Reading Properties with Variable Interpolation
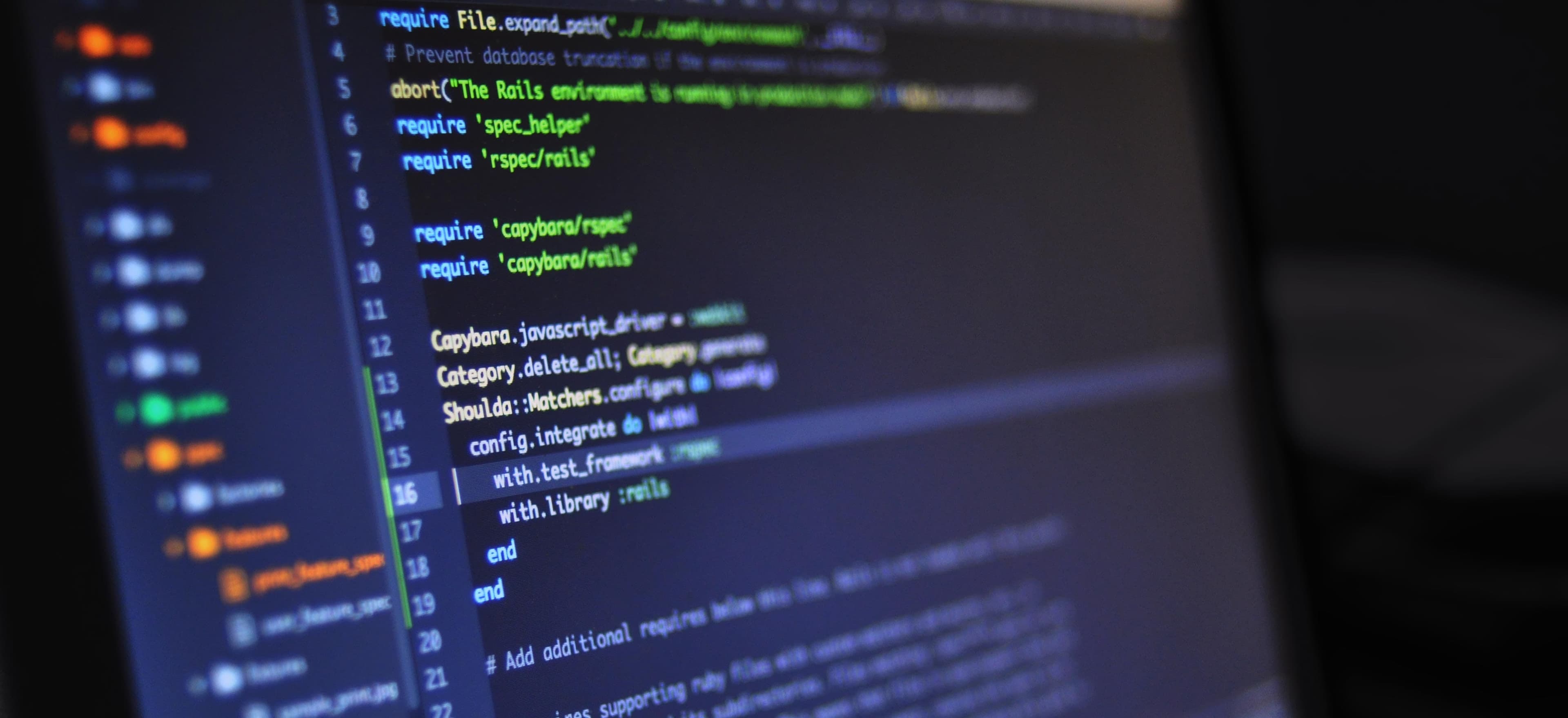
- Published on
When working with Java applications, it's essential to manage configurations effectively. One popular approach to handling configurations is by using properties files. These files store key-value pairs, allowing developers to externalize configuration from the code, which promotes easier maintainability and flexibility. In this article, we'll focus on reading properties with variable interpolation in Java.
What is Variable Interpolation?
Variable interpolation is the process of replacing placeholders in a string with actual values. In the context of properties files, variable interpolation allows us to reference and use values from the same or different keys within the properties file itself.
Let's consider a scenario where we have a properties file app.properties
with the following content:
app.name=MyApp
app.version=1.0
app.description=${app.name} version ${app.version}
Here, ${app.name}
and ${app.version}
are placeholders that we aim to interpolate with the actual values of app.name
and app.version
when reading the properties in our Java application.
Java Properties Class
In Java, the java.util.Properties
class provides a convenient way to manage and access properties. We can use this class to load properties from a file and then retrieve values using keys.
Reading Properties Without Variable Interpolation
Let's start by looking at how to read properties without variable interpolation. The Properties
class provides the load
method for loading properties from an input stream. Once loaded, we can retrieve values using the getProperty
method.
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
public class PropertiesReader {
public static void main(String[] args) {
Properties properties = new Properties();
try (InputStream input = new FileInputStream("app.properties")) {
properties.load(input);
String name = properties.getProperty("app.name");
String version = properties.getProperty("app.version");
System.out.println("Name: " + name);
System.out.println("Version: " + version);
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
In this example, we load the properties from the app.properties
file and retrieve the values using their respective keys. However, this approach does not handle variable interpolation.
Reading Properties with Variable Interpolation
To achieve variable interpolation, we can extend the java.util.Properties
class and override the getProperty
method to handle interpolation.
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Properties;
public class InterpolatedProperties extends Properties {
@Override
public String getProperty(String key) {
String value = super.getProperty(key);
if (value != null) {
StringBuilder resolved = new StringBuilder(value);
int startIdx;
while ((startIdx = resolved.indexOf("${")) != -1) {
int endIdx = resolved.indexOf("}", startIdx);
if (endIdx != -1) {
String variable = resolved.substring(startIdx + 2, endIdx);
String variableValue = super.getProperty(variable);
if (variableValue != null) {
resolved.replace(startIdx, endIdx + 1, variableValue);
}
} else {
break; // Stop if closing } is not found
}
}
value = resolved.toString();
}
return value;
}
public static void main(String[] args) {
InterpolatedProperties properties = new InterpolatedProperties();
try (InputStream input = new FileInputStream("app.properties")) {
properties.load(input);
String name = properties.getProperty("app.name");
String version = properties.getProperty("app.version");
String description = properties.getProperty("app.description");
System.out.println("Name: " + name);
System.out.println("Version: " + version);
System.out.println("Description: " + description);
} catch (IOException ex) {
ex.printStackTrace();
}
}
}
In this example, we create a subclass InterpolatedProperties
that overrides the getProperty
method to handle variable interpolation. When getProperty
is called, it resolves any placeholders within the retrieved value by replacing them with the actual values of the referenced keys.
By using this approach, we can achieve variable interpolation when reading properties in a Java application.
Final Considerations
In this article, we discussed the concept of variable interpolation in the context of reading properties in Java applications. We explored how to extend the java.util.Properties
class to incorporate variable interpolation, allowing for more dynamic and flexible property management.
By understanding and implementing variable interpolation, we can enhance the way we work with properties files, enabling more sophisticated configuration management within our Java applications.
For further reading, you may want to explore additional techniques for handling configurations, such as using libraries like Apache Commons Configuration or Spring Framework's @PropertySource
annotation. These resources can provide further insights into effectively managing configurations in Java.
Implementing variable interpolation in properties files can significantly improve the flexibility and maintainability of Java applications, and mastering this technique is a valuable skill for any Java developer.