Managing Unsynced Persistence in JPA 2.1
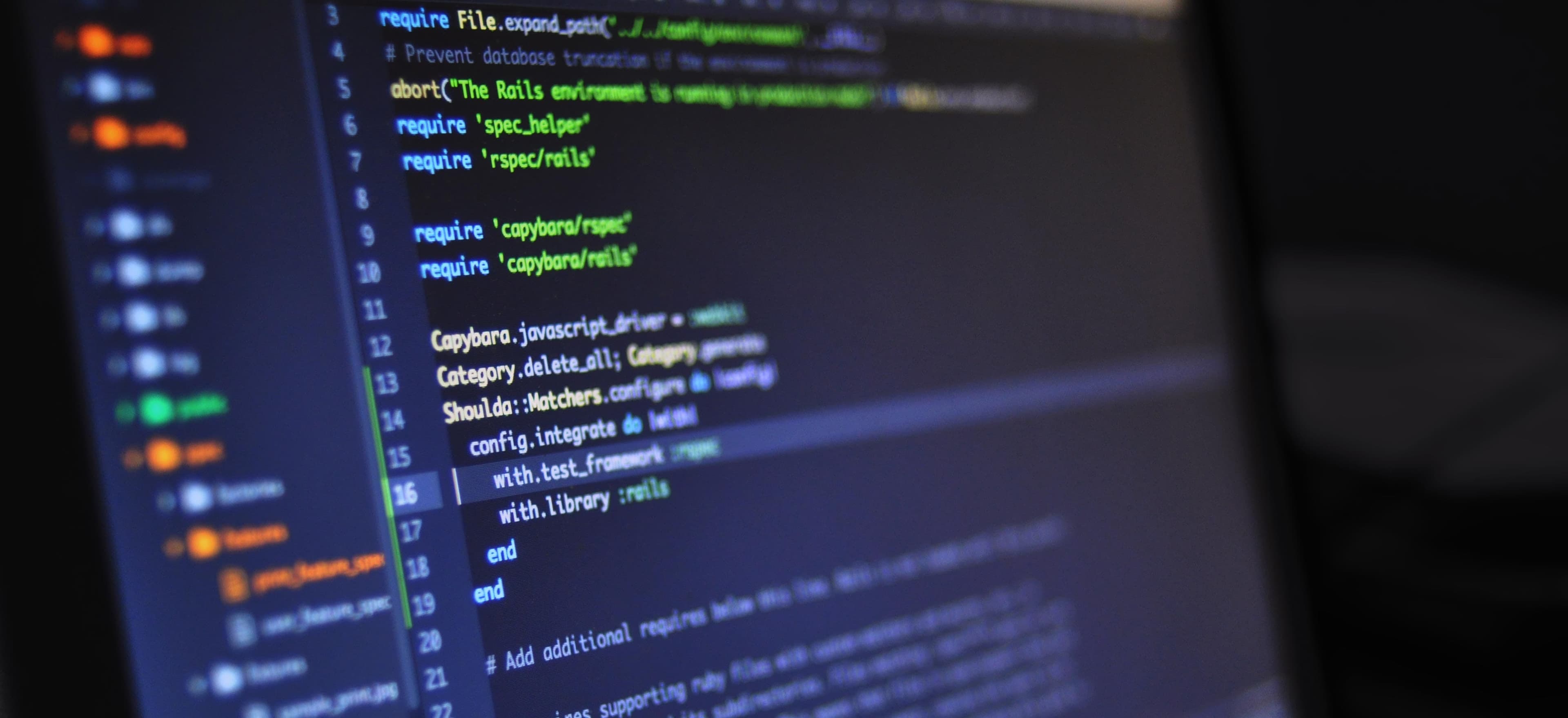
- Published on
Managing Unsynced Persistence in JPA 2.1
When working with Java Persistence API (JPA) 2.1, developers often encounter scenarios where entities are updated in the database outside of the application's managed context. This can result in inconsistencies between the database state and the in-memory entity state, leading to what is commonly referred to as "unsynced persistence."
