Mastering PWM Outputs on Raspberry Pi
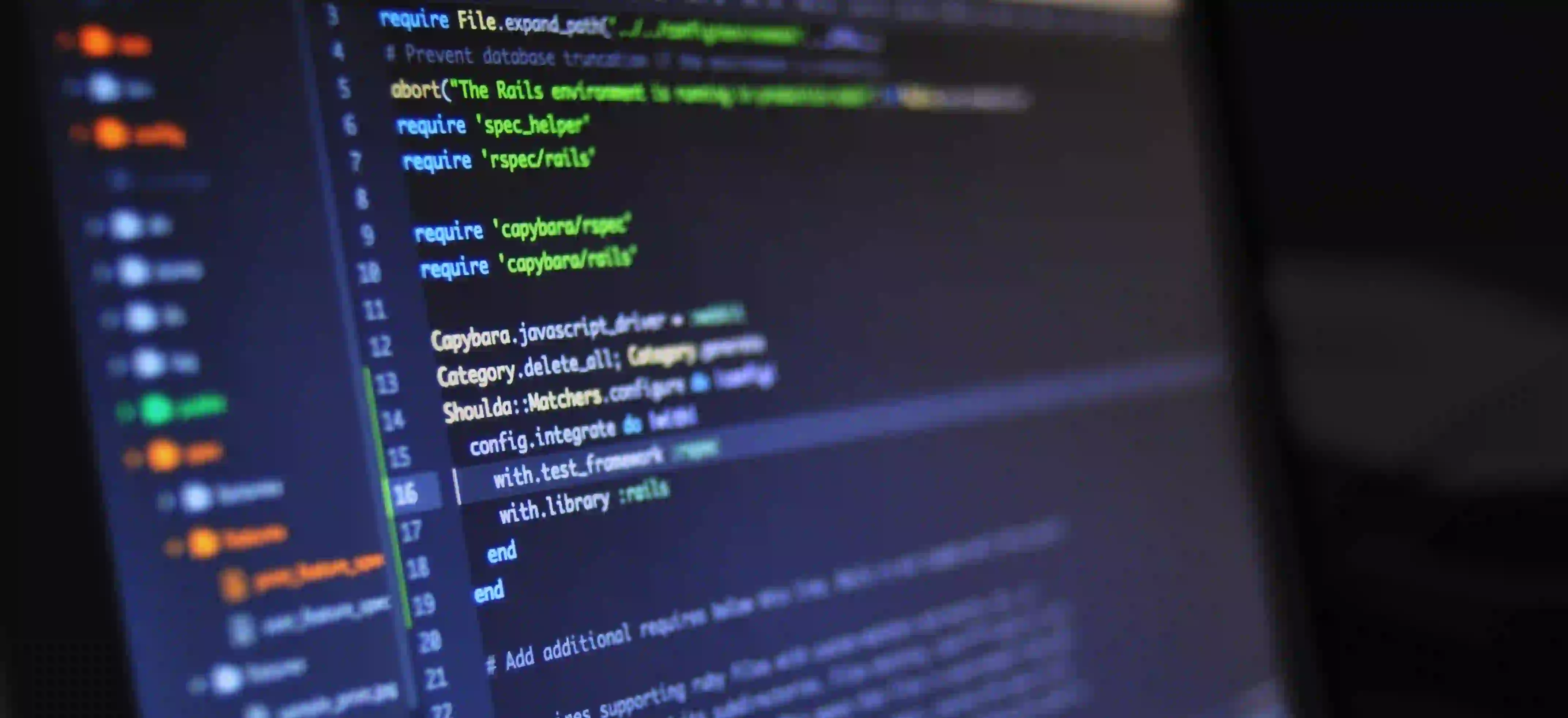
Mastering PWM Outputs on Raspberry Pi
Raspberry Pi is a powerful single-board computer that can be used for a variety of applications. One of the key features that makes Raspberry Pi suitable for controlling hardware is its ability to generate pulse-width modulation (PWM) signals. In this post, we will explore the basics of PWM and how to utilize it effectively on a Raspberry Pi using Java.
