Addressing Default Constructor Warnings in JDK 16
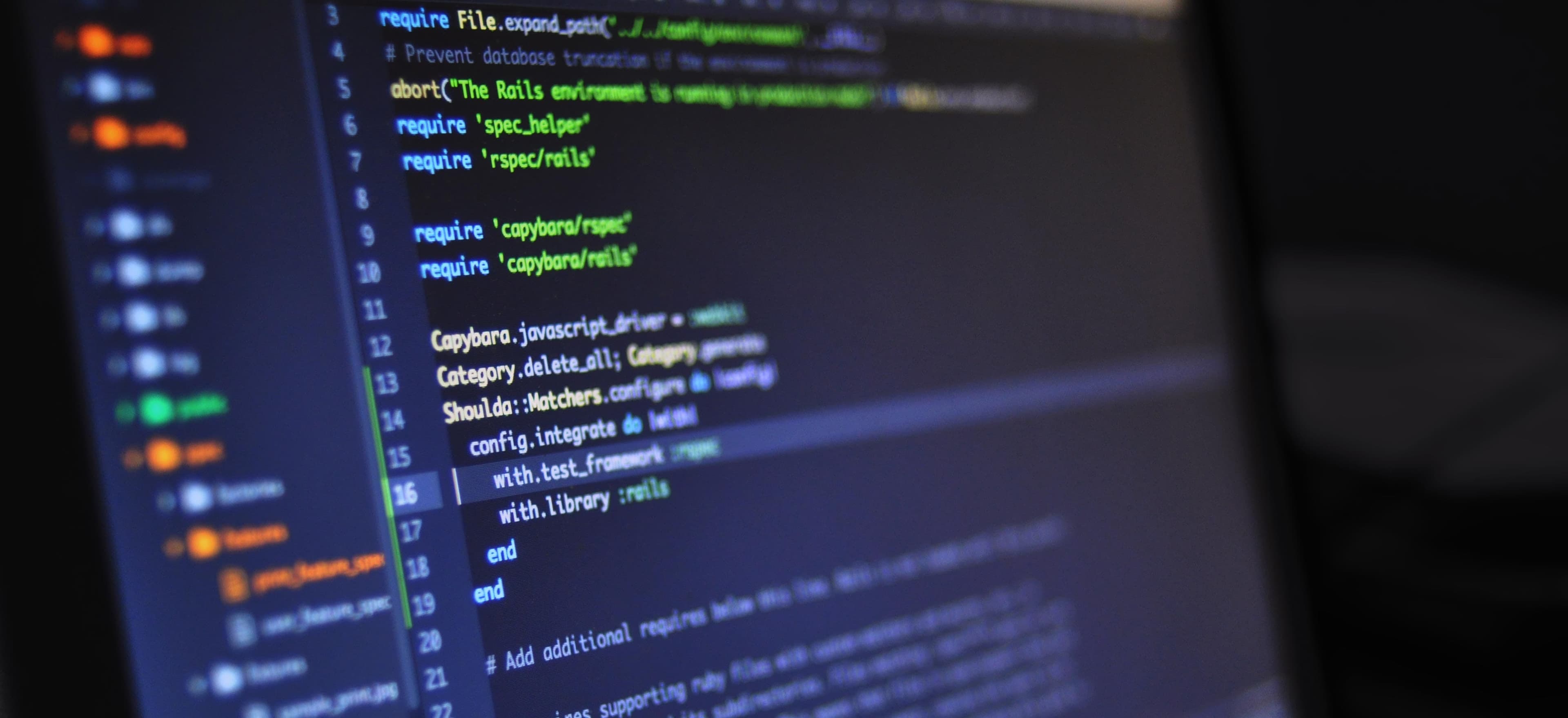
- Published on
Understanding Default Constructor Warnings in JDK 16
In JDK 16, a new warning was introduced to highlight the potential misuse of default constructors. This warning aims to improve code clarity, maintainability, and overall code quality. Let's delve into the significance of this warning, understand its implications, and explore how to address it in your Java code.
The Role of Default Constructors
Default constructors are automatically generated by the Java compiler when a class does not explicitly define any constructors. These constructors are implicitly available to initialize the object's state. While default constructors can be convenient, they can also lead to unintended consequences if not used judiciously.
The Warning
In JDK 16, the introduction of a warning for default constructors underscores the importance of explicitly defining constructors in Java classes. This warning encourages developers to be explicit about their intent and avoid relying solely on default constructors.
Why Is the Warning Important?
The warning serves as a reminder that default constructors might not convey the intended initialization logic clearly. It promotes the use of explicit constructors with clearly defined parameters, helping enhance code readability and maintainability. By addressing this warning, developers can make their code more self-explanatory and less error-prone.
How to Address the Warning
To address the default constructor warning in JDK 16, developers are encouraged to review their codebase and make intentional decisions about the usage of default constructors. Here are some steps to consider:
1. Explicitly Define Constructors
Explicitly defining constructors with appropriate parameters aligns with best practices and makes the code more explicit. By doing so, developers can convey their intentions clearly and establish a consistent approach to object initialization.
public class MyClass {
private int myField;
// Explicit constructor
public MyClass(int myField) {
this.myField = myField;
}
}
2. Review Default Constructor Usage
If the default constructor is used in the codebase, evaluate whether there is a genuine need for it. Consider whether an explicit constructor would provide better clarity and encapsulation of the object's initialization logic.
3. Utilize Dependency Injection
In scenarios where the default constructor is used for dependency injection frameworks or other similar purposes, consider using explicit dependency injection frameworks such as Spring Framework or Google Guice. These frameworks provide robust mechanisms for object creation and dependency management, minimizing the reliance on default constructors.
4. Leverage Builder Pattern
For classes requiring a large number of parameters for initialization, consider implementing the builder pattern. This allows for fluent and expressive object construction without the need for excessively long constructor parameter lists.
Embracing Best Practices
Addressing the default constructor warning in JDK 16 aligns with Java best practices and promotes cleaner, more maintainable code. By being intentional about constructors and object initialization, developers can enhance the overall robustness and clarity of their codebase.
Bringing It All Together
The default constructor warning in JDK 16 serves as a valuable prompt for developers to reevaluate their usage of default constructors and prioritize explicit constructor definitions. By heeding this warning and following the best practices outlined, developers can elevate the quality and clarity of their Java code. Embracing these principles ultimately contributes to a more resilient and comprehensible codebase.
In sum, addressing default constructor warnings in JDK 16 is an essential step towards fostering robust, explicit, and maintainable Java code.
For further insights on JDK 16 warnings and best practices in Java development, refer to the JDK 16 Documentation.
Checkout our other articles