Maximizing Performance with Hibernate Cache
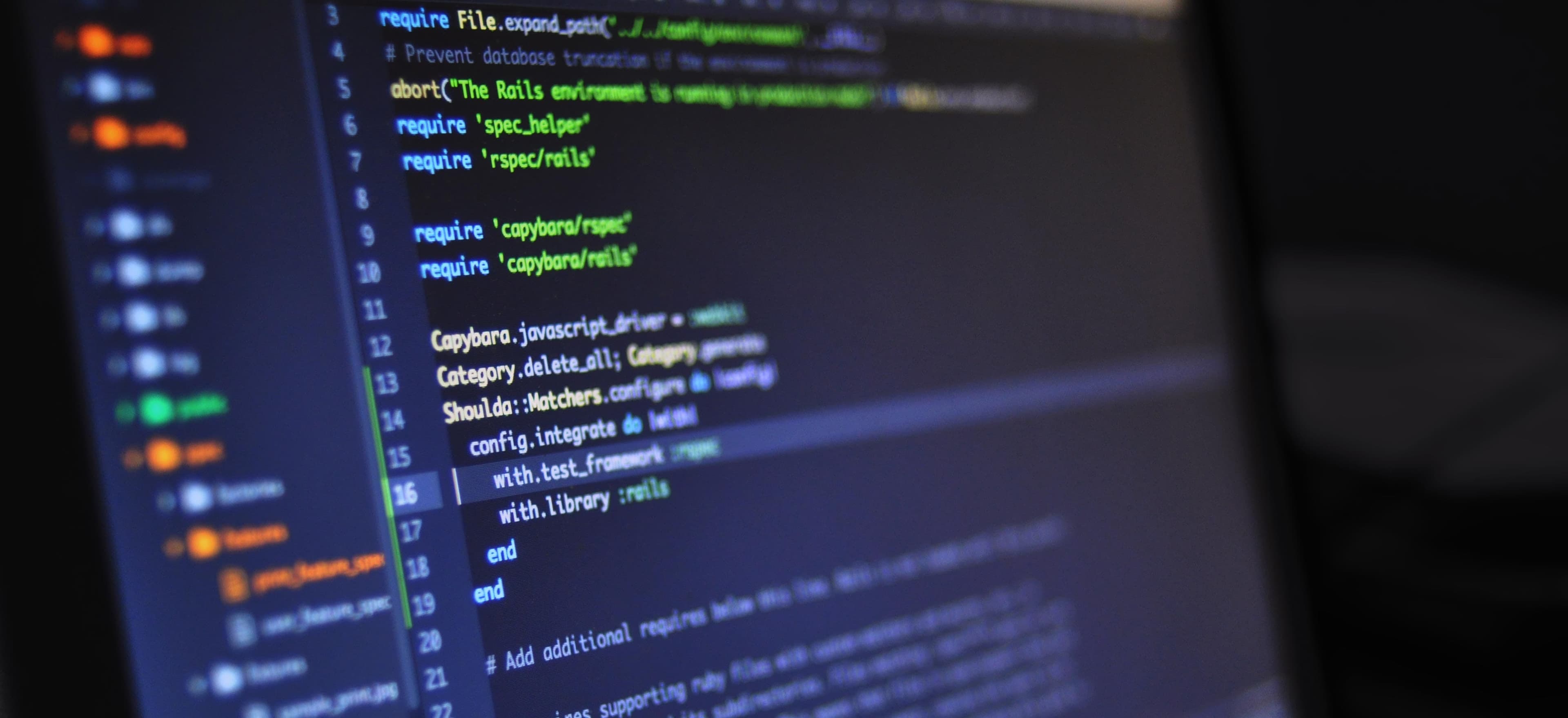
- Published on
Maximizing Performance with Hibernate Cache
When it comes to building robust and efficient Java applications, Hibernate is a popular choice for object-relational mapping (ORM). Hibernate simplifies the process of persisting Java objects to a relational database and provides a range of features to optimize performance. One such feature is the Hibernate cache, which can significantly improve the application's responsiveness and scalability. In this article, we will delve into the concept of Hibernate cache, its types, and strategies to maximize performance.
Understanding Hibernate Cache
What is Hibernate Cache?
In the context of Hibernate, a cache is a component that stores data to avoid the overhead of repeated database access. It serves as a temporary storage mechanism, holding frequently accessed data in memory. This helps in improving the overall performance by reducing the number of database calls.