Understanding PWM
PWM is a method used to generate analog signals using digital means. Instead of outputting a constant voltage, PWM rapidly switches the output on and off, creating an average voltage that corresponds to the desired analog value. This technique is commonly used for controlling motors, LED brightness, and other analog components.
Java and PWM on Raspberry Pi
To control PWM outputs on a Raspberry Pi using Java, we can utilize the Pi4J library. Pi4J is a Java library that provides a familiar way to access the Raspberry Pi's GPIO pins, including PWM functionality. Let's dive into the steps of setting up and using PWM outputs in Java on a Raspberry Pi.
Step 1: Setting up the Pi4J Library
First, we need to set up our Raspberry Pi with the Pi4J library. This involves installing the necessary dependencies and configuring the development environment. The Pi4J website provides detailed instructions on how to get started with the library.
Step 2: Accessing GPIO Pins
Once Pi4J is set up, we can start by accessing the GPIO pins on the Raspberry Pi. We initialize the GPIO controller and provision the desired pin for PWM output using Pi4J's API.
import com.pi4j.io.gpio.GpioController;
import com.pi4j.io.gpio.GpioFactory;
import com.pi4j.io.gpio.GpioPinPwmOutput;
import com.pi4j.io.gpio.RaspiPin;
// create gpio controller
final GpioController gpio = GpioFactory.getInstance();
// provision GPIO pin #01 as a PWM output
final GpioPinPwmOutput pwm = gpio.provisionPwmOutputPin(RaspiPin.GPIO_01);
In the above code, we import the necessary Pi4J classes and initialize the GPIO controller. We then provision pin 01 as a PWM output.
Step 3: Controlling PWM Output
Once the PWM pin is provisioned, we can start controlling the PWM output. Pi4J provides methods to set the PWM range and the actual PWM value. For example, to set the PWM range to 100 (corresponding to 100% duty cycle) and set the PWM value to 50 (50% duty cycle), we can use the following code:
// set the PWM range (i.e., the maximum
// range of the PWM signal, e.g., 100)
pwm.setPwmRange(100);
// set the PWM value (e.g., a value between 0 and 100)
pwm.setPwm(50);
In this code snippet, we set the PWM range to 100 and then set the PWM value to 50, resulting in a 50% duty cycle PWM signal.
Step 4: Putting It All Together
Finally, we can put everything together to create a PWM control application. For instance, we can create a simple program that gradually increases and decreases the PWM value, effectively dimming an LED connected to the PWM pin.
// Gradually increase PWM value
for (int i = 0; i <= 100; i++) {
pwm.setPwm(i);
// Add a short delay for a smooth transition
Thread.sleep(50);
}
// Gradually decrease PWM value
for (int i = 100; i >= 0; i--) {
pwm.setPwm(i);
// Add a short delay for a smooth transition
Thread.sleep(50);
}
The above code snippet demonstrates a smooth transition of PWM output to fade an LED in and out.
Key Takeaways
In conclusion, PWM is a valuable technique for generating analog signals using digital means. With the help of the Pi4J library, controlling PWM outputs on a Raspberry Pi becomes straightforward and efficient. By following the steps outlined in this post, you can harness the power of PWM in your Java-based Raspberry Pi projects.
Utilizing PWM on a Raspberry Pi opens up a wide array of possibilities for controlling hardware components, making it an essential skill for anyone working on embedded systems or IoT projects. With the knowledge gained from this post, you are now equipped to master PWM outputs on your Raspberry Pi using Java and Pi4J.
Now that you have a comprehensive understanding of how to harness PWM outputs on a Raspberry Pi, it's time to explore the many exciting projects and applications that can benefit from this powerful capability. Happy coding!
Remember to check the official Pi4J documentation for more in-depth information and explore the additional features and functionalities it offers.