Optimizing Test Coverage in Library Packages
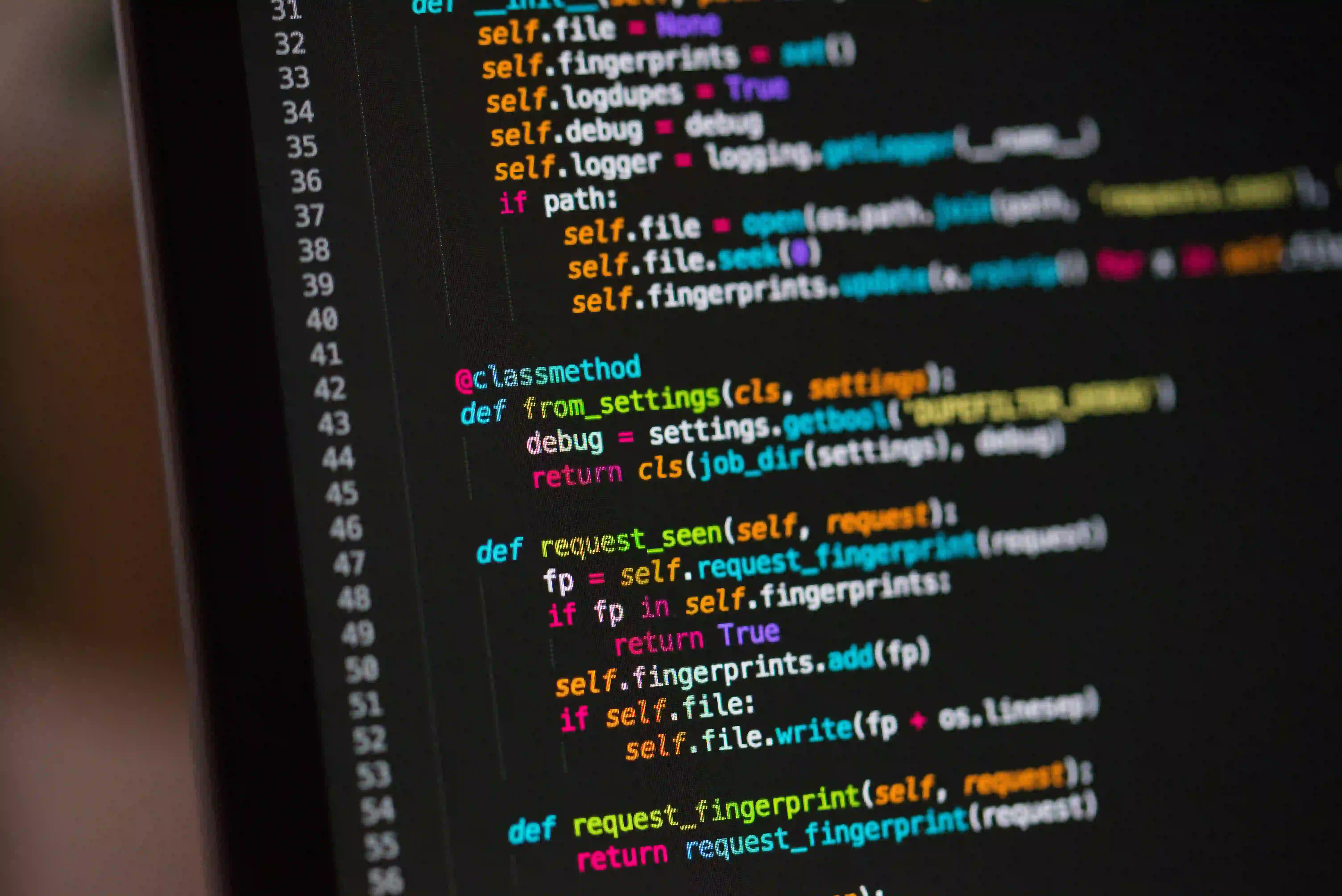
Maximizing Test Coverage in Java Library Packages
When developing a Java library, ensuring comprehensive test coverage is essential for maintaining code quality and robustness. In this blog post, we will explore strategies to maximize test coverage in Java library packages to guarantee the reliability and stability of the codebase.
Why Test Coverage Matters
Test coverage measures the percentage of code that is executed by tests. A high test coverage indicates that the majority of the code has been exercised by tests, resulting in greater confidence in the reliability of the software. For library packages, comprehensive tests are crucial as they serve as a contract for consumers, ensuring that the API functions as expected and that changes can be made with confidence in the resulting behavior.
Choosing the Right Testing Framework
Selecting an appropriate testing framework is the first step in maximizing test coverage. JUnit, the de-facto standard for unit testing in Java, provides a solid foundation for creating and executing tests. Utilizing JUnit's annotations such as @Test
, @Before
, and @After
can streamline the creation of test suites and fixtures. Additionally, libraries like Mockito can be employed for creating mock objects and simulating behaviors to facilitate thorough testing.
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 4);
assertEquals(7, result);
}
In-depth Unit Testing
Unit tests are the cornerstone of achieving high test coverage. By isolating individual components and testing them in isolation, potential bugs and regressions can be identified early in the development process. It's crucial to test not only the expected behavior but also edge cases and failure scenarios to ensure comprehensive coverage.
Employing Code Coverage Tools
Tools such as JaCoCo and Cobertura provide insights into the effectiveness of test suites by measuring code coverage. These tools identify which areas of the codebase are lacking in test coverage, enabling developers to target those areas and create additional tests to increase coverage. Integrating these tools into the build process allows for continuous monitoring of code coverage and empowers teams to set and achieve coverage targets.
Applying Test-Driven Development (TDD)
Test-driven development (TDD) promotes writing tests before implementing the corresponding functionality. By following a "test-first" approach, developers can ensure that every line of code is accompanied by a test. This practice not only leads to higher test coverage but also encourages thoughtful design and modular architecture, resulting in more testable and maintainable code.
Encouraging Collaboration and Code Reviews
Collaboration among team members fosters a culture of quality and accountability. Encouraging peers to review and contribute to test suites can unearth blind spots and edge cases that may have been overlooked. Through constructive feedback, the overall test coverage can be significantly improved, ultimately leading to a more reliable and robust library.
Integration and End-to-End Testing
In addition to unit testing, integration and end-to-end testing are vital for validating the behavior of the library as a whole. Integration tests verify the interaction between different components, while end-to-end tests ensure that the library functions as expected in real-world scenarios. Tools like Selenium and TestNG are valuable for creating automated end-to-end tests that mimic user interactions and verify the library's behavior in a production-like environment.
Continuous Integration and Deployment (CI/CD)
Integrating test suites into a continuous integration and deployment pipeline ensures that all changes to the library are subject to thorough testing. By automating the execution of tests upon every commit or pull request, potential issues can be identified early in the development process, preventing defects from propagating into subsequent stages.
In Conclusion, Here is What Matters
In conclusion, maximizing test coverage in Java library packages is imperative for delivering reliable, high-quality software. Through the utilization of appropriate testing frameworks, code coverage tools, test-driven development practices, collaborative code reviews, and comprehensive testing strategies, developers can ensure that their library is thoroughly validated and primed for production use. Embracing a culture of testing and quality assurance not only instills confidence in the codebase but also fosters a resilient and trustworthy library for its consumers.
To delve deeper into Java testing and coverage optimization, you can refer to the official JUnit documentation and explore advanced techniques for code coverage using tools like JaCoCo and Cobertura.
Remember, comprehensive tests not only validate the code but also embody the trust and confidence you wish to instill in your library users. Happy coding!