Optimizing Java Application Performance: Troubleshooting Memory Leaks
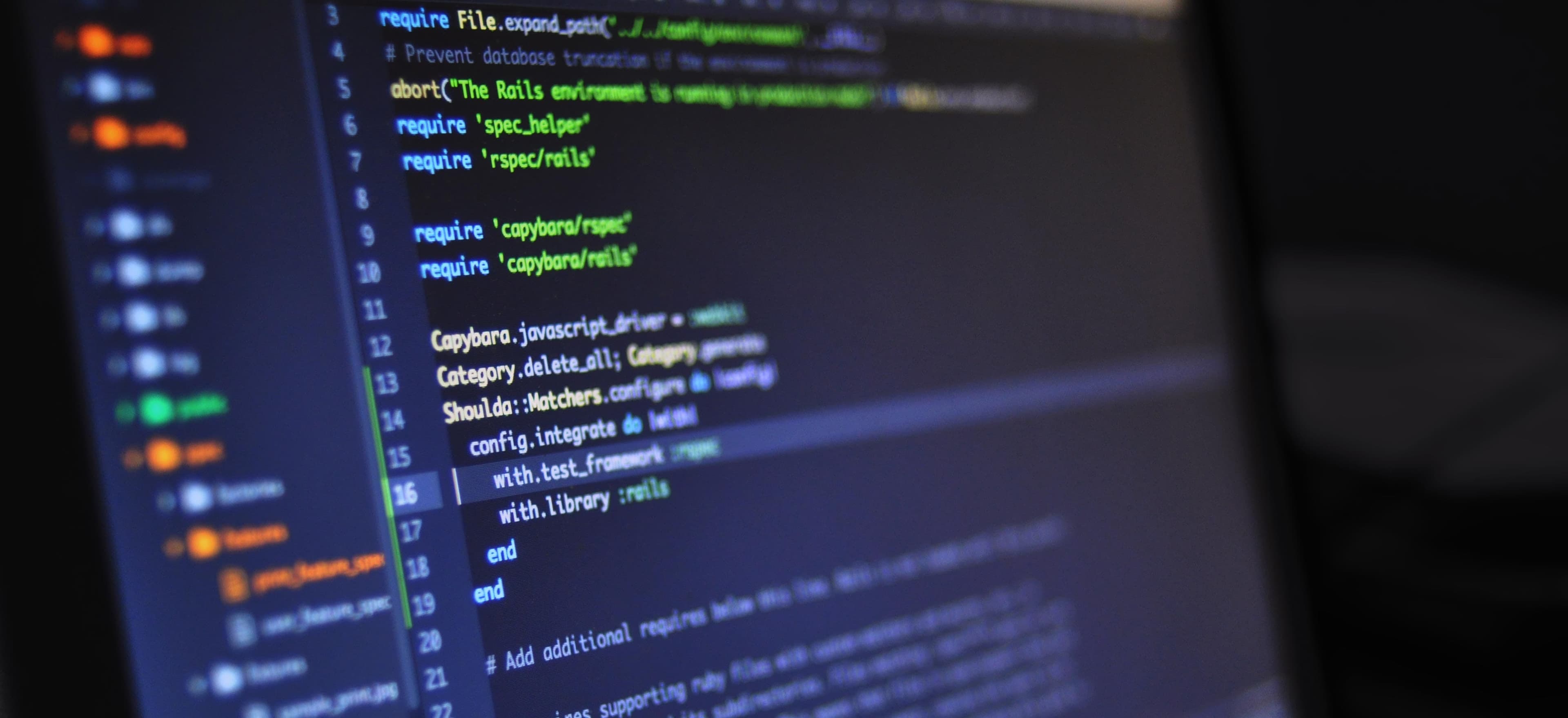
- Published on
Optimizing Java Application Performance: Troubleshooting Memory Leaks
As a Java developer, ensuring that your application runs smoothly and efficiently is essential. One common issue that plagues many Java applications is memory leaks. Memory leaks can lead to increased memory consumption, degraded performance, and even application crashes. In this post, we will dive into the world of troubleshooting memory leaks in Java applications and explore some best practices for optimizing memory usage.
Understanding Memory Leaks
Before we delve into troubleshooting memory leaks, it's crucial to understand what a memory leak is. In Java, a memory leak occurs when objects that are no longer needed are not garbage collected, leading to a gradual increase in memory usage over time. Memory leaks can be caused by various factors, such as holding onto references longer than necessary, improper use of caches, or misuse of third-party libraries.
