Avoiding N+1 Query Problems in Bulk Fetching with Hibernate
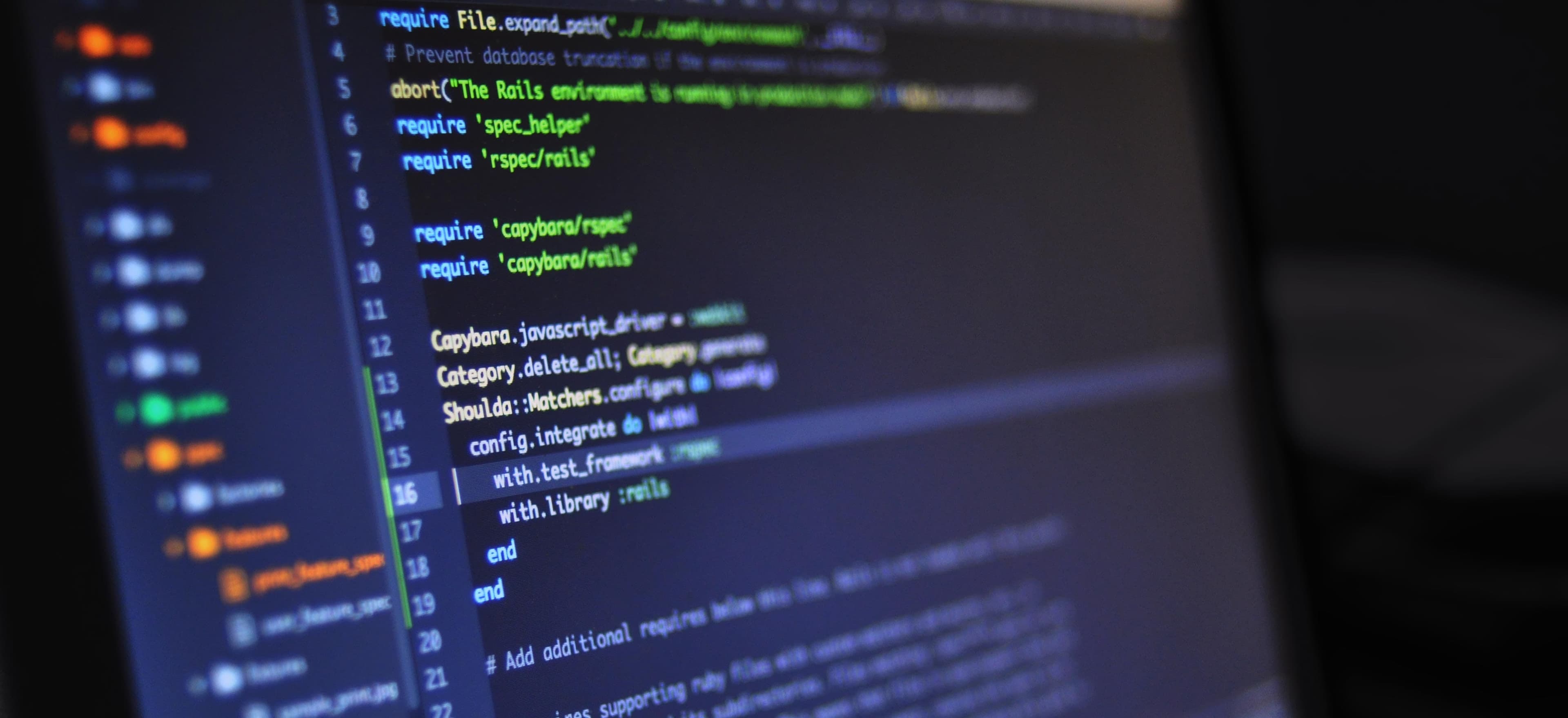
- Published on
Avoiding N+1 Query Problems in Bulk Fetching with Hibernate
In modern web application development, efficiency and performance are pivotal. One of the common causes of performance bottlenecks when using an Object-Relational Mapping (ORM) tool like Hibernate is the N+1 query problem. This issue arises when you load a collection of entities, and for each of these entities, a separate query is executed to retrieve associated entities. The N+1 query problem can drastically increase database load and slow down your application.
In this blog post, we'll explore the N+1 query problem, how to identify it, and techniques to avoid it, particularly focusing on bulk fetching strategies in Hibernate.
