Optimizing Performance for Customized Android Lists
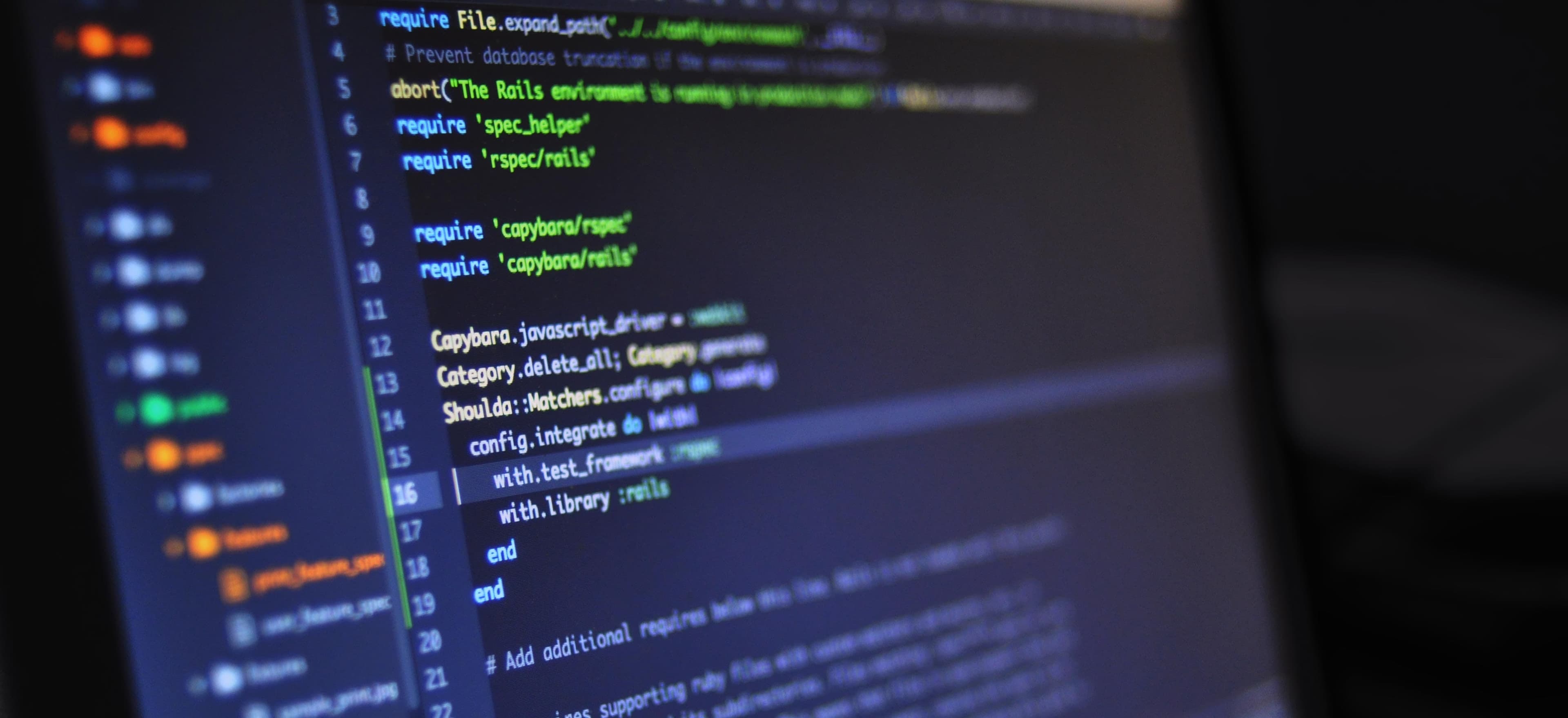
- Published on
Improving Performance for Customized Android Lists: A Java Developer's Guide
As a Java developer working on Android applications, you might come across the need to create customized lists to display complex data in a more user-friendly manner. However, as the complexity of the data and the customization of the list increases, it can have a significant impact on the app's performance. In this blog post, we will explore various techniques and best practices to optimize the performance of customized Android lists using Java.
Understanding the Problem
When dealing with customized lists in Android, the inefficiency in performance often stems from the way data is bound to the list items. Inefficient data binding can lead to UI lags, increased memory consumption, and poor scrolling performance, especially when dealing with large datasets.
