Managing Security Risks in Hybrid Multicloud Environments
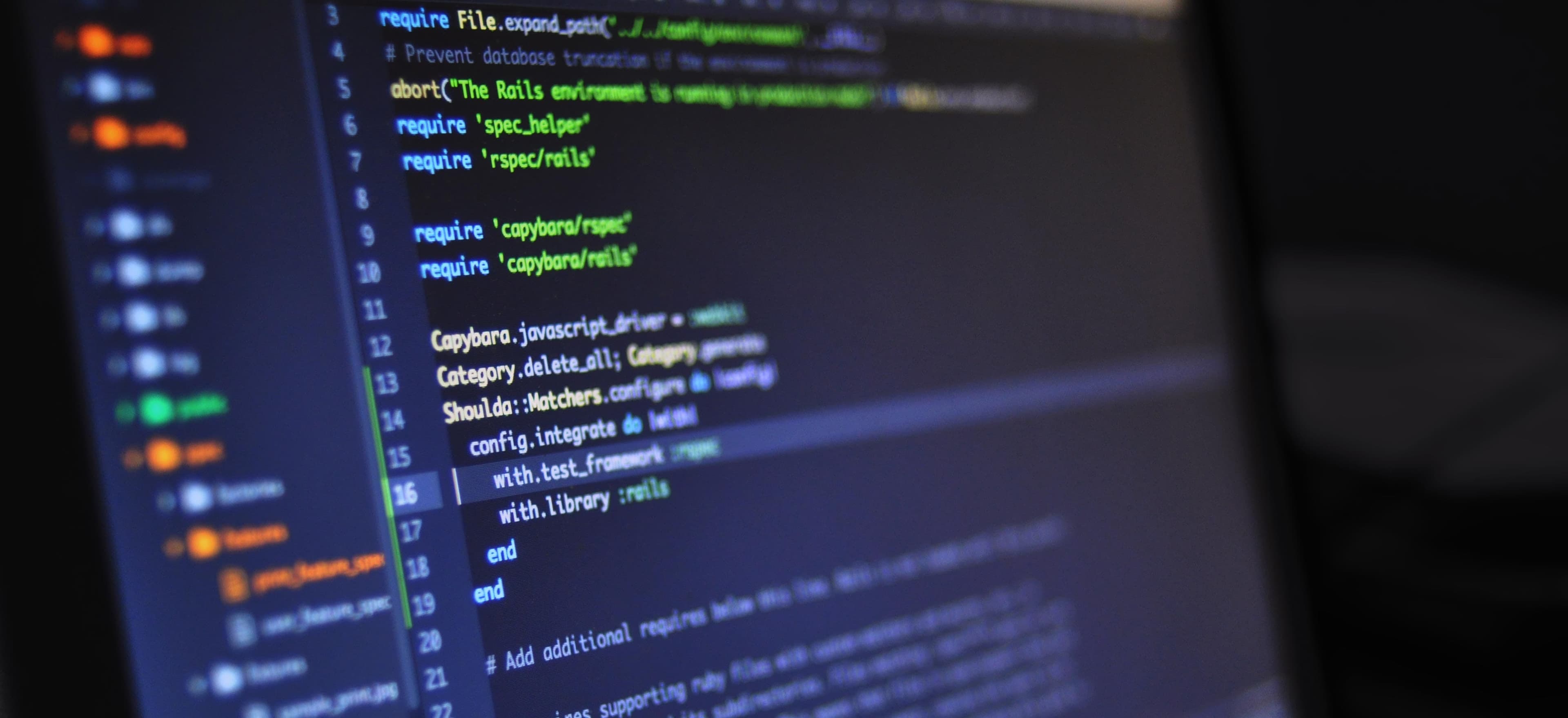
- Published on
Understanding Security Risks in Hybrid Multicloud Environments
As more organizations adopt multicloud strategies, the complexity of managing security across multiple cloud environments has become a significant concern. With the proliferation of hybrid multicloud deployments, the need for robust security measures has become more critical than ever. In this article, we will explore the unique security challenges posed by hybrid multicloud environments and discuss best practices for managing security risks effectively.
The Security Landscape of Hybrid Multicloud Environments
Hybrid multicloud environments combine private, public, and edge clouds, creating a diverse and interconnected ecosystem. This complexity introduces several security risks, including:
1. Data Protection and Privacy
Data stored and transmitted across different cloud environments increase the risk of unauthorized access and data breaches. As data traverses between on-premises and multiple cloud platforms, enforcing consistent data protection measures becomes a daunting task.
