Effective Agile Testing Strategies
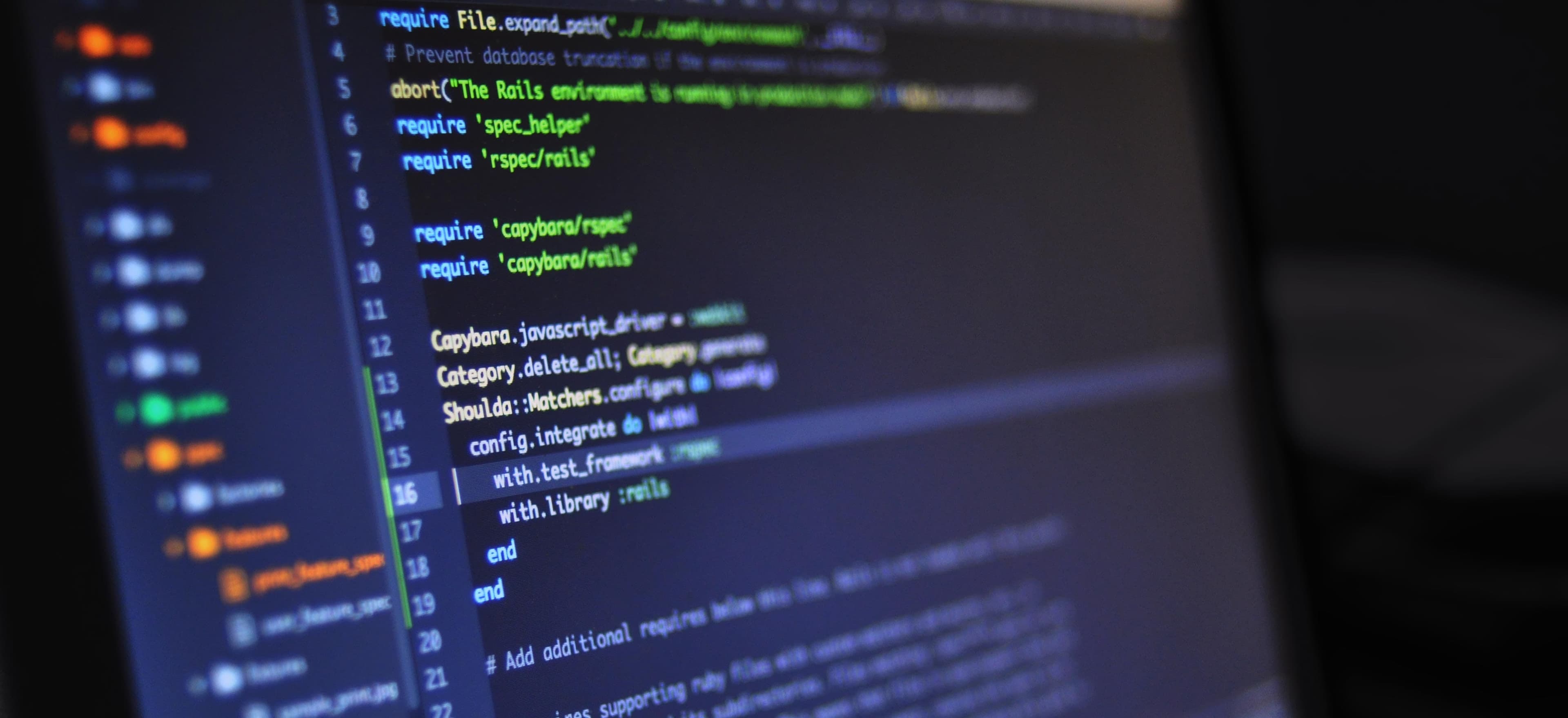
- Published on
Effective Agile Testing Strategies
In the world of software development, Agile has become the go-to methodology for many organizations. With its emphasis on iteration, collaboration, and flexibility, Agile has proven to be an effective approach for delivering high-quality software.
However, adopting Agile also brings about the need for robust testing strategies that can keep up with the rapid pace of development. In this blog post, we will explore some effective Agile testing strategies that can help teams ensure the quality and reliability of their software within the Agile framework.
Embracing Test-Driven Development (TDD)
One of the cornerstones of Agile testing is Test-Driven Development (TDD). TDD involves writing tests before writing the code, which helps in defining the expected behavior of the code. This practice not only ensures that the code meets the specified requirements but also acts as living documentation for the codebase.
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 5);
assertEquals(8, result);
}
Why: TDD fosters a laser focus on writing code that fulfills the required functionality. It also provides a safety net for refactoring and changing the code without the fear of unintended side effects.
Learn more: Test-Driven Development
Continuous Integration and Continuous Deployment (CI/CD)
In Agile development, the integration and deployment of code are continuous processes. Implementing a robust CI/CD pipeline that includes automated testing at various stages such as unit tests, integration tests, and end-to-end tests is crucial for ensuring the stability of the application as new features are added.
stage('Unit Test') {
sh 'mvn test'
}
stage('Integration Test') {
sh 'mvn verify'
}
Why: CI/CD ensures that any new code integrated into the codebase is immediately validated, and any issues are caught early in the development cycle, reducing the risk of integration conflicts and deployment failures.
Learn more: Continuous Integration vs. Continuous Delivery vs. Continuous Deployment
Automated Regression Testing
As Agile development involves frequent changes and additions to the codebase, the risk of regression issues is high. Implementing a comprehensive suite of automated regression tests helps in quickly identifying any unintended side effects of code changes.
@Test
public void testLoginFunctionality() {
// Simulate user login and check if the user is redirected to the dashboard
...
}
Why: Automated regression tests act as a safety net, allowing teams to make changes with confidence, knowing that existing functionalities have not been compromised.
Learn more: The Agile Testing Quadrants
Exploratory Testing
While automated tests are essential, they cannot uncover all possible scenarios and edge cases. Exploratory testing, where testers explore the application with minimal pre-defined scripts, aids in uncovering unforeseen issues and usability problems.
// Exploratory testing session to uncover usability issues in the shopping cart functionality
...
Why: Exploratory testing complements automated testing by focusing on uncovering issues that are hard to capture through automated scripts, thus providing a more comprehensive view of the application's quality.
Learn more: Exploratory Testing
Collaborative Testing
Agile emphasizes collaboration, and testing is no exception. In Agile teams, testers, developers, and product owners work closely together to ensure that the right tests are being conducted, and that the testing efforts align with the overall project goals.
// Joint review and testing session involving developers, testers, and product owners to ensure the feature meets all criteria
Why: Collaborative testing fosters a shared understanding of the application's requirements, leading to more effective testing efforts and higher quality software.
Learn more: Collaborative Testing
My Closing Thoughts on the Matter
In Agile development, testing is not just a phase but an integral part of the entire development process. By embracing Test-Driven Development, implementing robust CI/CD pipelines, using automated regression testing, supplementing with exploratory testing, and fostering collaborative testing efforts, Agile teams can ensure the delivery of high-quality software in a fast-paced and dynamic environment.
Adopting these strategies will help Agile teams maintain confidence in the quality of their code and facilitate the rapid and continuous delivery of value to customers.
Remember, in Agile, testing is not a separate phase, but an ongoing activity that runs parallel to development, ensuring that software is not only developed quickly but also built to the highest standards of quality and reliability.
So, how do you approach testing in your Agile environment? Share your thoughts and experiences!
#agiledev #testingstrategies #agiletesting #TDD #CI/CD #automatedtesting #exploratorytesting #collaborativetesting