Maximizing Code Reusability with Decorator Design Pattern
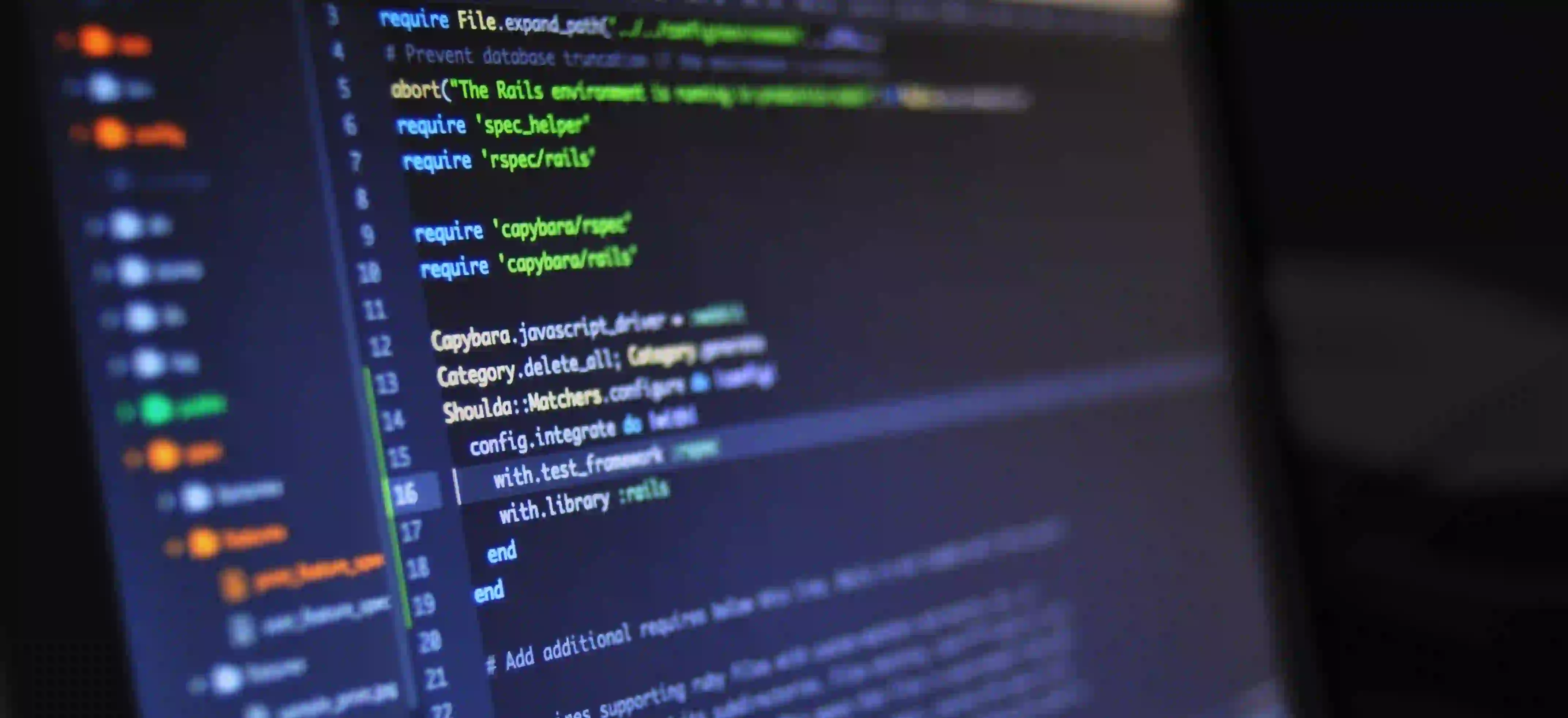
Maximizing Code Reusability with Decorator Design Pattern
In software development, one of the key principles is maximizing code reusability. This not only reduces development time but also leads to a more maintainable codebase. The Decorator design pattern is an essential tool in achieving this goal. It allows you to add functionality to objects dynamically, providing an alternative to subclassing for extending functionality. In this article, we will explore the Decorator design pattern in Java, its implementation, and how it can help in maximizing code reusability.