2. Identity and Access Management
Managing user identities and access controls across disparate cloud platforms and on-premises infrastructure poses a significant challenge. Inconsistent access policies and misconfigured identity management can lead to unauthorized access and insider threats.
3. Network Security
Interconnecting multiple cloud environments and on-premises infrastructure requires secure network configurations. Inadequate network security can expose vulnerabilities to malicious actors and compromise the integrity of data transmission.
4. Compliance and Governance
Ensuring compliance with industry regulations and internal governance standards across hybrid multicloud environments demands granular visibility and control. Failure to meet compliance requirements can result in severe legal and financial repercussions.
Best Practices for Managing Security Risks
1. Implement Consistent Security Policies
Establishing uniform security policies across all cloud environments and on-premises infrastructure is paramount. Utilizing tools like AWS Identity and Access Management (IAM) and Azure Active Directory enables centralized management of access controls and permissions.
2. Employ Multifactor Authentication (MFA)
Mitigate the risk of unauthorized access by implementing MFA across all access points. This additional layer of security enhances identity protection and minimizes the impact of compromised credentials.
3. Encrypt Data in Transit and at Rest
Leverage encryption mechanisms such as TLS/SSL for securing data transmission between cloud environments and on-premises infrastructure. Additionally, employing robust encryption methods for data at rest ensures its confidentiality and integrity.
4. Implement Network Segmentation
Isolating different cloud environments and segments of on-premises infrastructure through network segmentation reduces the attack surface and contains security breaches, thereby preventing lateral movement by potential attackers.
5. Continuous Monitoring and Threat Detection
Deploying robust Intrusion Detection Systems (IDS) and Security Information and Event Management (SIEM) solutions facilitates real-time monitoring, detection, and response to security threats across the hybrid multicloud environment.
6. Automation for Incident Response
Implement automated incident response mechanisms to swiftly address and remediate security incidents. Automation streamlines the incident response process, mitigating the impact of security breaches and minimizing downtime.
Harnessing the Power of Java for Security in Hybrid Multicloud Environments
Java, with its strong focus on security and platform independence, plays a pivotal role in addressing security challenges within hybrid multicloud environments. Let's delve into specific ways Java can bolster security in this complex landscape.
Secure Coding Practices
Java's robust security features, such as bytecode verification and runtime sandboxing, bolster the resilience of applications against vulnerabilities like buffer overflows and memory corruption. By adhering to secure coding practices and leveraging Java's built-in security mechanisms, developers can fortify their applications against potential exploits.
// Example of secure coding in Java
public class SecureExample {
public void processInput(String input) {
// Sanitize input to prevent SQL injection
String sanitizedInput = input.replaceAll("[^a-zA-Z0-9]", "");
// Process the sanitized input
}
}
In the above code snippet, the processInput
method demonstrates input sanitization to prevent SQL injection, showcasing a secure coding practice in Java.
Cryptography and Secure Communication
Java's extensive support for cryptographic operations and strong encryption protocols empowers developers to secure data transmission and storage within hybrid multicloud environments. Leveraging Java's Java Cryptography Architecture (JCA), developers can implement robust encryption and hashing algorithms to safeguard sensitive information.
// Example of encrypting data using Java's JCA
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class EncryptionExample {
public byte[] encryptData(byte[] inputData, SecretKey key) {
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(inputData);
}
}
The above code snippet showcases how Java's JCA can be utilized for encrypting data using the AES encryption algorithm, highlighting Java's prowess in secure communication.
Access Control and Authentication
Java's robust libraries and frameworks, such as Spring Security, facilitate the implementation of fine-grained access control and robust authentication mechanisms. Integrating Spring Security, developers can enforce stringent access policies and implement comprehensive authentication protocols across hybrid multicloud environments.
// Example of defining access control using Spring Security
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("USER", "ADMIN")
.and().formLogin();
}
}
In the provided snippet, the SecurityConfig
class demonstrates the definition of access control using Spring Security, showcasing Java's capabilities in enforcing access policies.
The Last Word
In conclusion, safeguarding hybrid multicloud environments against security risks necessitates a comprehensive approach encompassing consistent security policies, encryption, access control, and automated threat detection. Java's robust security features and versatile libraries empower developers to fortify applications and communications within these complex environments. By integrating best practices and leveraging Java's security capabilities, organizations can navigate the security challenges of hybrid multicloud deployments with confidence and resilience.
Checkout our other articles