Mastering Java Interview Questions: Your Key to Success
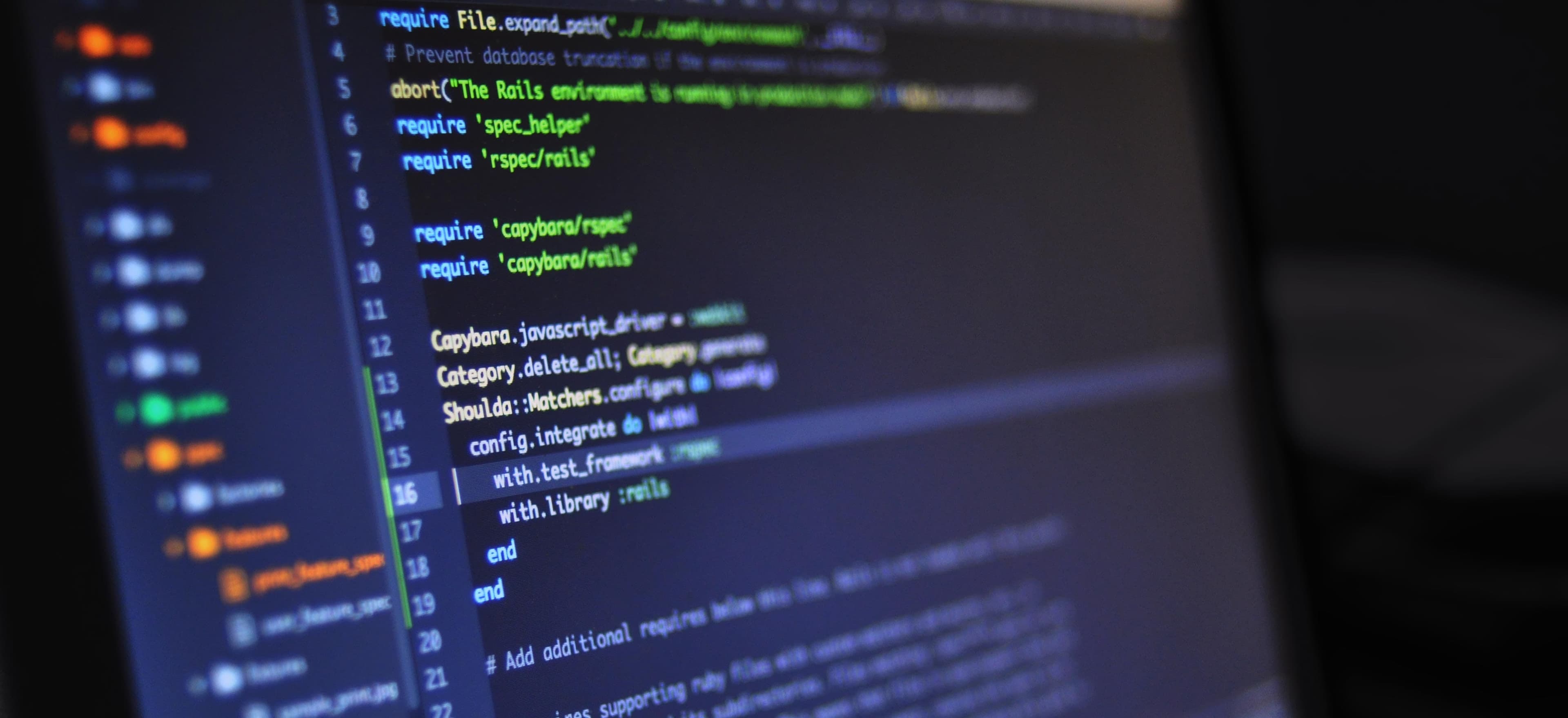
- Published on
Mastering Java Interview Questions: Your Key to Success
Are you getting ready for a Java developer interview and feeling the pre-interview jitters? Fret not, as we've got you covered! In this comprehensive guide, we'll walk you through some of the most common and challenging Java interview questions, providing insightful explanations and exemplary code snippets. Let's dive in!
1. What is Java?
Java is a widely-used, class-based, object-oriented programming language designed to have as few implementation dependencies as possible. It is intended to let application developers write once, run anywhere (WORA), meaning that compiled Java code can run on all platforms that support Java without the need for recompilation.
2. Explain the concept of Object-Oriented Programming (OOP).
Object-Oriented Programming is a programming paradigm based on the concept of "objects." These objects can contain data, in the form of fields (often known as attributes or properties), and code, in the form of procedures (often known as methods). OOP aims to implement real-world entities like inheritance, hiding, polymorphism, etc., in programming.
3. What is the difference between an interface and an abstract class in Java?
An interface in Java is a blueprint of a class that contains static constants and abstract methods, which are also known as "interfaces." On the other hand, an abstract class is a class that may contain a mix of methods that are declared with or without an implementation.
// Example of an interface
public interface Vehicle {
void start();
void stop();
}
// Example of an abstract class
public abstract class Shape {
abstract void draw();
public void color() {
System.out.println("Coloring the shape");
}
}
Why use an interface over an abstract class?
- Interfaces are useful when a class needs to implement multiple behaviors.
- Java doesn't support multiple inheritance, so using an interface helps achieve it.
4. What is the difference between the String
, StringBuffer
, and StringBuilder
classes in Java?
String
: Immutable and thread-safe.StringBuffer
: Mutable and thread-safe.StringBuilder
: Mutable but not thread-safe.
String str = "Hello"; // immutable
StringBuffer strBuffer = new StringBuffer("Hello"); // mutable and thread-safe
StringBuilder strBuilder = new StringBuilder("Hello"); // mutable but not thread-safe
Why choose StringBuilder
over StringBuffer
?
StringBuilder
should be preferred when the object is only accessed from a single thread. It offers better performance than StringBuffer
.
5. What is the difference between ArrayList
and LinkedList
?
ArrayList
: Uses a dynamic array to store the elements. Retrieval is faster compared to LinkedList.LinkedList
: Uses a doubly-linked list to store the elements. Insertion and deletion are faster compared to ArrayList.
ArrayList<String> arrayList = new ArrayList<>(); // dynamic array
LinkedList<String> linkedList = new LinkedList<>(); // doubly-linked list
When to use ArrayList
or LinkedList
?
- Use
ArrayList
when there are more retrievals, andLinkedList
for more insertions and deletions.
6. Discuss the concept of Exception Handling in Java.
Exception handling in Java is a mechanism to handle runtime errors. It is done using five keywords: try
, catch
, throw
, throws
, and finally
. Exceptions can be of the type checked (compile-time) or unchecked (runtime).
try {
// code that may throw an exception
} catch (ExceptionType1 ex) {
// handle ExceptionType1
} catch (ExceptionType2 ex) {
// handle ExceptionType2
} finally {
// always executed
}
Why is exception handling important?
Exception handling helps in maintaining the normal flow of the program even if an exception occurs, which prevents abnormal termination.
The Last Word
Mastering Java interview questions is crucial for anyone looking to ace a Java developer's interview. By understanding and practicing these concepts, you can confidently tackle various technical questions with ease. Keep learning, keep practicing, and you'll be well-prepared for your next Java interview!
Remember to check out Java Interview Questions for more comprehensive resources and practice questions.
Now, go conquer that interview! Good luck!