Using RecyclerView for Efficient List Rendering
To address the performance issues associated with lists in Android, the RecyclerView has become the go-to solution. Unlike its predecessor, ListView, RecyclerView is designed to efficiently re-use and recycle views as the user scrolls through the list. This makes it an ideal choice for implementing customized lists with improved performance.
Implementing a Basic RecyclerView in Java
public class CustomListAdapter extends RecyclerView.Adapter<CustomListAdapter.CustomViewHolder> {
private List<DataModel> dataList;
public CustomListAdapter(List<DataModel> dataList) {
this.dataList = dataList;
}
@Override
public CustomViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.custom_list_item, parent, false);
return new CustomViewHolder(view);
}
@Override
public void onBindViewHolder(CustomViewHolder holder, int position) {
DataModel data = dataList.get(position);
holder.bind(data);
}
@Override
public int getItemCount() {
return dataList.size();
}
public static class CustomViewHolder extends RecyclerView.ViewHolder {
private TextView itemName;
public CustomViewHolder(View itemView) {
super(itemView);
itemName = itemView.findViewById(R.id.item_name);
}
public void bind(DataModel data) {
itemName.setText(data.getName());
}
}
}
In the above code snippet, we have implemented a basic RecyclerView adapter in Java. We override the onCreateViewHolder
method to inflate the custom list item layout, onBindViewHolder
to bind the data to the views, and getItemCount
to return the total number of items in the list. By following this pattern, RecyclerView efficiently recycles the views and optimizes the performance of the list.
Optimizing Data Binding with ViewHolders
In the CustomViewHolder
class above, we bind the data to the views using the bind
method. This pattern helps optimize data binding by minimizing the calls to findViewById
and updating the views only when necessary.
Efficient Use of findViewById
It's crucial to optimize the use of findViewById
by only calling it when the view is being created, such as in the ViewHolder
constructor, and storing the references for future use. This helps in avoiding redundant view lookups during data binding.
Implementing ViewHolders for Different Item Types
When dealing with a more complex list that includes different types of items, such as headers, footers, or varying layouts, the use of multiple ViewHolders can further enhance performance. This approach allows for better management of different view types within the same RecyclerView adapter, ensuring a smoother and more efficient list rendering process.
Implementing Efficient Data Handling
In addition to optimizing the view rendering process, efficient data handling is equally important for improving the performance of customized Android lists.
Working with Large Datasets
When dealing with large datasets, it's essential to implement techniques like lazy loading and pagination to fetch and display data incrementally. This prevents the app from becoming unresponsive while loading a large amount of data and also conserves memory by fetching only the required data at a given time.
Asynchronous Data Loading
Fetching data from remote sources or performing complex computations should be done asynchronously to prevent blocking the main UI thread. Java provides APIs such as AsyncTask
or libraries like RxJava for handling asynchronous operations efficiently.
Utilizing ViewHolder Patterns for Performance Optimization
While using RecyclerView, it's crucial to understand and implement the ViewHolder pattern effectively. The ViewHolder pattern, when correctly applied, can significantly improve the performance of list items by reusing views and minimizing layout inflations.
Benefits of ViewHolder Pattern
- Reduces the number of calls to
findViewById
, leading to improved data binding performance. - Recycles views efficiently, reducing memory overhead and improving scrolling smoothness.
- Helps maintain a clean and organized list item structure, facilitating easier maintenance and updates.
Lessons Learned
Creating customized lists in Android using Java can be a powerful way to enhance the user experience, but it comes with performance challenges. By leveraging the RecyclerView, optimizing data binding with ViewHolders, implementing efficient data handling, and utilizing the ViewHolder pattern, Java developers can overcome these challenges and deliver high-performance, customized lists for their Android applications.
Optimizing the performance of customized Android lists not only improves the user experience but also reflects positively on the overall quality and responsiveness of the app. By incorporating these best practices and techniques, Java developers can ensure their customized lists perform efficiently, even with large datasets and complex data structures, providing users with a seamless and responsive app experience.
In conclusion, mastering the art of optimizing performance for customized Android lists in Java is essential for delivering high-quality Android applications with a smooth and responsive user interface.
References:
- Android RecyclerView
- Optimizing Your RecyclerView
- Understanding View Recycling in Android
Remember, the key to creating a great user experience is not just in the functionality of the app, but also in the performance and responsiveness it delivers. Keep coding and optimizing!