Improving Technical Skills: Key to Success
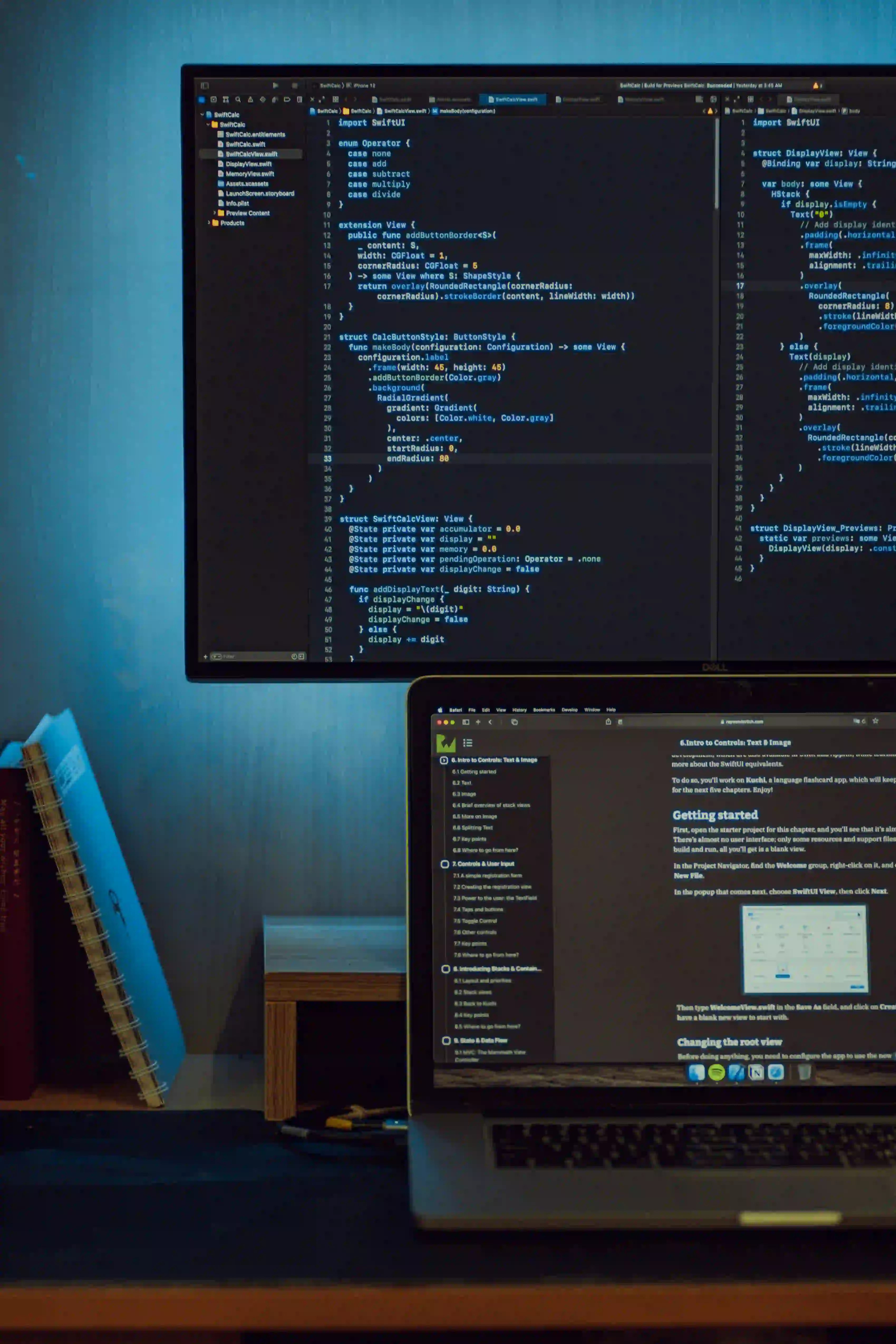
The Importance of Improving Your Java Programming Skills
As a Java developer, it's essential to continually enhance your skills to stay competitive in the dynamic world of software development. Whether you're a seasoned programmer or just starting with Java, the journey of learning and improvement never ends. In this post, we'll explore the significance of improving your Java programming skills, along with practical tips to help you along the way.
Embracing Continuous Learning
The field of technology is characterized by its rapid evolution. As new technologies, tools, and best practices emerge, it's crucial to stay abreast of these advancements. By upgrading your Java skills, you not only maintain your relevance in the industry but also pave the way for exciting career opportunities.
Mastering Core Java Concepts
A strong foundation in core Java concepts forms the backbone of proficient programming. Understanding topics such as data types, loops, and object-oriented programming principles is essential for writing efficient and maintainable code. Constantly revisiting these concepts and exploring advanced nuances will undoubtedly enhance your programming prowess.
public class HelloJava {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
In the snippet above, the HelloJava
class demonstrates the simplest form of a Java program. Understanding the nuances of this fundamental structure is vital for building more complex applications.
Leveraging Design Patterns and Best Practices
Familiarizing yourself with design patterns empowers you to write scalable and maintainable code. Whether it's the creational patterns like Singleton or structural patterns like Decorator, each pattern serves a distinct purpose in software design. Mastering these patterns not only enhances your problem-solving abilities but also sets you apart as a proficient Java developer.
public class Singleton {
private static Singleton instance;
private Singleton() {}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
The Singleton pattern, as depicted above, ensures that a class has only one instance and provides a global point of access to it. Understanding and implementing such patterns can greatly improve the quality and efficiency of your codebase.
Embracing Modern Frameworks and Libraries
The Java ecosystem is replete with libraries and frameworks that streamline development and empower you to build robust applications. Whether it's Spring for enterprise applications, Hibernate for database interactions, or Guava for core libraries, familiarizing yourself with these tools can significantly boost your productivity.
Integrating a framework like Spring allows you to create loosely coupled, reusable code while leveraging dependency injection and aspect-oriented programming. Such practices enhance the maintainability and testability of your codebase, marking you as a proficient Java developer.
Code Refactoring and Optimization
Improving your Java skills also entails honing the art of refactoring and optimizing existing code. As you gain experience, you'll realize that writing functional code is merely the first step; refining and enhancing it through strategic refactoring is equally essential.
To illustrate, consider the following code snippet:
public class Calculator {
public int add(int num1, int num2) {
return num1 + num2;
}
}
While the add
method is functional, optimizing it through the use of data structures or algorithmic improvements can elevate your coding abilities to the next level.
The Role of Testing and Debugging
Quality assurance is an integral part of software development. By mastering testing frameworks like JUnit and Mockito, you can ensure that your code is not only functional but also resilient to unforeseen scenarios. Additionally, adept debugging skills are invaluable in identifying and resolving issues within your codebase.
Engaging with the Java Community
The Java developer community is vibrant and resourceful. Engaging with forums, attending meetups, and participating in hackathons not only exposes you to diverse perspectives but also provides opportunities for networking and knowledge exchange. The insights gained from interactions within the community can catalyze your learning journey and propel you towards excellence.
Key Takeaways
In conclusion, the significance of improving your Java skills cannot be overstated. It is a continual process that demands commitment, curiosity, and a thirst for knowledge. By mastering core concepts, embracing best practices, and staying attuned to industry trends, you position yourself as an adept Java developer capable of tackling complex challenges with finesse.
So, embark on this journey of constant improvement, for in the realm of Java programming, honing your skills is not just a choice but a requisite for success.
Remember, the only way to do great work is to love what you do. So, embrace the art of Java programming, and let your passion fuel your quest for mastery.