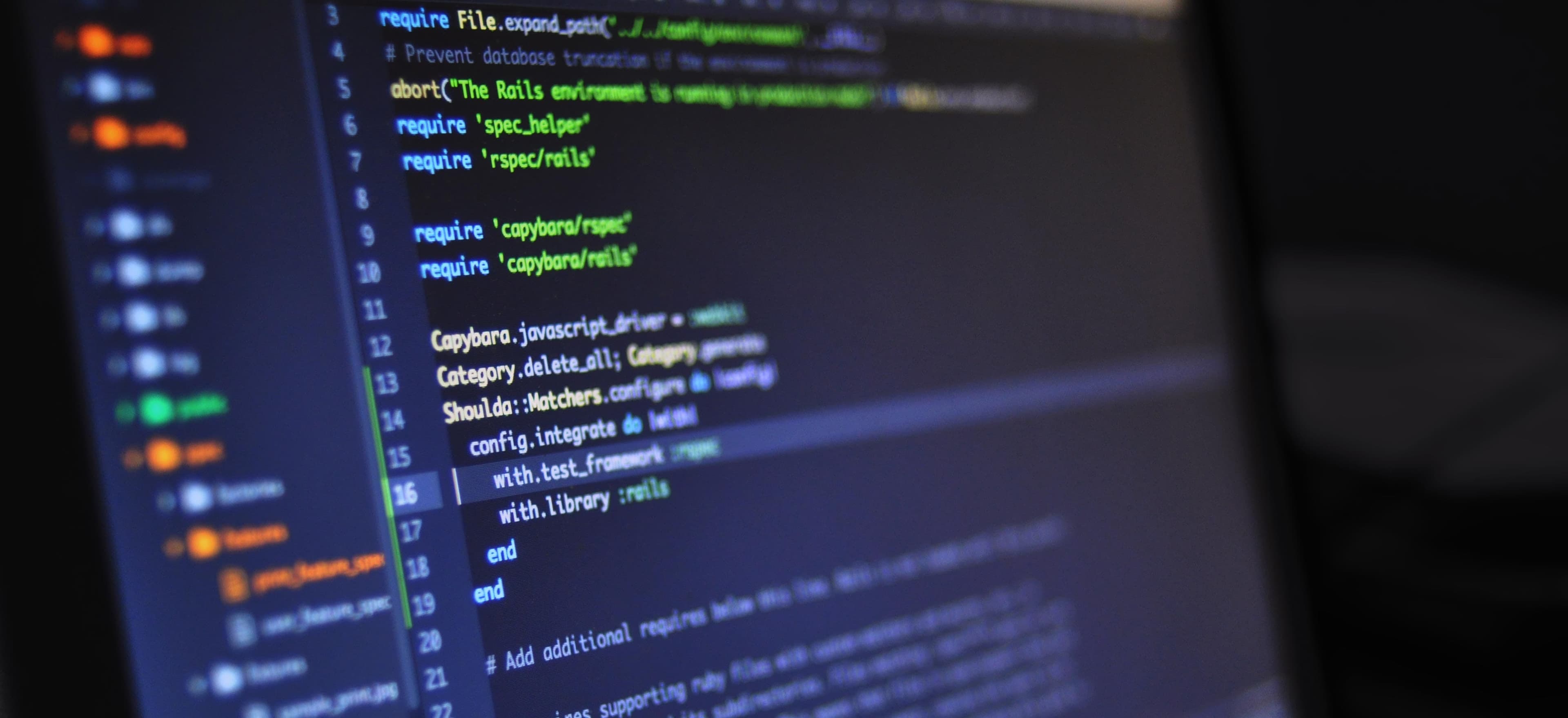
- Published on
Maximizing Performance with Asynchronous Grails Controllers
In today's fast-paced world, website performance plays a crucial role in user experience and search engine rankings. As a Java developer using the Grails framework, optimizing the performance of your web application is essential to ensure a smooth and responsive user experience. One powerful way to maximize performance in Grails is by utilizing asynchronous controllers.
Understanding Asynchronous Programming
Asynchronous programming allows you to perform tasks concurrently, enabling your application to handle more requests without blocking the thread. In traditional synchronous programming, each request is processed sequentially, potentially leading to thread blocking and reduced performance under heavy load.