Types of Hibernate Cache
-
First Level Cache:
- It is the session-level cache that is provided by default in Hibernate.
- The first level cache is associated with the Hibernate
Session
and is activated by default. - It ensures that an object loaded from the database is only loaded once within a given Hibernate
Session
.
-
Second Level Cache:
- Unlike the first level cache, the second level cache is shared across different sessions.
- It is not enabled by default and needs to be explicitly configured.
- It operates at the Session Factory level and can cache entities, collections, and queries.
Benefits of Hibernate Cache
-
Improved Performance:
- Reduces the number of SQL queries, thereby minimizing database round-trips.
- Caches frequently accessed data, leading to faster retrieval.
-
Reduced Database Load:
- Lowers the load on the database, thereby improving its overall throughput.
-
Consistency:
- Ensures data consistency by keeping track of the changes and avoiding stale data.
Drawbacks of Hibernate Cache
-
Memory Overhead:
- Caching large datasets can lead to higher memory consumption, potentially affecting the application's performance.
-
Cache Invalidation:
- Maintaining cache coherency and ensuring that the cached data remains consistent with the database can be challenging.
Strategies for Maximizing Performance
Configure Second Level Cache
To enable the second level cache, we need to configure the cache provider in the Hibernate configuration file (hibernate.cfg.xml
):
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
Here, we are using "Ehcache" as the cache provider. Other options include org.hibernate.cache.infinispan.InfinispanRegionFactory
for Infinispan and org.hibernate.cache.ehcache.SingletonEhCacheRegionFactory
for a singleton cache manager.
Entity Caching
To take advantage of the second level cache, entities need to be explicitly marked as cacheable using the @Cacheable
annotation:
@Entity
@Cacheable
public class Product {
// entity mapping
}
By marking the entity as cacheable, Hibernate will store instances of the entity in the second level cache, thus reducing the number of database accesses.
Query Caching
Hibernate also supports caching query results, which can be particularly beneficial for frequently executed or complex queries. By enabling query caching, the results of parameterized queries can be cached to avoid redundant database calls.
Annotate the query with @org.hibernate.annotations.QueryHints
to specify that the results should be cached:
Query query = session.createQuery("from Product p where p.category = :category")
.setParameter("category", category)
.setCacheable(true);
List<Product> products = query.getResultList();
By setting the setCacheable(true)
, Hibernate will cache the query and its results.
Utilize Cache Concurrency Strategies
Hibernate provides different cache concurrency strategies to control how the application interacts with the cache in a multi-threaded environment. These strategies determine how concurrent access to cached data is handled.
- Read-Only: Ideal for data that doesn't change frequently. It provides the best read performance and doesn't maintain any locking, assuming the data is read-only.
- Read-Write: Suitable for data that is frequently updated. It maintains locks to ensure data consistency between concurrent read and write operations.
- Non-Strict Read-Write: Provides a compromise between performance and consistency, allowing dirty reads but ensuring that data is eventually consistent.
- Transactional: Similar to read-write, but with additional transactional guarantees.
The concurrency strategy can be specified at the entity level or for individual collections using the @Cache
annotation.
Monitor Cache Usage
It is essential to monitor the usage and effectiveness of the cache to fine-tune the caching strategies. Tools like JMX (Java Management Extensions) provide valuable insights into cache hit rates, miss rates, and overall cache performance. By monitoring these metrics, developers can identify potential bottlenecks and optimize the cache configurations accordingly.
Final Considerations
In the realm of enterprise Java development, optimizing performance is a critical aspect that directly impacts the overall user experience. Hibernate provides a robust caching mechanism that can significantly enhance application performance by reducing database load and improving response times.
By understanding the types of caches, configuring the second level cache, and employing caching strategies such as entity caching, query caching, and concurrency settings, developers can maximize the benefits of Hibernate cache and deliver highly responsive applications.
Remember, while Hibernate cache can tremendously boost performance, it's crucial to strike a balance and judiciously apply caching to avoid potential pitfalls such as stale data and increased memory consumption. With the right approach, Hibernate cache stands as a powerful tool in the arsenal of performance optimization for Java applications.
Incorporating efficient caching mechanisms not only accelerates the application's responsiveness but also contributes to a more sustainable and scalable architecture, ultimately enriching the end-user experience.
Now, unleash the power of Hibernate cache and elevate your Java applications to the pinnacle of performance!