Optimizing Memory Allocation in Java Chronicle Bytes
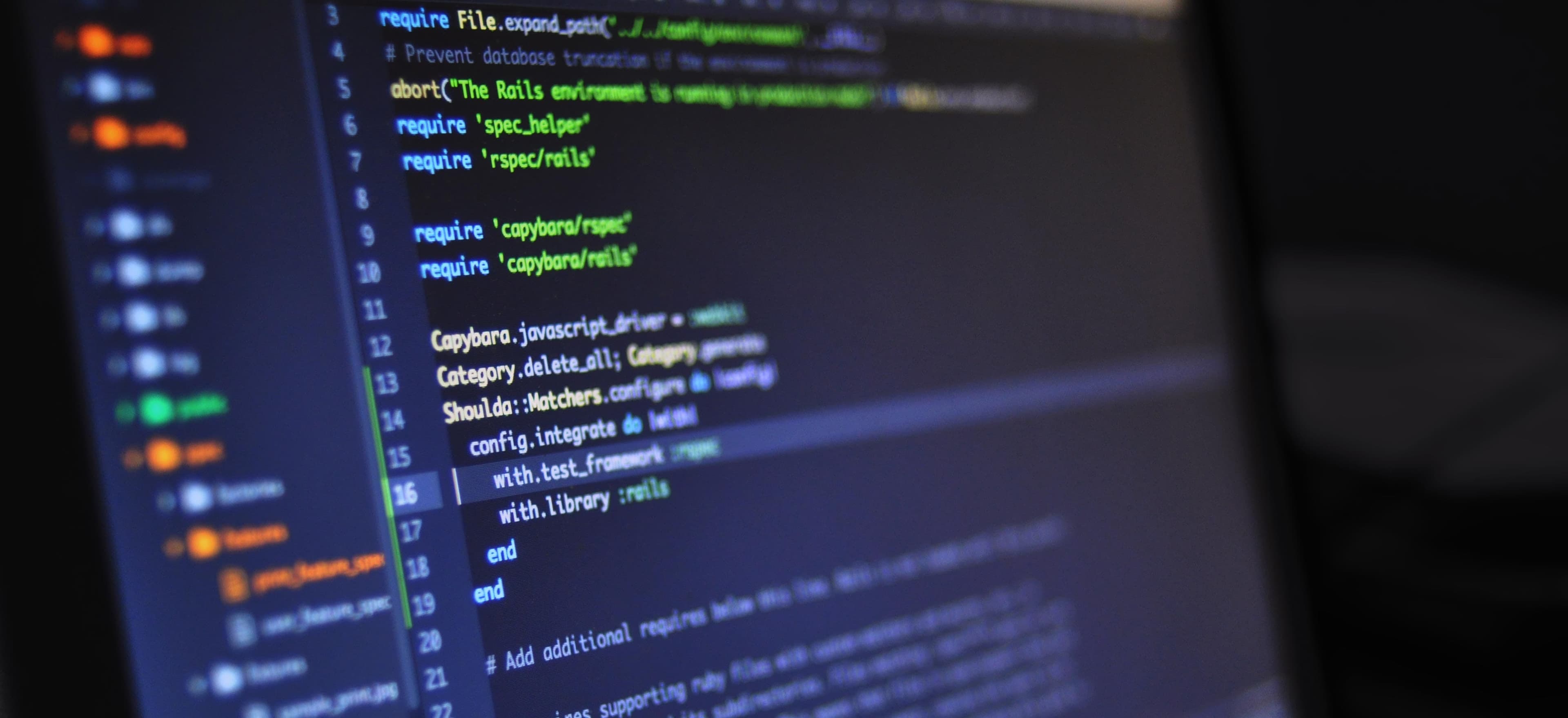
- Published on
Optimizing Memory Allocation in Java: A Deep Dive into Chronicle Bytes
When it comes to building high-performance, low-latency applications in Java, optimizing memory allocation is crucial. In this article, we'll take a deep dive into how Chronicle Bytes can be used to optimize memory allocation in Java and improve application performance.
What is Chronicle Bytes?
Chronicle Bytes is a library that provides low-level access to binary data in Java. It is designed to efficiently manage memory allocation and access binary data with minimal overhead. Chronicle Bytes is part of the Chronicle-Engine library, which is known for its high-performance and low-latency capabilities.
Efficient Memory Allocation with Chronicle Bytes
Optimizing memory allocation is essential for achieving high performance in Java applications. Excessive memory allocation can lead to increased garbage collection overhead and reduced overall application performance. Chronicle Bytes addresses this issue by providing efficient memory management for binary data.
Let's dive into some code examples to illustrate how Chronicle Bytes can be used to optimize memory allocation in Java.
// Allocate a new Chronicle Bytes object with a specific capacity
int capacity = 1024; // specify the capacity in bytes
Bytes<ByteBuffer> bytes = Bytes.elasticByteBuffer(capacity);
In this code snippet, we use Bytes.elasticByteBuffer(capacity)
to allocate a new Chronicle Bytes object with a specific capacity. This allows us to manage memory allocation more efficiently by pre-allocating the required capacity for our binary data.
// Write data to the Chronicle Bytes object
bytes.writeUTFΔ("Hello, Chronicle Bytes!");
Next, we use the writeUTFΔ
method to write UTF-encoded data to the Chronicle Bytes object. By using Chronicle Bytes' efficient memory allocation and data access mechanisms, we can minimize unnecessary memory overhead and improve overall application performance.
Minimizing Memory Overhead with Direct Memory Allocation
In Java, direct memory allocation can be used to minimize memory overhead and improve data access performance. Chronicle Bytes leverages direct memory allocation to efficiently manage binary data, resulting in lower memory overhead and improved application performance.
// Allocate a new Chronicle Bytes object with direct memory allocation
int capacity = 1024; // specify the capacity in bytes
Bytes<ByteBuffer> bytes = Bytes.elasticByteBuffer(capacity, false);
In this code snippet, we allocate a new Chronicle Bytes object with direct memory allocation by passing false
as the second argument to elasticByteBuffer
. This instructs Chronicle Bytes to use direct memory allocation, minimizing the memory overhead associated with the allocated binary data.
Improving Data Access Performance
Efficient memory allocation is just one part of the equation. Data access performance is equally important when it comes to building high-performance applications. Chronicle Bytes provides efficient data access mechanisms that minimize overhead and improve data access performance.
// Read data from the Chronicle Bytes object
String message = bytes.readUTFΔ();
System.out.println(message);
In this code snippet, we use the readUTFΔ
method to efficiently read UTF-encoded data from the Chronicle Bytes object. Chronicle Bytes' optimized data access mechanisms ensure that data is accessed with minimal overhead, resulting in improved overall application performance.
My Closing Thoughts on the Matter
Optimizing memory allocation and data access performance is essential for building high-performance, low-latency applications in Java. Chronicle Bytes provides efficient memory allocation and data access mechanisms that help minimize memory overhead and improve overall application performance.
By leveraging Chronicle Bytes, developers can build high-performance applications that deliver low-latency, efficient data processing. If you're interested in learning more about Chronicle Bytes and its capabilities, check out the official documentation and start optimizing your Java applications for peak performance.
In conclusion, Chronicle Bytes is a powerful tool for optimizing memory allocation in Java, and its efficient data access mechanisms make it a valuable asset for building high-performance applications.