Troubleshooting ImageView Display in Custom ListView Adapter
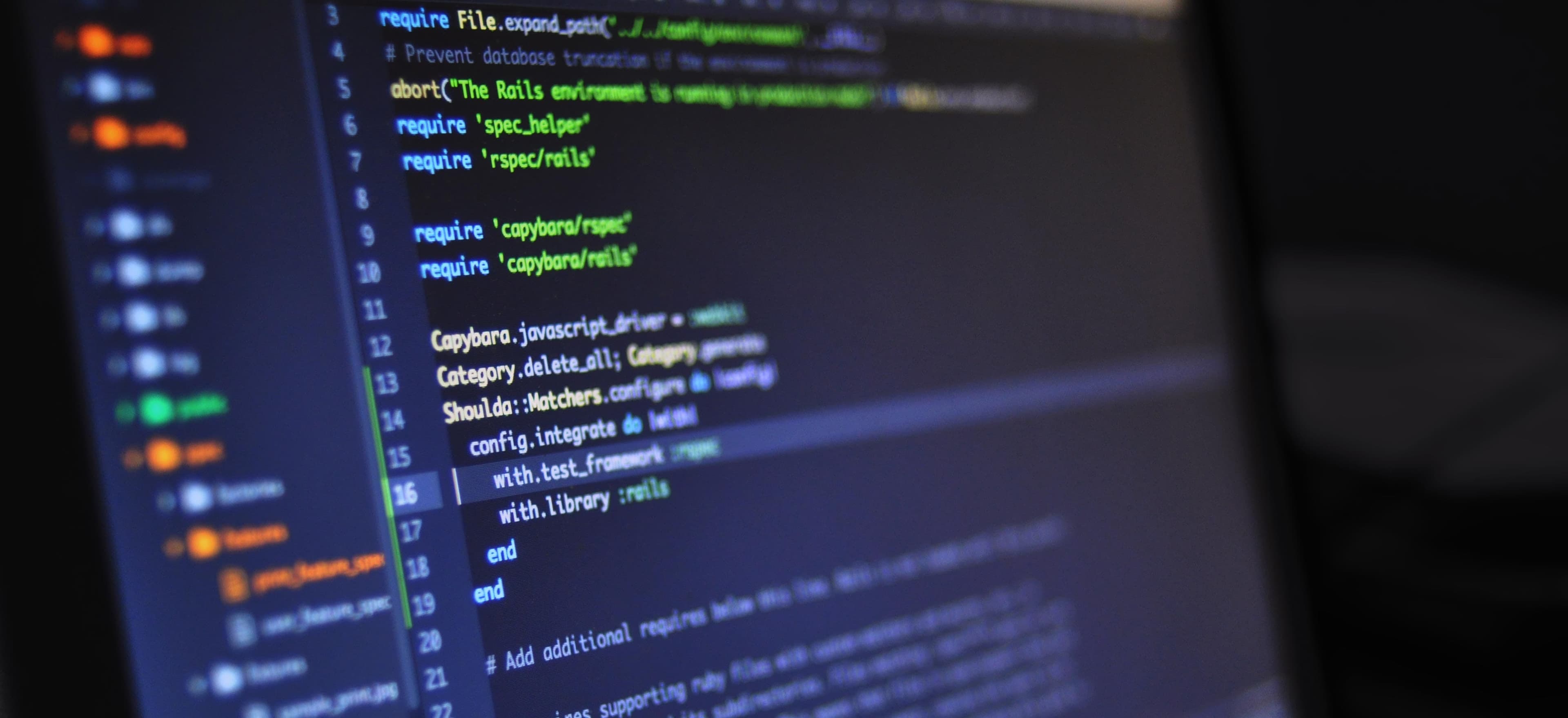
- Published on
Troubleshooting ImageView Display in Custom ListView Adapter
ListView is a fundamental component in Android development when it comes to displaying scrollable lists of items. Sometimes, while working with a custom ListView adapter, you may encounter issues with displaying ImageView correctly. In this blog post, we will discuss a common problem associated with displaying images in a custom ListView and how to troubleshoot and resolve it.
The Problem
Let's consider a scenario where you have a custom ListView with an adapter that includes ImageView to display images alongside other data. After implementing the adapter and setting it to the ListView, you notice that the images are not displaying as expected. Instead, some items may have incorrect or missing images while others display fine.
This issue often occurs due to the recycling behavior of ListView where views are reused as the user scrolls, leading to incorrect images being displayed for certain items.
The Solution
To address the problem of incorrect image display in a custom ListView adapter, we can utilize the ViewHolder pattern and implement proper handling of the ImageView within the adapter's getView method.
Below is an example of a custom ListView adapter with ImageView and how to troubleshoot and resolve the image display issue:
public class CustomAdapter extends ArrayAdapter<CustomItem> {
private Context context;
private ArrayList<CustomItem> itemList;
public CustomAdapter(Context context, ArrayList<CustomItem> itemList) {
super(context, 0, itemList);
this.context = context;
this.itemList = itemList;
}
@NonNull
@Override
public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) {
ViewHolder viewHolder;
if(convertView == null) {
convertView = LayoutInflater.from(context).inflate(R.layout.list_item, parent, false);
viewHolder = new ViewHolder();
viewHolder.itemImageView = convertView.findViewById(R.id.itemImageView);
// Initialize other views here if needed
convertView.setTag(viewHolder);
} else {
viewHolder = (ViewHolder) convertView.getTag();
}
CustomItem item = itemList.get(position);
// Load image into ImageView
Picasso.get().load(item.getImageUrl()).into(viewHolder.itemImageView);
// Bind other data to views here
// Example: viewHolder.itemTitle.setText(item.getTitle());
return convertView;
}
static class ViewHolder {
ImageView itemImageView;
// Declare other views here if needed
// Example: TextView itemTitle;
}
}
In the above code snippet, we have a CustomAdapter for a ListView with a ViewHolder to contain references to views within the list item layout. We utilize the Picasso library to load images into the ImageView efficiently.
Explanation
-
ViewHolder Pattern: By implementing the ViewHolder pattern, we ensure that views are recycled properly and prevent incorrect images from being displayed when scrolling the ListView. This pattern reduces the number of findViewById calls, improving the adapter's performance.
-
Picasso Library: We use the Picasso library to handle image loading and caching. Picasso simplifies the process of loading images from URLs and takes care of image caching, resizing, and placeholder management, thereby enhancing image rendering efficiency.
By incorporating these measures, the adapter efficiently manages the display of ImageView within the custom ListView, resolving the issue of incorrect image display.
Lessons Learned
When working with a custom ListView adapter in Android, it's crucial to understand and implement best practices to ensure smooth and correct display of ImageView and other views. The ViewHolder pattern and libraries like Picasso play a vital role in optimizing the performance and behavior of ListView components when dealing with images.
By following the provided example and incorporating these techniques into your custom ListView adapters, you can troubleshoot and resolve issues related to incorrect image display, providing a seamless and efficient user experience.
In conclusion, understanding the intricacies of ListView adapters and implementing efficient handling of ImageView can significantly impact the performance and visual presentation of your Android application.
Remember, addressing the specific needs and challenges of ListView components, such as image display, contributes to the overall user satisfaction and usability of your app.
To delve deeper into the ViewHolder pattern and ListView optimization, you can explore the official Android Developer documentation on ListView and ViewHolder.