Identifying Memory Leaks
The first step in troubleshooting memory leaks is to identify the areas in your code where memory leaks may be occurring. Tools such as Java VisualVM, YourKit, or JConsole can be immensely helpful in this process. These tools provide insights into memory usage, object creation, and garbage collection behavior, allowing you to pinpoint potential memory leak hotspots in your application.
Here's an example of how you can use JConsole to monitor memory usage and identify potential memory leaks:
public class MemoryLeakExample {
public static void main(String[] args) {
// Perform operations and monitor memory usage with JConsole
}
}
By monitoring the memory usage of your application using JConsole, you can identify any abnormal memory consumption patterns that may indicate the presence of memory leaks.
Implementing Best Practices to Avoid Memory Leaks
Preventing memory leaks is always better than dealing with them after they occur. By following some best practices, you can significantly reduce the likelihood of memory leaks in your Java applications.
Proper Resource Management
One common cause of memory leaks is improper resource management, such as not closing database connections or file handles after their use. Using try-with-resources or ensuring explicit resource closure in finally blocks can help mitigate this issue.
try (Connection connection = DriverManager.getConnection(url, user, password)) {
// Use the connection
} catch (SQLException e) {
// Handle exception
} // Connection is automatically closed at the end of the try block
The above code snippet demonstrates the use of try-with-resources to properly close a database connection, thereby preventing potential memory leaks associated with unclosed connections.
Avoiding Unnecessary Object Retention
Retaining references to objects longer than necessary can also lead to memory leaks. Carefully managing object lifecycles and avoiding unnecessary retention of objects can help mitigate this issue.
public class MemoryLeakExample {
private static List<Object> objectList = new ArrayList<>();
public void addObject(Object obj) {
objectList.add(obj);
}
public void removeObject(Object obj) {
objectList.remove(obj);
}
}
In the above example, if removeObject
is not called after removing an object from the list, it can lead to a memory leak as the object will still be referenced in the objectList
.
Memory Profiling and Analysis
Using tools like VisualVM or YourKit for memory profiling and analysis can provide valuable insights into object retention, memory usage, and potential memory leak culprits in your application. By analyzing memory snapshots and identifying long-lived objects, you can take proactive measures to address potential memory leaks.
Mitigating Memory Leaks with Proper Garbage Collection
Proper garbage collection is essential for mitigating memory leaks in Java applications. By understanding garbage collection behavior and tuning the garbage collection settings, you can optimize memory usage and reduce the risk of memory leaks.
Understanding Garbage Collection Algorithms
Java offers various garbage collection algorithms, such as the Parallel GC, Concurrent Mark-Sweep (CMS) GC), and Garbage-First (G1) GC. Each algorithm has its strengths and weaknesses, and understanding them can help in selecting the most suitable garbage collection algorithm for your application.
Tuning Garbage Collection Settings
By tuning garbage collection settings, such as heap size, garbage collection algorithm, and collection intervals, you can optimize memory management and minimize the risk of memory leaks.
java -Xmx2G -Xms512M -XX:+UseG1GC -XX:MaxGCPauseMillis=200
In the above command, we set the initial heap size to 512MB and the maximum heap size to 2GB, and we use the G1 garbage collection algorithm with a maximum pause time of 200 milliseconds. This configuration can help in mitigating memory leaks by efficiently managing memory and garbage collection.
Closing Remarks
Memory leaks can have detrimental effects on the performance and stability of Java applications. By understanding the causes of memory leaks, identifying potential leak sources, and implementing best practices for memory management, you can optimize your Java applications to mitigate memory leaks and improve overall performance.
Remember, preventing memory leaks through proper resource management, object lifecycle management, and proactive memory profiling is crucial. Additionally, tuning garbage collection settings and understanding garbage collection algorithms can further enhance memory management in your Java applications.
In conclusion, proactive monitoring, sound coding practices, and understanding of memory management in Java are key to optimizing application performance and preventing memory leaks.
By implementing the best practices discussed in this post, you can enhance the reliability and efficiency of your Java applications while ensuring optimal memory usage and performance.
Optimizing Java application performance through effective memory leak troubleshooting is an ongoing process, but the rewards of a stable and efficient application make it well worth the effort.