Disabling Hibernate Autocommit to Force Manual Command Execution
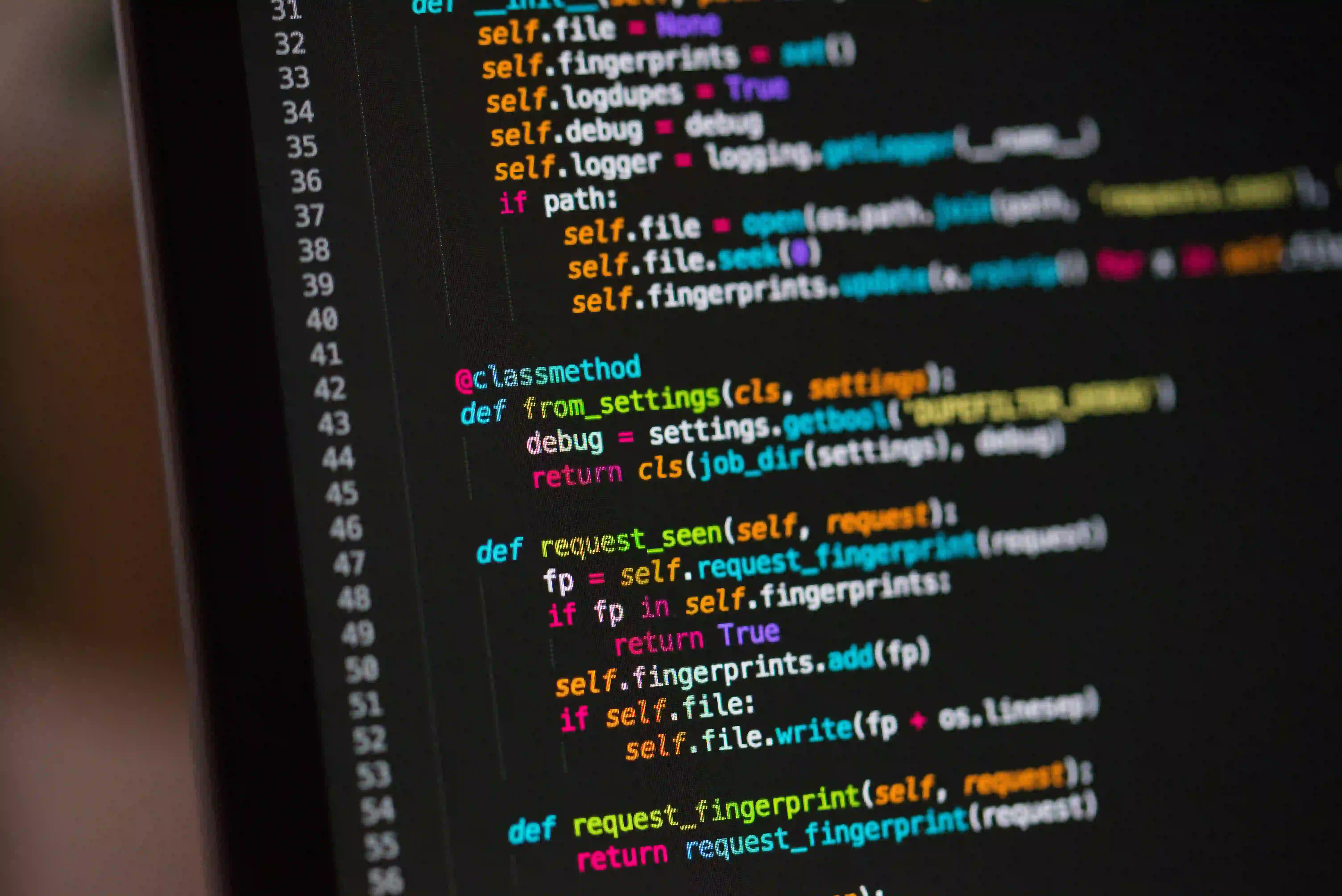
Disabling Hibernate Autocommit to Force Manual Command Execution
In certain scenarios, it becomes necessary to disable the default autocommit behavior of Hibernate to manually execute commands. This approach offers greater control and flexibility when dealing with database transactions. In this article, we will explore how to disable Hibernate autocommit and manage manual command execution effectively.
Understanding Autocommit in Hibernate
By default, Hibernate operates in autocommit mode, wherein every SQL statement is immediately committed to the database. This behavior is suitable for many use cases, as it simplifies transaction management. However, in some cases, developers may prefer to exert explicit control over the transaction boundaries and commit statements manually.
Disabling Autocommit
To disable autocommit in Hibernate, we can utilize the Transaction
interface and its associated methods. Let's take a look at a practical example of how to accomplish this.
Session session = sessionFactory.openSession();
Transaction transaction = session.beginTransaction();
try {
// Perform database operations within the transaction
// ...
// Commit the transaction
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
} finally {
session.close();
}
In the above code snippet, we open a session using the SessionFactory
and begin a transaction. We then execute the necessary database operations within the try block. Upon successful execution, we commit the transaction. In case of any exceptions, we roll back the transaction. Finally, we close the session.
By following this pattern, developers can handpick the statements that should be part of the same transaction and exercise explicit control over committing or rolling back these statements.
Why Disable Autocommit?
Disabling autocommit in Hibernate serves various purposes:
-
Batch Processing: When dealing with large datasets, switching off autocommit allows developers to group multiple database operations into a single transaction, thus enhancing performance and minimizing database round trips.
-
Complex Business Logic: Certain business processes demand intricate, multi-step database operations that need to be treated as a single unit of work. Disabling autocommit enables explicit control over transaction boundaries, ensuring data integrity.
-
Error Handling: Manual transaction management permits custom error handling and recovery strategies, ensuring that the database is left in a consistent state in case of failures.
-
Custom Locking: Disabling autocommit facilitates the explicit application of locks on specific resources when necessary, offering enhanced concurrency control.
Considerations and Best Practices
While disabling autocommit provides benefits, it also introduces additional responsibilities and considerations for developers.
-
Resource Management: It is essential to close database resources (such as sessions) promptly to prevent resource leaks and potential performance degradation. Utilizing try-with-resources or finally blocks is a recommended practice.
-
Concurrency: Manual transaction management requires careful attention to concurrency issues. Developers should assess potential contention and isolation levels to ensure data consistency and minimize deadlocks.
-
Performance Impact: Explicit transaction handling may impact performance, particularly when handling a large number of concurrent transactions. Thorough performance testing and optimization are crucial in such scenarios.
Wrapping Up
In conclusion, disabling autocommit in Hibernate empowers developers with greater control and flexibility over database transactions. By leveraging manual transaction management, developers can optimize performance, enforce data integrity, and implement custom error handling strategies. However, it is important to weigh the benefits against the additional complexity and responsibilities introduced by this approach.
For further insights into Hibernate and transaction management, refer to the official Hibernate documentation.
Do share your thoughts and experiences with manual transaction management in Hibernate!