Troubleshooting CDI Programmatic Dependency Disambiguation
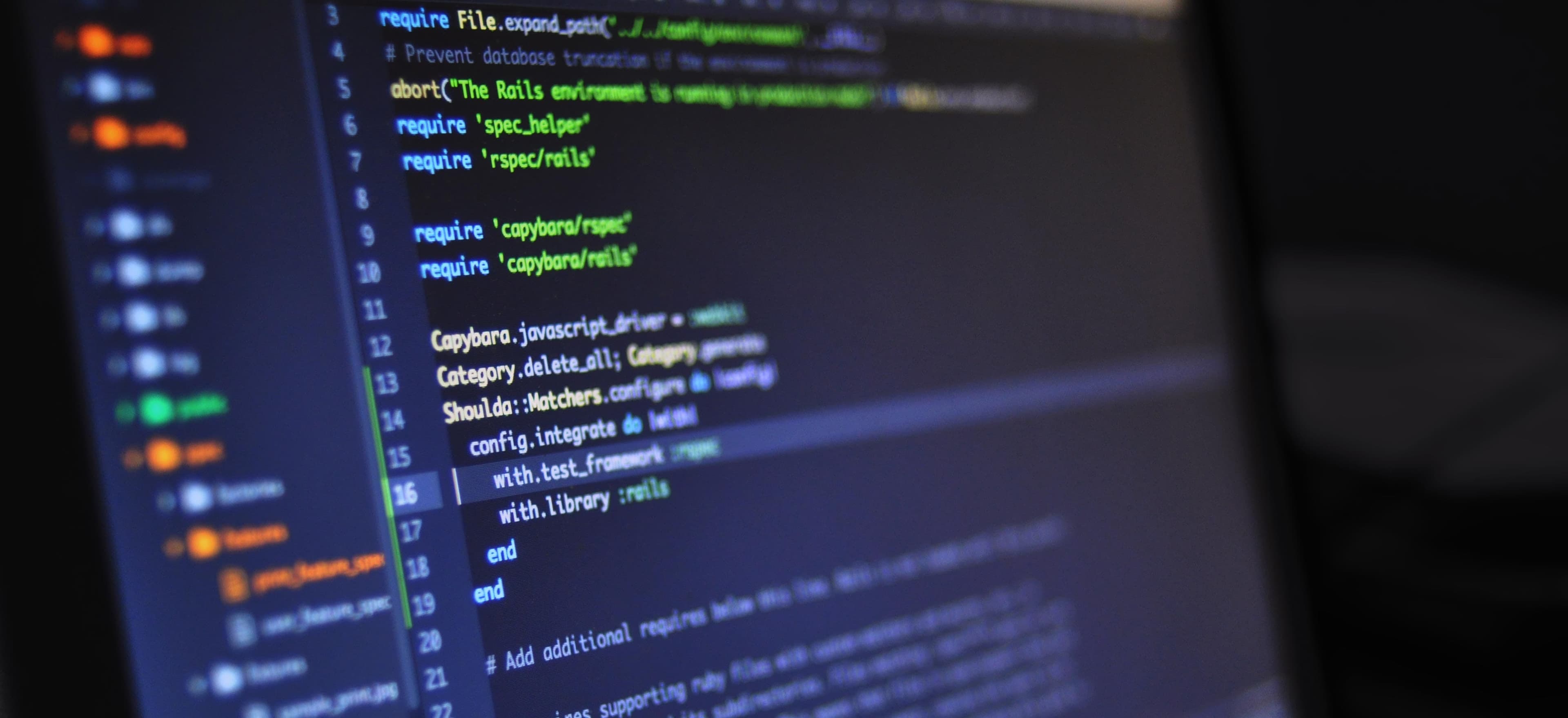
- Published on
Troubleshooting CDI Programmatic Dependency Disambiguation
Contexts and Dependency Injection (CDI) is a powerful Java EE standard for dependency injection and lifecycle management of Java components. It allows you to decouple components and manage their lifecycle without tight coupling. However, when it comes to programmatic dependency disambiguation in CDI, developers often face challenges in troubleshooting and resolving issues effectively.
In this article, we will explore common issues encountered during programmatic dependency disambiguation in CDI and how to troubleshoot and resolve them effectively.
Understanding Programmatic Dependency Disambiguation
In CDI, when there are multiple beans eligible for injection, you may face an ambiguous dependency resolution issue. CDI provides mechanisms to handle such situations programmatically through annotations like @Named
, @Any
, and @Default
. These annotations help in disambiguating the dependencies and instruct the CDI container on which bean to inject.
Let's dive into the common issues and their solutions related to programmatic dependency disambiguation in CDI.
Issue 1: AmbiguousResolutionException
One of the common issues encountered during programmatic dependency disambiguation is the AmbiguousResolutionException
. This exception occurs when the CDI container is unable to resolve the ambiguity between multiple eligible beans for injection.
Solution 1: Using the @Named Annotation
You can use the @Named
annotation to disambiguate the injection point. By annotating a bean with @Named
, you provide a unique name to the bean, which can be used in the injection point to specify the exact bean to be injected.
@Named("uniqueName")
public class MyBean {
// class implementation
}
@Inject
@Named("uniqueName")
private MyBean myBean;
Solution 2: Using the @Any Annotation
Another approach is to use the @Any
annotation, which allows CDI to inject any eligible bean.
@Inject
@Any
private Instance<MyBean> myBeans;
In this case, you can programmatically select the desired bean using the Instance
API provided by CDI.
Issue 2: UnsatisfiedResolutionException
Another issue that developers often encounter is the UnsatisfiedResolutionException
, which occurs when a bean cannot be resolved for injection.
Solution: Using the @Default Annotation
The @Default
annotation can be used to define a default bean for injection when there are multiple eligible beans.
@Default
public class DefaultBean implements MyBean {
// class implementation
}
By annotating a bean with @Default
, you specify it as the default candidate for injection when no other qualifier applies.
Issue 3: UnreachableResolutionException
The UnreachableResolutionException
may occur when the CDI container cannot reach a bean that needs to be injected.
Solution: Verifying Bean Accessibility
Ensure that the bean you are trying to inject is reachable and meets the necessary accessibility criteria. Check the scope, qualifiers, and conditions for the bean’s availability.
Wrapping Up
Programmatic dependency disambiguation is a powerful feature of CDI, but it can lead to various issues if not handled properly. By understanding the common issues and their solutions, you can effectively troubleshoot and resolve dependency disambiguation problems in your CDI applications.
Remember to use @Named
, @Any
, @Default
, and verify bean accessibility to overcome ambiguous, unsatisfied, and unreachable resolution issues in CDI.
Mastering programmatic dependency disambiguation in CDI will enhance your ability to build well-structured and maintainable Java EE applications.
Continue your learning journey by exploring more about CDI and JavaEE.