Optimizing Large Dataset Creation in Couchbase
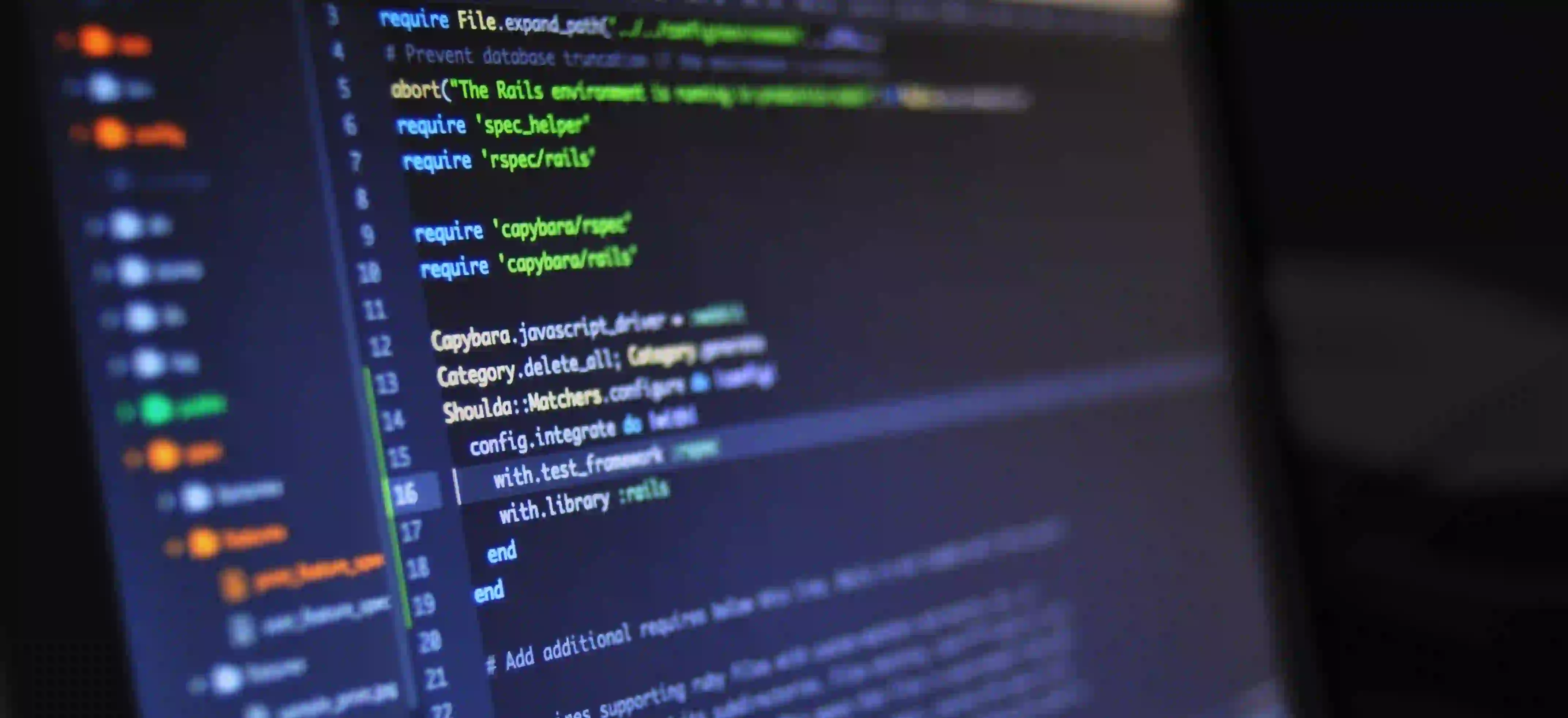
Optimizing Large Dataset Creation in Couchbase
When working with large datasets in Couchbase, it's crucial to optimize the process of creating and populating these datasets to ensure efficient performance. In this article, we'll explore various strategies and best practices for optimizing the creation of large datasets in Couchbase using Java. We'll cover topics such as batch processing, asynchronous programming, and data modeling to improve the overall efficiency and speed of dataset creation.
Understanding the Problem
Creating large datasets in Couchbase involves inserting, updating, or upserting a significant amount of data into one or more buckets. This process can become a bottleneck if not managed effectively, leading to increased latency and reduced performance. It's essential to approach dataset creation with optimization in mind to minimize the impact on the application's overall responsiveness.
Using Batch Processing
Batch processing is a technique used to divide a large dataset creation task into smaller, more manageable chunks. This approach minimizes the strain on system resources and allows for better control over the data insertion process. In Java, you can utilize libraries such as java.util.stream
to implement batch processing effectively.
List<Document> documents = // Load documents to be inserted
int batchSize = 1000;
int totalDocuments = documents.size();
for (int i = 0; i < totalDocuments; i += batchSize) {
List<Document> batch = documents.subList(i, Math.min(i + batchSize, totalDocuments));
// Insert the batch of documents into Couchbase
// Use asynchronous APIs for improved performance
}
In the above code snippet, we're breaking down the dataset creation into batches of 1000 documents to be inserted into Couchbase. This approach reduces the likelihood of overwhelming the system and allows for smoother, more efficient data population.
Leveraging Asynchronous APIs
Asynchronous programming can significantly improve the performance of large dataset creation by allowing the application to continue executing other tasks while awaiting the completion of database operations. Couchbase provides asynchronous APIs that support non-blocking data access, which is crucial when working with large datasets.
// Using asynchronous API for document insertion
bucket.defaultCollection().async().upsert(docId, document)
.whenComplete((result, ex) -> {
if (ex != null) {
// Handle insertion error
} else {
// Insertion successful
}
});
By leveraging asynchronous APIs, the application can initiate database operations and proceed with other tasks without waiting for the operations to complete. This approach enhances concurrency and overall responsiveness, especially when dealing with substantial data volumes.
Efficient Data Modeling
Data modeling in Couchbase plays a vital role in optimizing dataset creation. By organizing data according to access patterns and query requirements, you can streamline the insertion process and improve overall performance. Consider using Couchbase's document key design to distribute data evenly across the cluster and facilitate efficient retrieval.
// Example of efficient document key design
String userId = "user123";
String documentKey = "user::" + userId;
JsonObject userData = JsonObject.create()
.put("name", "John Doe")
.put("email", "john.doe@example.com");
bucket.defaultCollection().upsert(documentKey, userData);
In the above example, we're utilizing a specific document key design to store user-related data efficiently. By incorporating meaningful keys and organizing data logically, we can optimize dataset creation and retrieval, leading to improved performance.
Monitoring and Fine-Tuning
Optimizing large dataset creation in Couchbase is an iterative process. Monitoring the performance of data insertion operations and identifying potential bottlenecks are crucial steps in fine-tuning the process. Utilize Couchbase's monitoring and management tools to gather insights into resource utilization, latency, and throughput, allowing for informed adjustments and optimizations.
Final Considerations
Efficiently creating large datasets in Couchbase using Java involves a combination of batch processing, asynchronous programming, efficient data modeling, and continuous monitoring. By implementing these strategies and best practices, developers can optimize the dataset creation process, leading to improved performance and responsiveness within their applications.
Optimizing large dataset creation in Couchbase is essential for handling substantial data volumes while maintaining overall application efficiency. By incorporating batch processing, asynchronous APIs, efficient data modeling, and continuous monitoring, developers can ensure that dataset creation is both efficient and scalable within a Couchbase environment.