Optimizing Performance in LibGDX Game Development
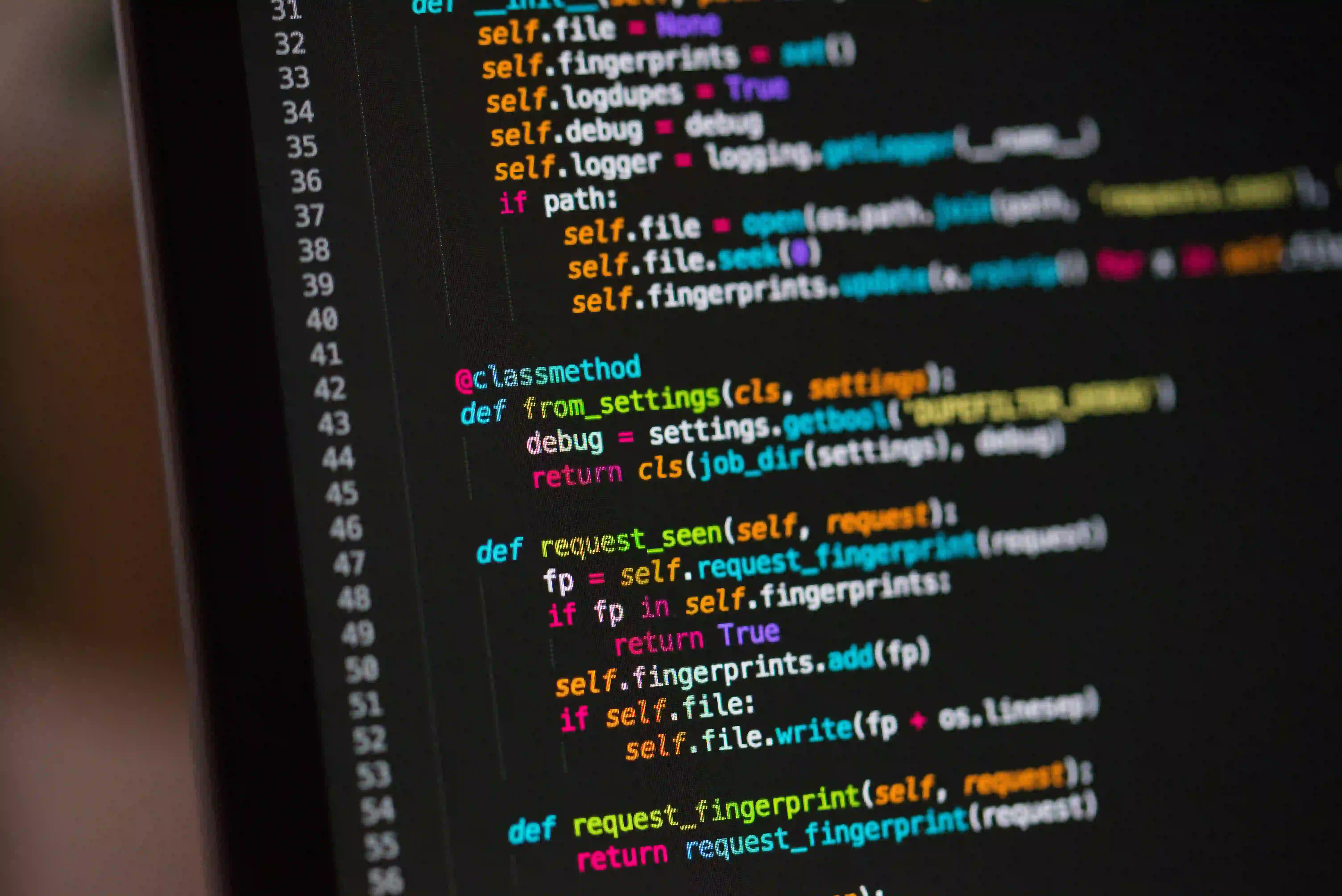
Optimizing Performance in LibGDX Game Development
LibGDX is a popular open-source game development framework that allows developers to write games in Java and deploy them across different platforms. While the framework provides powerful tools and features for game development, it's essential to optimize the performance of a game built with LibGDX to ensure a smooth and engaging user experience.
In this blog post, we'll explore various techniques and best practices for optimizing performance in LibGDX game development. We'll cover topics such as efficient rendering, memory management, and performance profiling. By the end of this post, you'll have a solid understanding of how to make your LibGDX games run faster and more efficiently.