Understanding the N+1 Query Problem
The N+1 query problem occurs when fetching a list of entities (lets say N entities) along with their related entities. Instead of one query to fetch all data, the application sends one query for the main entities followed by N additional queries, one for each entity's associated data.
Here’s an illustration:
- Fetch all
Author
records: SELECT * FROM Author (1 query) - For each author, fetch their
Books
: SELECT * FROM Books WHERE author_id = ? (N queries)
This leads to N + 1 queries—hence the term.
Example Query Breakdown
Suppose you want to retrieve all authors and their books; the naive approach would result in multiple database hits. Here is what it may look like:
List<Author> authors = session.createQuery("FROM Author").list(); // 1 Query
for (Author author : authors) {
List<Book> books = author.getBooks(); // N Queries
}
If there are 100 authors, you’ll end up executing 101 queries. This is not efficient!
Detecting N+1 Query Problems
To first mitigate the problem, you need to be able to detect it. Tools like Hibernate's statistics, and SQL logging can be beneficial. Ensure you have logging enabled in your Hibernate configuration:
hibernate.show_sql=true
hibernate.format_sql=true
hibernate.use_sql_comments=true
You may also use:
Statistics stats = sessionFactory.getStatistics();
stats.setStatisticsEnabled(true);
System.out.println("Queries executed: " + stats.getQueryExecutionCount());
This way, you can monitor how many SQL queries your application runs and identify potential N+1 issues.
Strategies to Avoid N+1 Queries
To eliminate N+1 query problems, you can utilize several strategies including:
- Eager Fetching: Loading associated entities at the same time as the parent entity.
- Batch Fetching: Fetching collections in batches rather than individually.
- Join Fetching: Using HQL or Criteria API to fetch entities with their associations in one go.
1. Eager Fetching
Eager fetching is a strategy where the associated entities are loaded at the same time as the main entity. This can be achieved using the @OneToMany
or @ManyToMany
annotations with fetch = FetchType.EAGER
in your entity classes.
@Entity
public class Author {
@Id
private Long id;
@OneToMany(fetch = FetchType.EAGER)
private List<Book> books;
// getters and setters
}
When you now query authors, Hibernate will load books simultaneously, preventing additional queries.
Downsides of Eager Fetching
However, be cautious; eager fetching can lead to loading unwanted data, which can impact performance and memory. Always prefer this when you need relationships frequently.
2. Batch Fetching
Batch fetching allows you to load a set of collections in chunks. You can configure the batch size using the @BatchSize
annotation.
@OneToMany
@BatchSize(size = 10)
private List<Book> books;
In this example, Hibernate loads the associated books in batches of 10, dramatically reducing the number of database calls.
Example Code
Here’s a code illustration:
@Entity
public class Author {
@Id
private Long id;
@OneToMany
@BatchSize(size = 10)
private List<Book> books;
// other fields, getters, and setters
}
When you fetch Authors, Hibernate will issue fewer queries by grouping associations, thus enhancing performance.
3. Join Fetching
Join fetching combines the selection of an entity with the selection of its related entities. This can be efficiently executed through HQL or Criteria API.
Using HQL
List<Author> authors = session.createQuery(
"SELECT a FROM Author a JOIN FETCH a.books").getResultList();
This results in a single query that retrieves all authors along with their books through a LEFT JOIN.
Example Code Using Criteria API
Using the Criteria API:
CriteriaBuilder cb = session.getCriteriaBuilder();
CriteriaQuery<Author> cq = cb.createQuery(Author.class);
Root<Author> author = cq.from(Author.class);
author.fetch("books", JoinType.LEFT);
cq.select(author);
List<Author> authors = session.createQuery(cq).getResultList();
Both methods solve the N+1 problem effectively, but remember that joining too many tables can create dense and complex result sets, possibly impacting performance.
Closing Remarks
The N+1 query problem can severely hinder the performance of applications using Hibernate, but understanding and employing strategies such as eager fetching, batch fetching, and join fetching can mitigate this issue. Always profile your queries and adopt an approach that balances load and performance.
For more in-depth information on Hibernate and optimizing performance, check out these resources:
- Hibernate Documentation
- Hibernate Performance Tuning
By leveraging the techniques outlined in this post, you can enhance your application’s performance, ensuring it scales gracefully as user demand grows. Happy coding!