Optimizing Google App Engine Staging for Performance
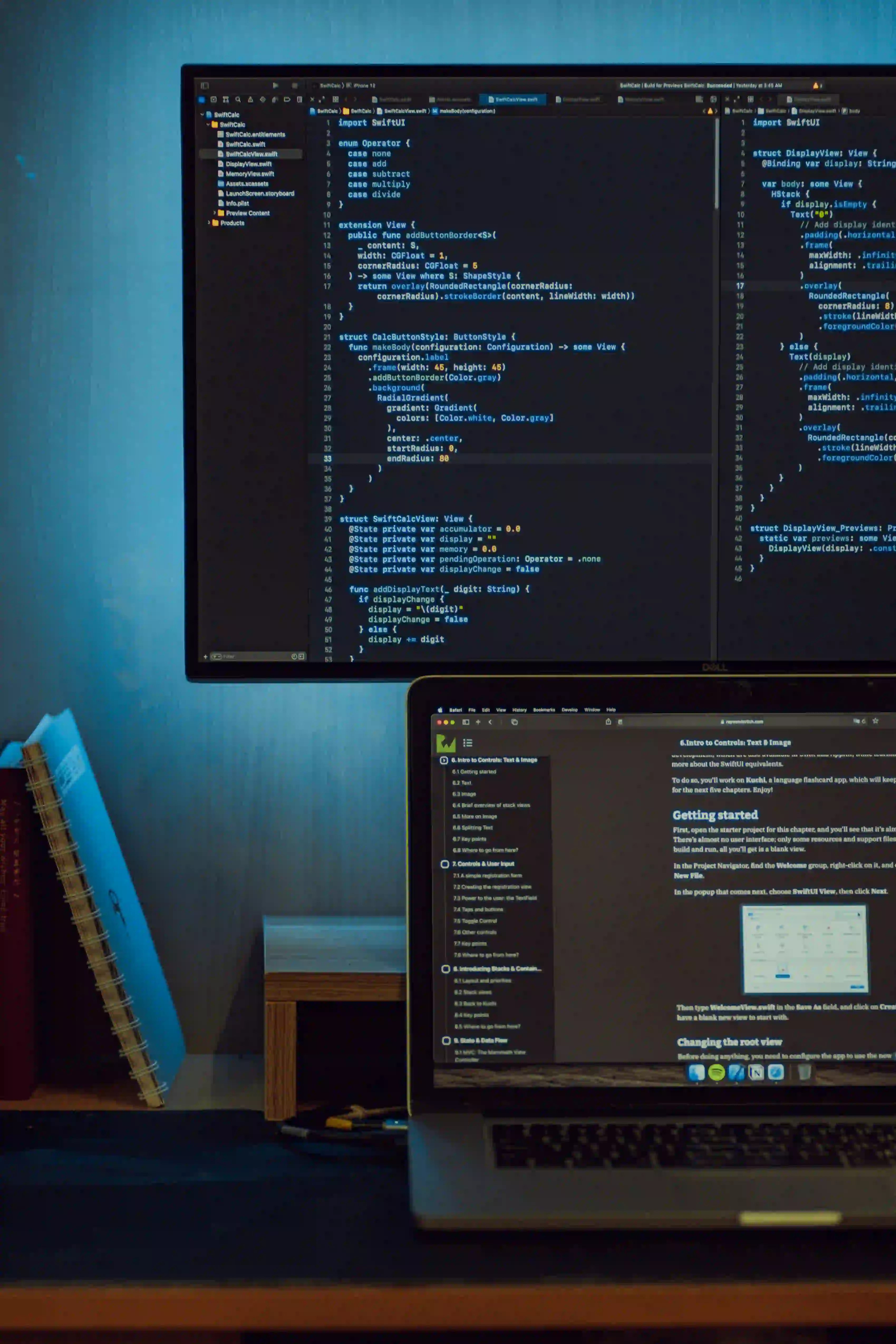
Optimizing Google App Engine Staging for Performance
Google App Engine is a Platform as a Service (PaaS) offering that allows developers to build and host applications on Google's infrastructure. It supports multiple programming languages, including Java, and provides a scalable, reliable, and low-latency environment for running web applications.
In this article, we will focus on optimizing the performance of Java applications hosted on Google App Engine by implementing best practices for staging. Staging is the process of preparing your application for deployment to a production environment. Optimizing staging can significantly improve the overall performance and user experience of your application.