Efficient Rendering
Rendering is a crucial aspect of game development, and inefficient rendering can significantly impact the performance of your game. In LibGDX, rendering is typically done using the SpriteBatch
class, which allows you to draw 2D images and textures. To optimize rendering performance, consider the following best practices:
Sprite Packing
Sprite packing involves combining multiple textures into a single larger texture, reducing the number of draw calls required to render them. LibGDX provides the TexturePacker
tool, which allows you to pack individual images into a single texture atlas. Using texture atlases can significantly reduce the overhead of switching between textures during rendering.
// Example of using a texture atlas in LibGDX
TextureAtlas textureAtlas = new TextureAtlas(Gdx.files.internal("textures.atlas"));
Sprite Batching
Sprite batching involves grouping together similar sprites and drawing them in a single draw call. This can be achieved by using the SpriteBatch
class to draw multiple sprites at once. Minimizing the number of draw calls can greatly improve rendering performance, especially on mobile and other resource-constrained devices.
// Example of sprite batching with SpriteBatch in LibGDX
spriteBatch.begin();
// Draw multiple sprites here
spriteBatch.end();
Use of Meshes and Shaders
For more advanced rendering effects, consider using meshes and shaders in LibGDX. Meshes allow you to define custom geometry for rendering, while shaders enable you to apply complex visual effects to your sprites. While these techniques can add visual flair to your game, they should be used judiciously to avoid unnecessary performance overhead.
Memory Management
Efficient memory management is critical for optimal performance in game development. In Java, memory management is handled by the garbage collector, but excessive garbage collection can lead to hiccups and stutters in your game. To optimize memory usage in your LibGDX game, consider the following strategies:
Object Pooling
Object pooling is a technique where a pool of reusable objects is maintained to avoid the overhead of object creation and garbage collection. In LibGDX, object pooling can be particularly beneficial for frequently used objects such as bullets, enemies, or particles.
// Example of object pooling in LibGDX
ObjectPool<Bullet> bulletPool = new ObjectPool<>(Bullet::new, 10);
Bullet bullet = bulletPool.obtain();
// Use the bullet
bulletPool.free(bullet);
Asset Management
Proper asset management is crucial for minimizing memory usage in a LibGDX game. Load and unload assets such as textures, sounds, and music as needed to conserve memory. LibGDX provides the AssetManager
class, which allows you to efficiently manage game assets and their lifecycles.
// Example of asset management using AssetManager in LibGDX
AssetManager assetManager = new AssetManager();
assetManager.load("background.png", Texture.class);
assetManager.finishLoading();
Texture background = assetManager.get("background.png");
// Dispose unused assets when no longer needed
assetManager.unload("background.png");
Performance Profiling
Performance profiling is the process of analyzing and measuring the performance of your game to identify bottlenecks and areas for improvement. In LibGDX, you can use various tools and techniques for performance profiling, such as:
ApplicationListener's render Method
The render
method in your game's ApplicationListener
implementation is a good place to start measuring performance. Use a profiling tool or performance monitor to analyze the time spent in the render
method and identify any performance bottlenecks.
LibGDX Performance Profiling Tools
LibGDX provides a set of performance profiling tools, such as the PerformanceCounter
class, which allows you to measure the time taken by specific code sections. Additionally, tools like the FPSLogger
class can help track the frame rate of your game.
// Example of using PerformanceCounter in LibGDX for profiling
PerformanceCounter renderCounter = new PerformanceCounter("Render");
renderCounter.start();
// Code to be profiled
renderCounter.stop();
External Profiling Tools
Consider using external profiling tools such as Java Flight Recorder, VisualVM, or third-party profilers to gather detailed performance metrics and identify performance hotspots in your LibGDX game.
Key Takeaways
Optimizing performance in LibGDX game development is essential for creating smooth and responsive games. By implementing efficient rendering techniques, optimizing memory management, and using performance profiling tools, you can significantly improve the performance of your LibGDX games.
In conclusion, mastering performance optimization in LibGDX requires a combination of best practices, tools, and a keen understanding of how the framework handles rendering, memory, and performance. By incorporating these strategies into your game development workflow, you can deliver high-performance games that provide an enjoyable experience for players across different platforms.
Remember, performance optimization is an ongoing process, and it's essential to continuously monitor and fine-tune your game for the best possible performance.
Now, go forth and create blazing-fast games with LibGDX! Happy optimizing!
References: