Optimizing Java EE Application Deployment with Docker and Maven
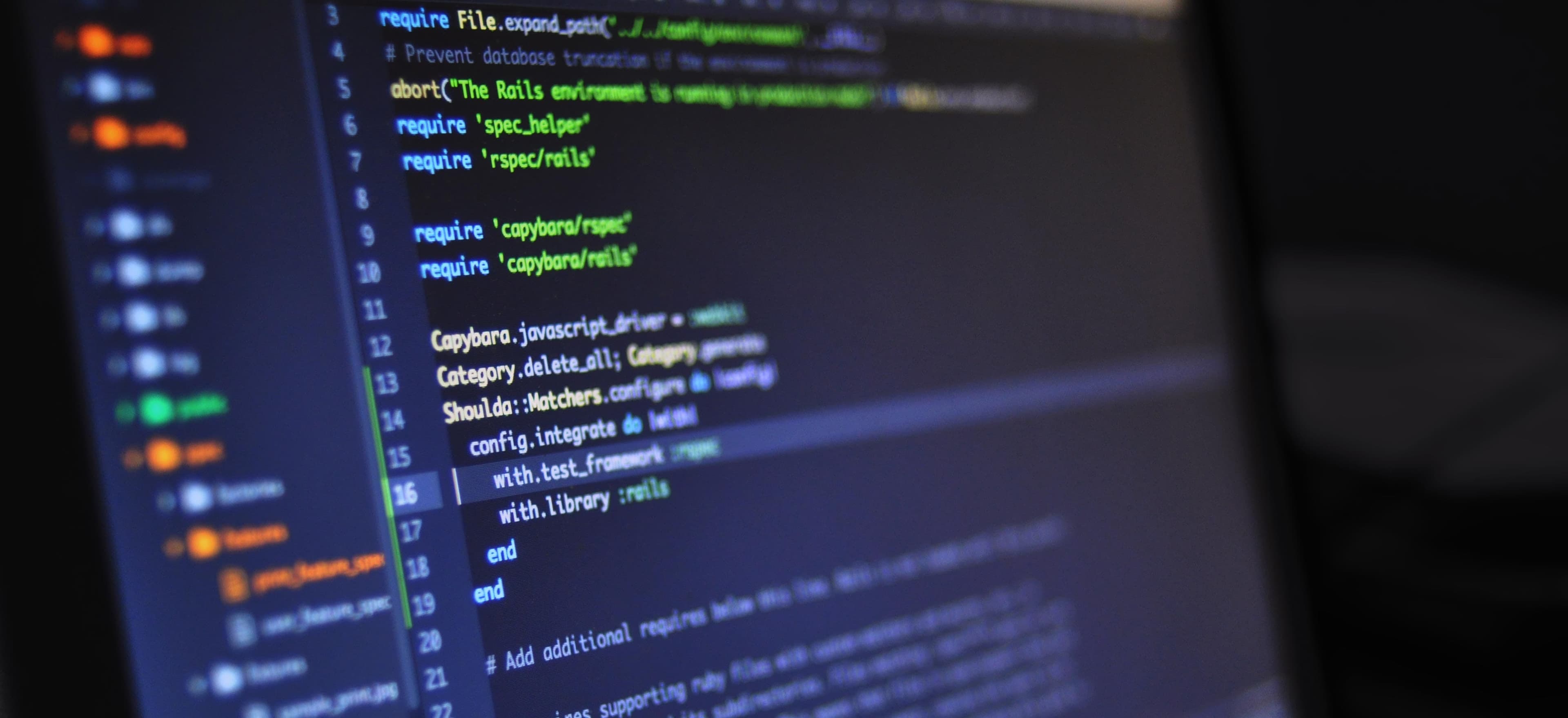
- Published on
Optimizing Java EE Application Deployment with Docker and Maven
In today's fast-paced development environment, deploying a Java EE application efficiently is crucial. With the growing popularity of Docker and Maven, optimizing the deployment process has become easier and more effective. In this article, we will explore how to leverage Docker and Maven to streamline the deployment of Java EE applications.
What is Docker?
Docker is a platform that enables developers to develop, ship, and run applications in a consistent environment called a container. Containers are lightweight, portable, and self-sufficient, providing an isolated environment for applications to run without affecting the host system.
Why Use Docker for Deploying Java EE Applications?
Using Docker for deploying Java EE applications offers several benefits, including:
-
Consistency: Docker ensures that the application runs consistently across different environments, whether it's development, testing, or production.
-
Isolation: Containers isolate the application and its dependencies, preventing conflicts with other applications or the host system.
-
Portability: Docker containers can be easily moved between different environments, making the deployment process more flexible and efficient.
Leveraging Maven for Java EE Application Build
Maven is a widely used build automation tool for Java projects. It simplifies the build process by managing project dependencies, compiling the source code, running tests, and packaging the application into a distributable format.
To begin, ensure that you have Maven installed on your system. You can do this by running the following command:
mvn -version
If you do not have Maven installed, you can follow the official installation guide provided by the Apache Maven project.
Once Maven is installed, create a pom.xml
file in the root directory of your Java EE application. This file will define the project structure, dependencies, and build configuration for your application.
Here's an example pom.xml
file:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-java-ee-app</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<!-- Add your Java EE dependencies here -->
</dependencies>
<build>
<finalName>my-java-ee-app</finalName>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<!-- Add other plugins for packaging, testing, etc. -->
</plugins>
</build>
</project>
In this example, the pom.xml
file defines the project's groupId
, artifactId
, version
, and packaging
. It also includes a placeholder for dependencies and build configurations.
Building a Docker Image for Java EE Application
To build a Docker image for your Java EE application, create a Dockerfile
in the root directory of your project. The Dockerfile
contains instructions for building the Docker image, including dependencies, environment setup, and application deployment.
Here's an example Dockerfile
for a Java EE application:
# Use a base image containing Java and Tomcat
FROM tomcat:8-jre8
# Copy the war file into the webapps directory of Tomcat
COPY target/my-java-ee-app.war /usr/local/tomcat/webapps/
In this example, the Dockerfile
uses a base image that includes Java and Tomcat, copies the Java EE application (war file) into the webapps directory of Tomcat, and prepares the environment for running the application.
To build the Docker image, navigate to the root directory of your Java EE application and run the following command:
docker build -t my-java-ee-app .
This command builds a Docker image for your Java EE application with the tag my-java-ee-app
based on the instructions in the Dockerfile
.
Running the Dockerized Java EE Application
Once the Docker image is built, you can run the Dockerized Java EE application using the following command:
docker run -p 8080:8080 my-java-ee-app
This command runs the Docker container for your Java EE application, mapping port 8080 on the host to port 8080 within the container. You can access the running application in a web browser by navigating to http://localhost:8080/my-java-ee-app
.
Bringing It All Together
In this article, we have explored how to optimize the deployment of Java EE applications using Docker and Maven. By leveraging Docker for consistent, isolated, and portable application deployment, and utilizing Maven for streamlined build processes, developers can simplify the deployment workflow and ensure the reliability and scalability of their Java EE applications.
By following these best practices and incorporating tools like Docker and Maven into your development workflow, you can streamline the deployment process and focus on delivering high-quality Java EE applications with confidence and efficiency.
For more in-depth information, you may refer to the official Docker documentation and Maven website.
Remember, optimizing your Java EE application deployment not only saves time and effort but also enhances the overall performance and reliability of your application.