Understanding Staging in Google App Engine
Staging in Google App Engine involves building and packaging your application for deployment. This process typically includes compiling source code, resolving dependencies, and creating deployment artifacts. Optimizing staging is crucial because it directly impacts the speed and efficiency of deployment, as well as the runtime performance of the application.
Optimizing Dependency Management
One of the key factors that can affect the performance of staging is dependency management. Efficiently managing dependencies can lead to faster build times and reduced deployment overhead. Here are a few tips for optimizing dependency management in a Java application:
Use Dependency Caching
Leverage build tools such as Maven or Gradle to incorporate dependency caching. Caching dependencies locally can significantly reduce the time required for resolving and downloading dependencies during the staging process. This can be achieved by utilizing local repository configurations and build optimizations.
<!-- Example of Maven settings.xml configuration for local repository -->
<settings>
<localRepository>/path/to/local/repository</localRepository>
</settings>
Dependency Exclusions
Carefully review the dependencies used in your project and consider excluding any unnecessary transitive dependencies. Unnecessarily bloated dependencies can impact both staging and runtime performance. Use tools like mvn dependency:tree
to inspect and analyze the dependency tree, and exclude any redundant or conflicting dependencies.
<!-- Example of excluding transitive dependencies using Maven -->
<dependency>
<groupId>com.example</groupId>
<artifactId>example-artifact</artifactId>
<exclusions>
<exclusion>
<groupId>com.unwanted</groupId>
<artifactId>unwanted-dependency</artifactId>
</exclusion>
</exclusions>
</dependency>
Compilation and Build Optimizations
Efficient compilation and build processes are crucial for improving staging performance. Here are some best practices to optimize compilation and build times for Java applications:
Incremental Compilation
Utilize build tools that support incremental compilation, such as Gradle with its continuous build feature. Incremental compilation allows for faster builds by only recompiling the source files that have changed since the last build, minimizing unnecessary recompilation overhead.
// Example Gradle configuration for continuous build
tasks.withType(JavaCompile) {
options.compilerArgs.addAll(['-g:source'])
}
Parallel Execution
Leverage build tools that support parallel execution of tasks to take advantage of multi-core processors. For example, Gradle provides built-in support for parallel task execution, allowing tasks to run concurrently and maximize resource utilization for faster build times.
// Example Gradle configuration for parallel task execution
tasks.withType(JavaCompile) {
options.fork = true
options.incremental = true
options.forkOptions.jvmArgs.addAll(['-Xmx4g', '-XX:MaxPermSize=1024m'])
}
Artifact Packaging and Distribution
The final stage of staging involves packaging the application into deployable artifacts. Efficient artifact packaging and distribution are critical for optimizing deployment times and ensuring a smooth transition to the production environment. Consider the following best practices for artifact packaging and distribution:
Lightweight Packaging
Strive to keep the size of deployable artifacts to a minimum by excluding unnecessary files and resources. For example, configure build tools to exclude test classes, documentation, and other non-essential files from the final artifact to reduce deployment payload and improve transfer times.
<!-- Example of excluding test classes in Maven -->
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<configuration>
<excludes>
<exclude>**/Test*.class</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
Artifact Compression
Consider compressing deployable artifacts to reduce the size of the deployment package. For instance, use tools like Apache Maven Assembly Plugin or Gradle's built-in zip task to create compressed artifact archives, which can expedite artifact transfer and reduce storage requirements.
// Example Gradle configuration for artifact compression
task zip(type: Zip) {
from 'build/distributions'
archiveFileName = 'my-application.zip'
destinationDir(new File(buildDir, 'archives'))
}
Bringing It All Together
In conclusion, optimizing Google App Engine staging for Java applications is crucial for achieving peak performance and efficient deployment. By implementing best practices for dependency management, compilation and build optimizations, and artifact packaging and distribution, you can significantly improve the speed, efficiency, and overall performance of your application on Google App Engine.
As a next step, consider exploring additional resources and documentation on Google App Engine and Java performance optimization to further enhance your understanding and proficiency in this area.
By following these best practices and constantly seeking to improve your staging processes, you can ensure that your Java applications on Google App Engine are consistently well-optimized for performance, delivering a superior user experience.