By contrast, asynchronous programming enables non-blocking behavior, allowing the application to continue processing other tasks while waiting for slow I/O operations, such as accessing a database or making an API call. This concurrency significantly improves the responsiveness and overall performance of your application.
Implementing Asynchronous Controllers in Grails
Grails provides seamless support for asynchronous programming through its controllers. By leveraging the event-driven and non-blocking nature of Grails, you can create high-performance web applications. Let's dive into how you can implement asynchronous controllers in Grails.
Annotating the Controller Actions
To make a controller action asynchronous in Grails, you can annotate the action with the @Async
annotation. This simple annotation tells Grails to execute the action asynchronously, utilizing the underlying capabilities of the framework to handle concurrency.
import grails.async.NonBlocking
import grails.async.Async
class MyController {
@Async
def asyncAction() {
// Asynchronous logic here
}
}
Leveraging Promises
In Grails, asynchronous programming often involves working with Promises. A Promise represents a future value and allows you to handle the result once it becomes available. By leveraging Promises, you can create non-blocking and responsive controller actions.
import grails.async.Promise
class MyController {
def asyncAction() {
def promise = task {
// Asynchronous logic here
}
promise.onComplete { result ->
// Handle the result
}
}
}
Using Asynchronous I/O Operations
Another key aspect of optimizing performance with asynchronous controllers in Grails is utilizing asynchronous I/O operations. When interacting with external resources like databases or APIs, using asynchronous I/O operations prevents the thread from being blocked, enabling the application to handle more concurrent requests efficiently.
import grails.async.Promise
class MyController {
def asyncDatabaseOperation() {
def promise = task {
// Asynchronous database operation
// Example: accessing a database using GORM
def result = Book.where { title == "Grails in Action" }.list()
result
}
promise.onComplete { result ->
// Handle the database operation result
}
}
}
Benefits of Asynchronous Controllers
Implementing asynchronous controllers in your Grails application offers several significant benefits, all of which contribute to maximizing performance.
Improved Scalability
Asynchronous programming allows your application to handle a larger number of concurrent requests without consuming additional resources. This enhanced scalability is crucial for web applications experiencing high traffic or frequent I/O operations.
Enhanced Responsiveness
By avoiding thread blocking, asynchronous controllers ensure that your application remains responsive, providing a seamless user experience even when dealing with time-consuming tasks.
Resource Efficiency
Asynchronous programming maximizes the efficient utilization of system resources, as threads are not needlessly blocked while waiting for I/O operations to complete. This efficient resource management leads to better overall performance.
Better Throughput
The non-blocking nature of asynchronous controllers results in better throughput, as the application can process multiple requests simultaneously, leading to improved performance metrics.
Best Practices for Asynchronous Controllers
While asynchronous programming can greatly enhance the performance of your Grails application, it's essential to follow best practices to ensure reliable and maintainable code.
Monitoring and Testing
Monitor the performance of your asynchronous controllers to identify any potential bottlenecks and ensure that they are performing optimally. Additionally, create comprehensive tests to validate the behavior and performance of asynchronous actions under various scenarios.
Proper Error Handling
Asynchronous programming introduces new considerations for error handling. It's crucial to implement robust error handling mechanisms to manage exceptions and failures in asynchronous controller actions effectively.
Thorough Documentation
Document the asynchronous controller actions thoroughly, including details about the asynchronous behavior and any specific considerations for developers who may work with the code in the future.
In Conclusion, Here is What Matters
Optimizing the performance of your Grails application by leveraging asynchronous controllers is a powerful approach to creating high-performance, scalable, and responsive web applications. By embracing asynchronous programming, you can unlock the full potential of Grails and deliver a superior user experience while efficiently managing system resources.
Asynchronous controllers are a valuable tool in the arsenal of any Grails developer striving to maximize application performance. With careful design and implementation, asynchronous programming can elevate your Grails application to new heights of speed and responsiveness, ensuring that it meets the demands of modern web development.
By mastering the art of asynchronous programming in Grails, you can position your application for success in an increasingly competitive digital landscape.
Ready to unlock the full potential of asynchronous programming in Grails? Dive deeper into the asynchronous capabilities of Grails and propel your web applications to new levels of performance!
Checkout our other articles