Deadlock Prevention with Reentrant Locks
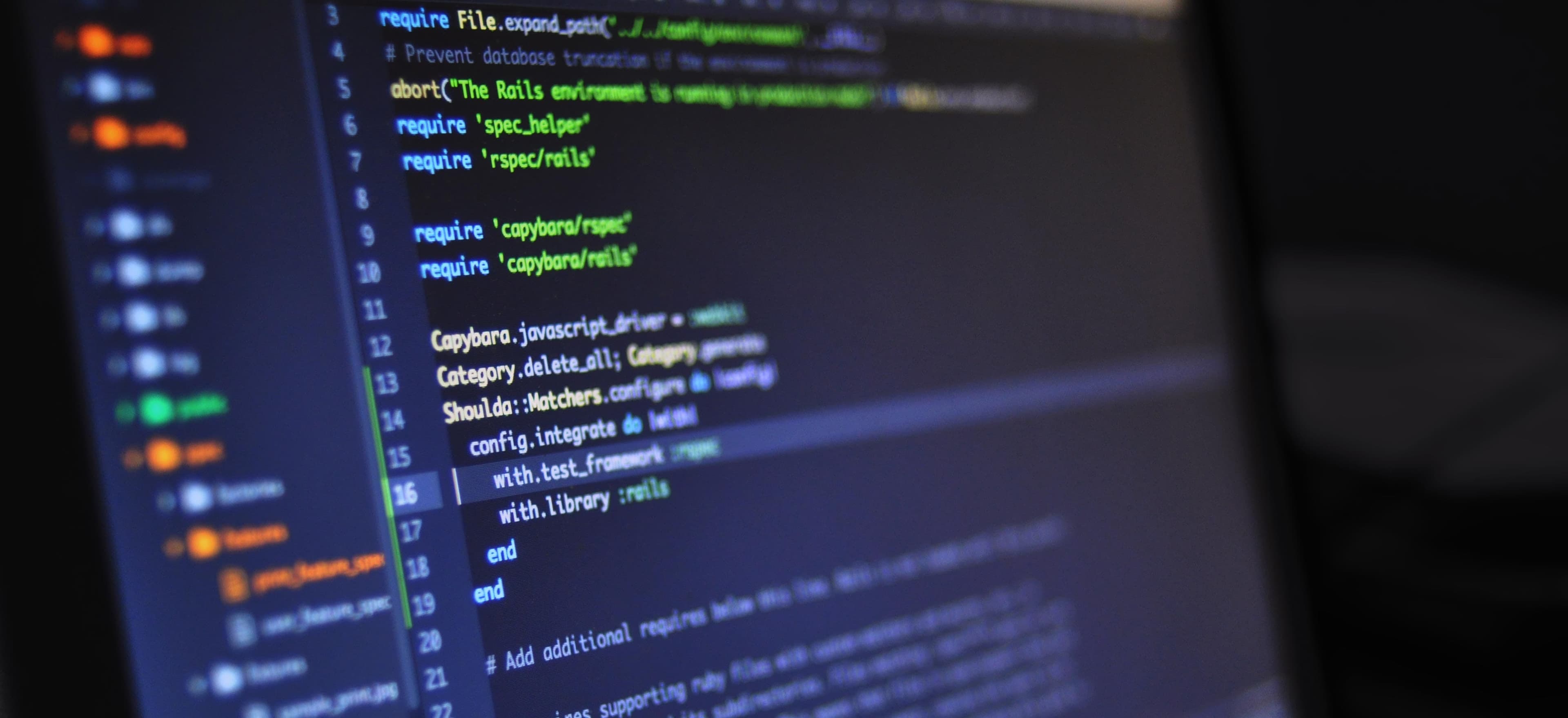
- Published on
Deadlock Prevention with Reentrant Locks
Deadlock is a common issue in multi-threaded applications. It occurs when two or more threads are blocked forever, waiting for each other. In Java, one way to prevent deadlock is by using the ReentrantLock class.
Understanding Reentrant Locks
Java's ReentrantLock is a powerful alternative to the traditional synchronized keyword for managing access to shared resources in a multi-threaded environment. It allows greater control over locking behavior and provides additional features not available with intrinsic locks.
Example of Using Reentrant Locks
import java.util.concurrent.locks.ReentrantLock;
public class SharedResource {
private final ReentrantLock lock = new ReentrantLock();
public void accessResource() {
lock.lock();
try {
// critical section
} finally {
lock.unlock();
}
}
}
In the above example, the lock
object is used to control access to the critical section. The lock()
method is called to acquire the lock, and the unlock()
method is called in a finally block to release the lock, ensuring that it is released even if an exception occurs.
Deadlock Prevention with Reentrant Locks
Now, let's dive into how Reentrant Locks can be used to prevent deadlock in Java.
Use tryLock() with timeout
One approach to preventing deadlock is to use the tryLock()
method with a timeout. This allows a thread to attempt to acquire the lock, but if the lock is not available within a specified time, the thread can choose to do something else rather than waiting indefinitely.
public class SharedResource {
private final ReentrantLock lock1 = new ReentrantLock();
private final ReentrantLock lock2 = new ReentrantLock();
public void accessResources() {
while (true) {
if (lock1.tryLock()) {
try {
if (lock2.tryLock()) {
try {
// critical section
break;
} finally {
lock2.unlock();
}
}
} finally {
lock1.unlock();
}
}
// Do something else to avoid deadlock
}
}
}
In this example, the tryLock()
method is used to attempt to acquire the locks lock1
and lock2
. If the lock acquisition fails, the thread can choose to do something else to avoid deadlock.
Using Lock Ordering
Another approach to preventing deadlock with Reentrant Locks is to establish a lock acquisition order and ensure that all threads follow the same order when acquiring multiple locks.
public class SharedResource {
private final ReentrantLock lock1 = new ReentrantLock();
private final ReentrantLock lock2 = new ReentrantLock();
public void accessResources() {
// Acquire locks in a consistent order
if (lock1.tryLock()) {
try {
if (lock2.tryLock()) {
try {
// critical section
} finally {
lock2.unlock();
}
}
} finally {
lock1.unlock();
}
}
// Handle the situation when lock1 is not acquired
}
}
By following a consistent order of lock acquisition, it is possible to avoid cyclic dependencies between locks, which is a common cause of deadlock.
Using lockInterruptibly()
The lockInterruptibly()
method allows a thread to acquire the lock unless the thread is interrupted. This can be useful for preventing deadlock by allowing a thread to be interrupted while waiting for a lock, thus avoiding indefinite blocking.
public class SharedResource {
private final ReentrantLock lock1 = new ReentrantLock();
private final ReentrantLock lock2 = new ReentrantLock();
public void accessResources() throws InterruptedException {
lock1.lockInterruptibly();
try {
lock2.lockInterruptibly();
try {
// critical section
} finally {
lock2.unlock();
}
} finally {
lock1.unlock();
}
}
}
In the above example, the lockInterruptibly()
method is used to acquire the locks, allowing the thread to be interrupted if it is waiting for the lock.
Wrapping Up
Deadlock prevention is a critical aspect of multi-threaded programming. Java's Reentrant Locks provide powerful mechanisms to prevent deadlock, including using tryLock()
with timeout, lock ordering, and lockInterruptibly()
. By understanding and implementing these techniques, developers can create more robust and reliable multi-threaded applications.
For further reading on Reentrant Locks and deadlock prevention, check out the official Java documentation and Concurrency in Java by Brian Goetz.