Troubleshooting Project Jigsaw Module Dependency Issues
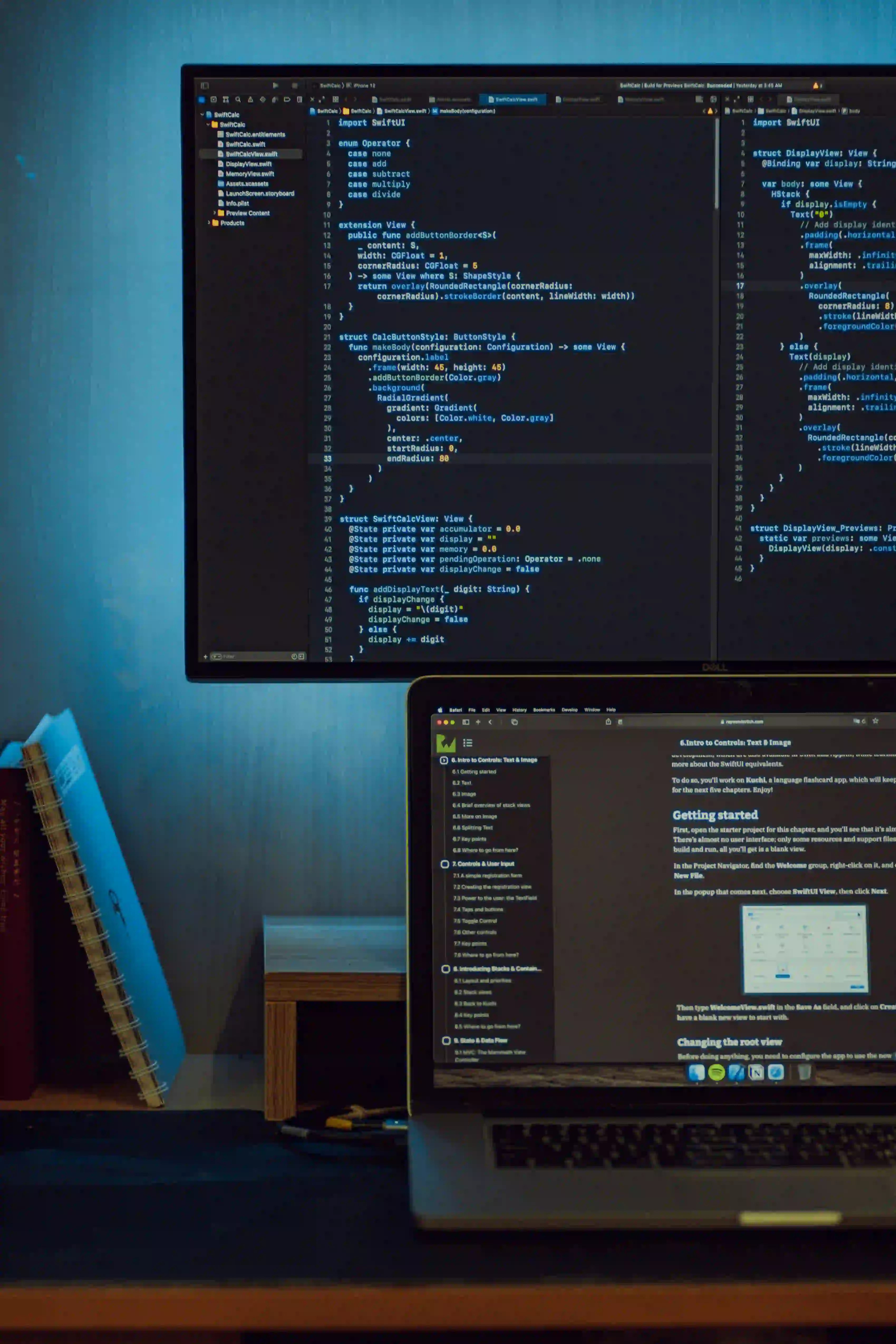
Troubleshooting Project Jigsaw Module Dependency Issues
In Java 9, Project Jigsaw introduced a modular system, enabling developers to organize code into cohesive and reusable modules. While this brings several benefits, such as improved code maintainability and encapsulation, it also comes with its own set of challenges, particularly in managing module dependencies. In this article, we will delve into common module dependency issues and how to troubleshoot them effectively.
Understanding Module Dependencies
Before diving into troubleshooting, let's revisit the basics of module dependencies in Java. In a modular application, a module can explicitly state which other modules it depends on. This is achieved through the module-info.java
file, where the requires
keyword is used to declare dependencies. For example:
module com.example.mymodule {
requires com.example.dependencymodule;
}
In this example, com.example.mymodule
depends on com.example.dependencymodule
. When the modular system is in use, it is the responsibility of the Java platform to ensure that all dependencies are resolved at both compile time and runtime.
Common Dependency Issues
Missing Module Dependencies
One of the most common issues is encountering a java.lang.module.FindException
, indicating that a required module is missing or cannot be found. This can occur due to various reasons, such as incorrect module names, missing module declaration, or misconfigured module paths.
Conflicting Module Versions
When multiple modules depend on different versions of the same module, conflicts can arise. This often leads to runtime errors, such as java.lang.module.ResolutionException
, where the JVM is unable to resolve the conflicting module versions.
Circular Dependencies
Circular dependencies occur when two or more modules depend on each other directly or indirectly. The modular system does not support circular dependencies and detecting them can be challenging since they might not be evident during compile time.
Troubleshooting Strategies
1. Verify Module Declarations
Start by ensuring that the module-info.java
files in each module accurately declare their dependencies using the requires
keyword. Verify the module names and ensure they are correctly referenced.
2. Check Module Paths
Inspect the module paths and ensure that all required modules are accessible from the specified paths. Incorrect configurations here can lead to missing module exceptions.
3. Use jdeps
Tool
The jdeps
tool provided in the JDK is a valuable resource for analyzing and troubleshooting module dependencies. By running jdeps
on a modular JAR, you can identify unresolved dependencies and circular dependencies.
4. Resolve Conflicting Versions
When encountering conflicting module versions, consider using a module that explicitly exports the conflicting package with a different name, allowing both versions to be used without conflict.
5. Update to the Latest JDK
As Project Jigsaw evolves, newer JDK releases often contain bug fixes and improvements related to module resolution and dependencies. Updating to the latest JDK version can help to resolve certain dependency issues.
Closing the Chapter
Troubleshooting module dependency issues in Project Jigsaw requires a thorough understanding of the modular system and the available tools for analysis. By carefully examining module declarations, paths, and employing tools like jdeps
, many common issues can be resolved effectively. Additionally, staying updated with the latest JDK releases can ensure access to fixes and enhancements related to module dependencies. With these strategies and best practices in place, developers can navigate and resolve module dependency issues with confidence.
As you navigate the world of Java module dependencies, you may find it beneficial to read more about the latest developments in Project Jigsaw. Additionally, exploring real-world examples of module dependencies in large-scale Java applications can provide deeper insights into best practices.