Managing Concurrent Access with Intrinsic Locks
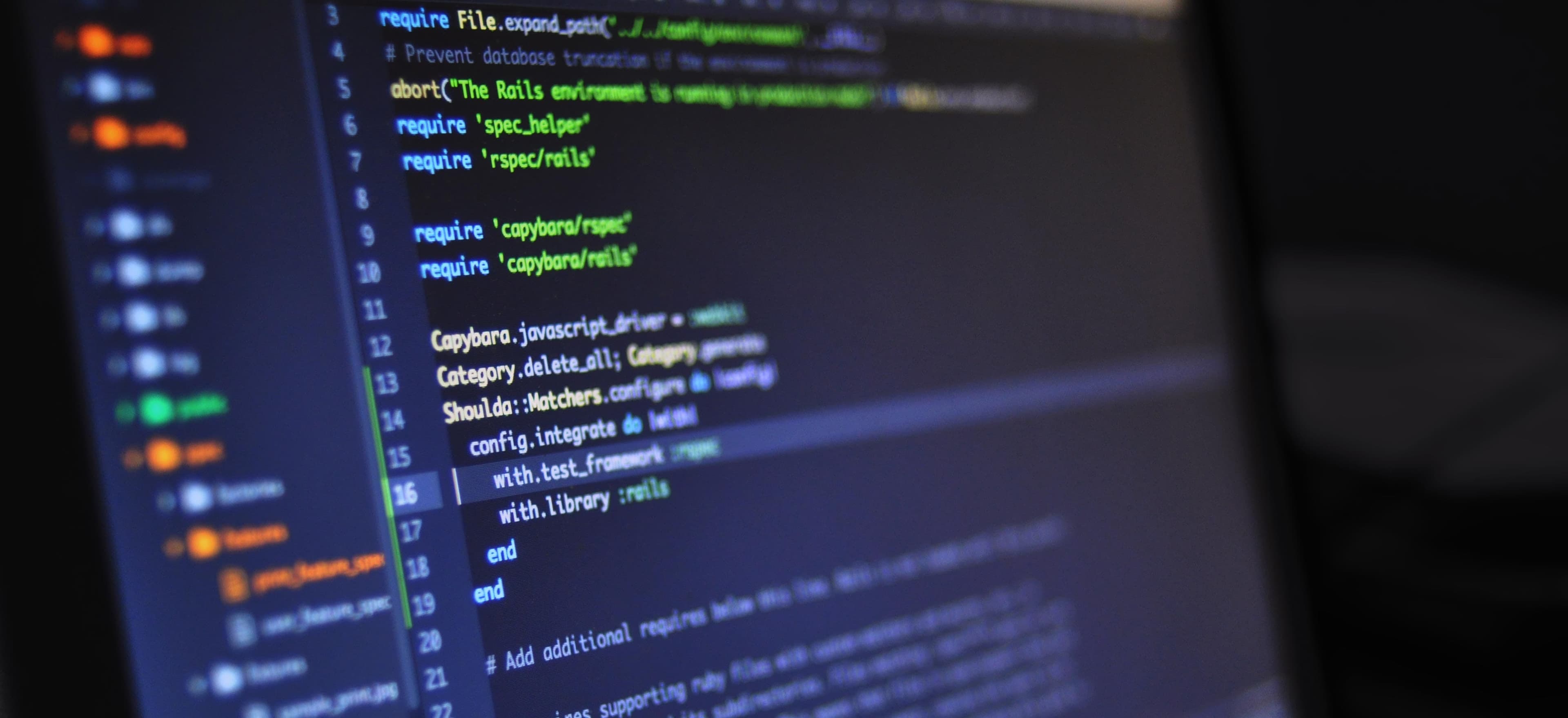
- Published on
Understanding Intrinsic Locks in Java
In Java, when we talk about intrinsic locks, we are referring to the built-in locking mechanism provided by the language. The concept of intrinsic locks is crucial when it comes to managing concurrent access to shared resources in a multi-threaded environment.
What are Intrinsic Locks?
In Java, every object has an intrinsic lock associated with it. This lock is also known as a monitor lock. When a thread wants to access a synchronized block of code on an object, it must first acquire the intrinsic lock of that object. This ensures that only one thread can execute the synchronized code block at a time, preventing concurrent access and potential data corruption.
Example of Acquiring an Intrinsic Lock
Let's take a look at an example of how a thread acquires the intrinsic lock of an object:
public class IntrinsicLockExample {
private final Object lock = new Object();
public void performSynchronizedAction() {
synchronized (lock) {
// Synchronized code block
// Only one thread can execute this block at a time
}
}
}
In the above example, the synchronized
keyword is used to acquire the intrinsic lock of the lock
object. This ensures that only one thread can execute the performSynchronizedAction
method at a time.
Why Use Intrinsic Locks?
In a multi-threaded environment, it's crucial to ensure that shared resources are accessed in a thread-safe manner. Intrinsic locks provide a simple and effective way to achieve this thread safety by allowing only one thread to access the synchronized code block at a time.
Pitfalls and Considerations
While intrinsic locks are powerful, it's essential to use them judiciously to avoid potential pitfalls such as deadlocks and thread contention. Deadlocks can occur when threads end up waiting indefinitely for each other to release locks, leading to a stalemate situation. Thread contention can arise when multiple threads are vying for the same lock, potentially impacting performance.
Best Practices for Using Intrinsic Locks
-
Keep Synchronized Blocks Minimal: To minimize the potential for deadlocks and contention, it's best to keep synchronized blocks as minimal as possible. Only critical sections of code that involve shared resources should be synchronized.
-
Use Reentrant Locks for Greater Flexibility: In situations where finer-grained control is needed, consider using
ReentrantLock
from thejava.util.concurrent
package. It provides additional features such as fairness and the ability to back out of an attempted lock acquisition.
Demonstrating Intrinsic Locks in Action
Let's walk through a simple example to demonstrate the use of intrinsic locks in managing concurrent access.
Suppose we have a BankAccount
class that represents a simple bank account:
public class BankAccount {
private double balance;
public synchronized void deposit(double amount) {
balance += amount;
}
public synchronized void withdraw(double amount) {
balance -= amount;
}
public synchronized double getBalance() {
return balance;
}
}
In this example, each of the deposit
, withdraw
, and getBalance
methods is synchronized. This means that only one thread can execute any of these methods at a time, ensuring the integrity of the balance
data.
Final Considerations
Intrinsic locks in Java provide a fundamental mechanism for managing concurrent access and ensuring thread safety when working with shared resources. Understanding how to use intrinsic locks effectively, while being mindful of potential pitfalls, is essential for writing robust multi-threaded applications.
By leveraging intrinsic locks and following best practices, we can write Java code that is not only thread-safe but also performs efficiently in a concurrent environment.
To delve deeper into the topic of concurrency and synchronization in Java, consider exploring the official Java documentation on concurrency.
In summary, intrinsic locks are a foundational concept in Java concurrency, and mastering their usage is key to writing reliable and scalable multi-threaded applications.
Let’s embrace intrinsic locks for better concurrency control in our Java applications!