Understanding the Decorator Design Pattern
The Decorator pattern is a structural design pattern that allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. This pattern is particularly useful when you need to add or alter the functionality of objects at runtime.
At its core, the Decorator pattern involves creating a set of decorator classes that are used to wrap concrete components. These decorators add new functionality to the objects they wrap. The key benefit of this approach is that it allows for flexible and easy customization of individual objects without impacting the behavior of other objects of the same class.
Example Use Case: Coffee Order System
Suppose we are developing a coffee order system, and we want to provide the option for adding additional ingredients such as milk, sugar, or whipped cream to the base coffee. Using the Decorator pattern, we can create decorators for each additional ingredient, enabling dynamic customization of the coffee orders without the need for an explosion of subclasses.
Implementing the Decorator Design Pattern in Java
Let’s dive into a practical implementation of the Decorator design pattern in Java. Below is a simplified example where we have a base interface Coffee
representing the core functionality, and concrete implementations such as SimpleCoffee
and MilkCoffee
as well as decorator classes like CoffeeDecorator
.
// Base interface representing the core functionality
public interface Coffee {
double getCost();
String getIngredients();
}
// Concrete implementation of the base interface
public class SimpleCoffee implements Coffee {
@Override
public double getCost() {
return 2;
}
@Override
public String getIngredients() {
return "Coffee";
}
}
// Decorator class
public abstract class CoffeeDecorator implements Coffee {
protected final Coffee decoratedCoffee;
public CoffeeDecorator(Coffee decoratedCoffee) {
this.decoratedCoffee = decoratedCoffee;
}
public double getCost() {
return decoratedCoffee.getCost();
}
public String getIngredients() {
return decoratedCoffee.getIngredients();
}
}
// Concrete decorator, in this case for adding milk
public class MilkCoffee extends CoffeeDecorator {
public MilkCoffee(Coffee decoratedCoffee) {
super(decoratedCoffee);
}
public double getCost() {
return super.getCost() + 0.5;
}
public String getIngredients() {
return super.getIngredients() + ", Milk";
}
}
In this example, the CoffeeDecorator
class acts as the base decorator with MilkCoffee
representing a specific decorator. The getCost
and getIngredients
methods are overridden in the concrete decorator to modify the behavior by adding the cost and ingredients of the additional milk.
By using the Decorator pattern, we have created a flexible way to extend the functionality of our core Coffee
object without the need for multiple subclasses. This approach is highly valuable in scenarios where we need to dynamically customize the behavior of objects at runtime.
Benefits of Using the Decorator Design Pattern
The Decorator pattern provides several benefits, including:
-
Code Reusability: By using decorators, you can add new functionality to an object without altering its structure, promoting code reusability and minimizing code duplication.
-
Flexible Extension: The Decorator pattern allows for a flexible and dynamic way to add or modify object behavior at runtime, providing more options for customization.
-
Simplified Maintenance: With the Decorator pattern, adding new features or modifying existing ones becomes more straightforward and does not impact other parts of the codebase, making maintenance easier.
Additional Resources
To further explore the Decorator design pattern and its application in Java, check out these resources:
- The Decorator Pattern - Design Patterns: Elements of Reusable Object-Oriented Software
- Decorator Design Pattern in Java
Key Takeaways
The Decorator design pattern is a powerful tool for maximizing code reusability in Java applications. By allowing the dynamic addition of new functionality to objects, it promotes a more flexible and maintainable codebase. Understanding and leveraging such design patterns is crucial for building scalable and robust software systems.
In conclusion, the Decorator pattern offers an elegant solution to the challenge of extending object behavior without the need for excessive subclassing. It aligns with the principles of object-oriented design and contributes to the creation of more adaptable and reusable code. Start applying the Decorator pattern in your Java projects to unlock its potential for maximizing code reusability.