Functional Programming: Pure Functions in JavaScript
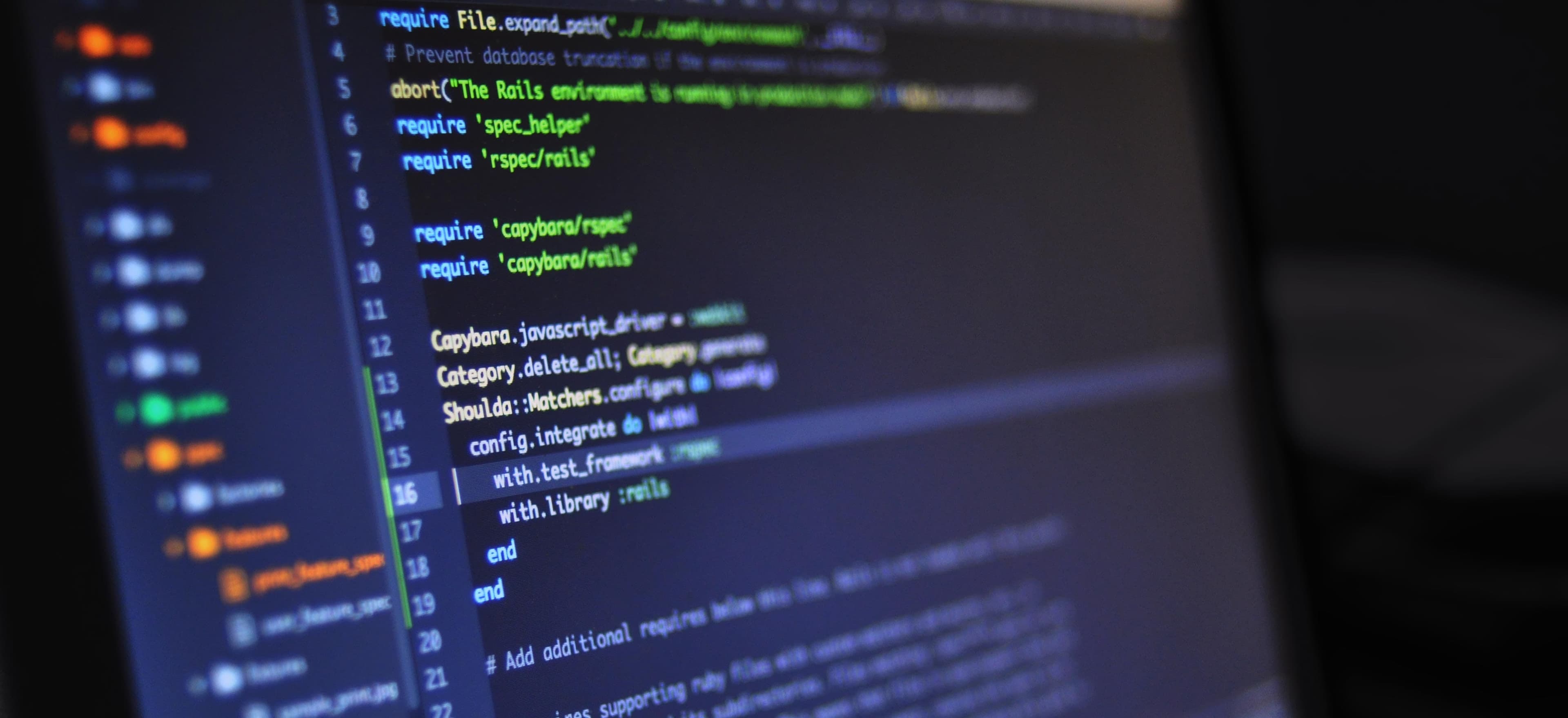
- Published on
The Power of Pure Functions in JavaScript
When it comes to functional programming, pure functions play a pivotal role. They are an indispensable concept that can significantly impact the way we write and maintain our JavaScript code. In this article, we'll delve into the world of pure functions, exploring their definition, characteristics, and the benefits they offer.
What are Pure Functions?
In the realm of programming, a pure function is a function that, given the same input, will always return the same output and does not have any side effects. This means that pure functions do not modify the state of the program outside of their scope, nor do they rely on or modify external state.
Characteristics of Pure Functions
-
Deterministic: Pure functions will consistently produce the same result for the same input, regardless of when or how many times they are invoked.
-
No Side Effects: They do not modify any external state or variables, making them predictable and easier to reason about.
Benefits of Pure Functions
Using pure functions yields several advantages, such as:
-
Easy Testing: The deterministic nature of pure functions simplifies unit testing since you can precisely predict their output based on the input.
-
Reasoning and Debugging: With no side effects to worry about, understanding and debugging code become more straightforward and less error-prone.
-
Referential Transparency: Pure functions exhibit referential transparency, enabling the substitution of function calls with their respective results without affecting the program's behavior.
Let's Code
Enough theory, let's dive into some code examples to illustrate the concept of pure functions in JavaScript.
Consider the following function that calculates the square of a number:
function square(x) {
return x * x;
}
This square
function is a pure function because it always returns the square of the input x
and does not cause any side effects.
Now, let's contrast this with a non-pure function:
let y = 10;
function addValue(x) {
return x + y;
}
In this case, the addValue
function relies on the external variable y
, making it impure as its result is influenced by a factor external to its input.
Immutability and Pure Functions
Immutability is closely tied to the concept of pure functions. In JavaScript, objects and arrays are mutable by default, meaning their values can be changed after instantiation. Pure functions discourage this mutability, advocating for the use of immutable data structures to maintain the purity of functions.
Consider the following function that modifies an array in-place:
let numbers = [1, 2, 3];
function pushNumber(num) {
numbers.push(num);
}
The pushNumber
function directly modifies the numbers
array, violating the principle of purity.
To address this, we can refactor the function to maintain immutability:
let numbers = [1, 2, 3];
function pushNumber(num, arr) {
return [...arr, num];
}
In the refactored version, a new array is created by spreading the elements of the original array and appending the new number. This ensures that the original array remains unchanged, aligning with the concept of immutability.
Embracing the Power of Functional Programming
By recognizing and leveraging the advantages of pure functions, you can embrace the paradigm of functional programming within JavaScript. This not only leads to more robust and maintainable code but also aligns with the principles of declarative and concise programming.
Integrating libraries like Ramda or Lodash can further enrich your functional programming endeavors in JavaScript, providing a plethora of utility functions designed to work seamlessly with pure functions.
Wrapping Up
In conclusion, pure functions constitute an essential building block of functional programming in JavaScript, offering a myriad of benefits such as predictability, ease of testing, and improved code maintainability. Embracing the purity of functions and immutability paves the way for writing cleaner, more concise, and reliable code, aligning with the core tenets of functional programming. As you continue to refine your JavaScript skills, remember that the journey to mastering pure functions is a powerful step toward becoming a more proficient and disciplined developer.