In this blog post, we will explore the concept of unsynced persistence in JPA 2.1, discuss its implications, and provide best practices for effectively managing unsynced persistence to ensure data integrity within your Java applications.
Understanding Unsynced Persistence
Unsynced persistence occurs when the data stored in the database is different from the state of the entities managed by the JPA persistence context. This misalignment typically occurs when external processes or other applications modify the database records associated with JPA entities without the application's knowledge.
In JPA 2.1, entities are expected to be synchronized with the database when changes are made, either through explicit manipulation of entity objects or by relying on JPA's automatic dirty checking mechanism. However, when changes occur outside of the JPA context, these inconsistencies can arise.
Implications of Unsynced Persistence
Unsynced persistence can lead to various issues within an application, including:
-
Data Inconsistencies: Discrepancies between the in-memory entity state and the database state can lead to incorrect or outdated data being used within the application.
-
Concurrency Problems: Uncontrolled updates to database records can conflict with changes made within the application, potentially leading to data corruption or lost updates.
-
Unexpected Behavior: Operations such as cascading updates or deletions may not function as expected when entities are not properly synchronized with the database.
Best Practices for Managing Unsynced Persistence
To address the challenges posed by unsynced persistence in JPA 2.1, consider implementing the following best practices:
1. Optimistic Locking
Optimistic locking is a mechanism that prevents conflicting updates to database records by leveraging version control. By annotating entity fields with @Version
, JPA automatically manages a version attribute and utilizes it to detect concurrent modifications. When an entity is updated, JPA verifies the version to ensure that the data has not changed since it was initially retrieved. If a concurrent modification is detected, an OptimisticLockException
is thrown, allowing the application to handle the conflict appropriately.
@Entity
public class Product {
@Id
private Long id;
// Other fields
@Version
private Long version; // Version attribute for optimistic locking
}
2. Refreshing Entities
In situations where unsynced persistence is suspected, you can explicitly refresh entities from the database to discard any changes made outside of the application's context. The EntityManager
provides the refresh
method, which enables a developer to reload the state of a managed entity from the database.
Product product = entityManager.find(Product.class, productId);
// ... external updates may have occurred
entityManager.refresh(product); // Reload entity state from the database
3. Detaching and Merging Entities
When dealing with long-running conversations or disconnected scenarios, detaching and merging entities can help manage unsynced persistence. By detaching an entity from the persistence context, it becomes unaffected by changes made within the application, allowing it to be reattached and synchronized by merging it back when necessary.
Product product = entityManager.find(Product.class, productId);
entityManager.detach(product); // Detach the entity from the persistence context
// ... perform operations without affecting the detached entity
entityManager.merge(product); // Merge the detached entity back into the persistence context
4. Implementing Custom Synchronization Logic
For more complex scenarios, implementing custom synchronization logic tailored to the specific requirements of the application can effectively manage unsynced persistence. This may involve leveraging database triggers, change data capture mechanisms, or incorporating event-driven architectures to react to external changes and ensure data consistency.
5. Avoiding Long Lived Entity Managers
Using a long-lived EntityManager
increases the likelihood of encountering unsynced persistence due to extended periods of entity management. Using a short-lived EntityManager
within well-defined transaction boundaries can minimize the risk of inconsistencies and facilitate better state management.
Closing Remarks
Managing unsynced persistence in JPA 2.1 is crucial for ensuring data integrity and consistent behavior within Java applications. By understanding the implications of unsynced persistence and implementing best practices such as optimistic locking, entity refreshing, detaching and merging entities, custom synchronization logic, and using short-lived EntityManager
instances, developers can effectively mitigate the challenges associated with unsynced persistence.
Establishing robust strategies for managing unsynced persistence not only safeguards the application's data integrity but also promotes reliable and predictable behavior in the face of concurrent updates and external modifications. By adhering to these best practices, JPA developers can confidently navigate the complexities of unsynced persistence and build resilient, high-quality applications.
For further reading on managing persistence in JPA, consider exploring the official documentation on Java Persistence API (JPA) and the JPA specification for a comprehensive understanding of persistence management techniques and best practices.
Thank you for reading! If you found this post helpful, feel free to share it with your colleagues and peers. Happy coding!