Improving Performance of Android SlidingPaneLayout
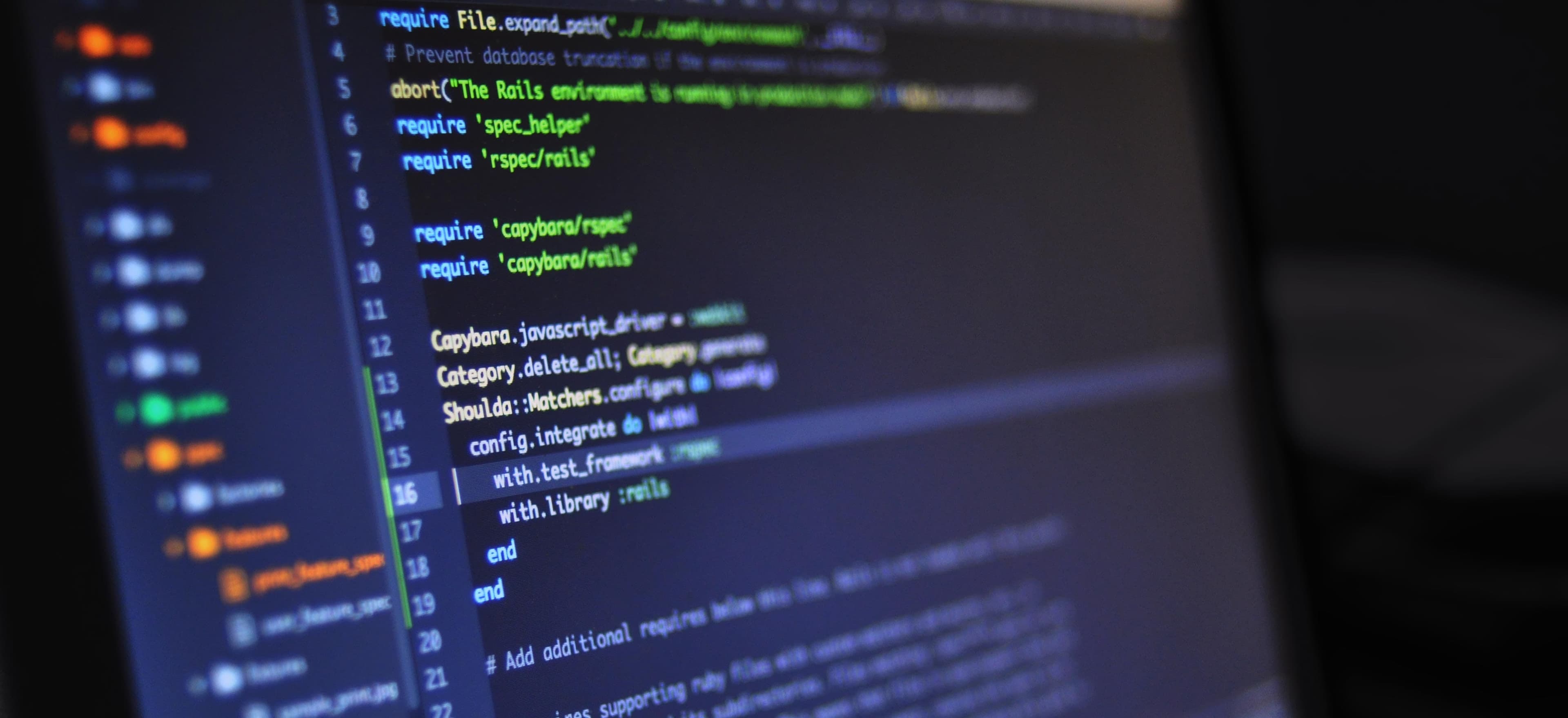
- Published on
Improving Performance of Android SlidingPaneLayout
SlidingPaneLayout is a useful component in Android for creating sliding panels, but it can sometimes suffer from performance issues, especially when dealing with complex UI and heavy interactions. In this blog post, we'll explore some tips and tricks to improve the performance of SlidingPaneLayout in your Android app.
Understanding SlidingPaneLayout
Before we dive into performance improvements, let's briefly discuss what SlidingPaneLayout is and how it's typically used in Android app development.
SlidingPaneLayout is a ViewGroup that helps in creating a multi-pane layout, where two views are split side by side, with one being the master pane and the other being the detail pane. It's commonly used in scenarios such as navigation drawers and split view interfaces.
Performance Challenges
While SlidingPaneLayout provides great functionality for building interactive UIs, it can face performance challenges, especially when handling complex layouts, large datasets, and frequent interactions. Some common performance issues include laggy animations, unresponsive touch events, and high CPU usage.
Tips to Improve Performance
1. Reduce Overdraw
Overdraw occurs when the same pixel on the screen is drawn multiple times in a single frame, which can significantly impact rendering performance. To reduce overdraw in SlidingPaneLayout, make sure to optimize your layout hierarchy and minimize the use of unnecessary backgrounds and transparency.
Example:
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FFFFFF"> <!-- Consider removing unnecessary background -->
...
</RelativeLayout>
2. Use Hardware Layers
Applying hardware acceleration to the SlidingPaneLayout and its child views can offload rendering operations to the GPU, resulting in smoother animations and improved performance. You can enable hardware acceleration at the activity level or on specific views using the android:layerType
attribute or by calling setLayerType()
in code.
Example:
slidingPaneLayout.setLayerType(View.LAYER_TYPE_HARDWARE, null);
3. Optimize View Rendering
In scenarios where the master pane of the SlidingPaneLayout contains complex views or heavy UI components, consider optimizing view rendering by using techniques such as view recycling, lazy loading, and reducing unnecessary view nesting.
Example:
// Implement view recycling for the master pane ListView
viewHolder.itemView.setOnClickListener(v -> {
// Handle item click
});
4. Implement Touch Event Optimization
When dealing with touch events in SlidingPaneLayout, optimize event handling to ensure smooth interaction. Avoid unnecessary event dispatching and processing, and consider implementing touch slop and velocity-based optimizations for drag gestures.
Example:
@Override
public boolean onInterceptTouchEvent(MotionEvent ev) {
// Implement touch event interception logic
return super.onInterceptTouchEvent(ev);
}
Key Takeaways
By applying the aforementioned tips and tricks, you can significantly improve the performance of SlidingPaneLayout in your Android app. Remember to profile your app using tools like Android Profiler to identify performance bottlenecks and measure the impact of your optimizations.
Incorporating these performance improvements will not only enhance the user experience but also contribute to the overall responsiveness and efficiency of your app.
For further insights into Android performance optimization, consider exploring Google's official Android Performance tips: Android Performance Overview
Start implementing these practices in your Android app today and witness a noticeable enhancement in the performance of your SlidingPaneLayout-based UIs. Happy coding!