Efficient File Transfer with Maven and Amazon S3
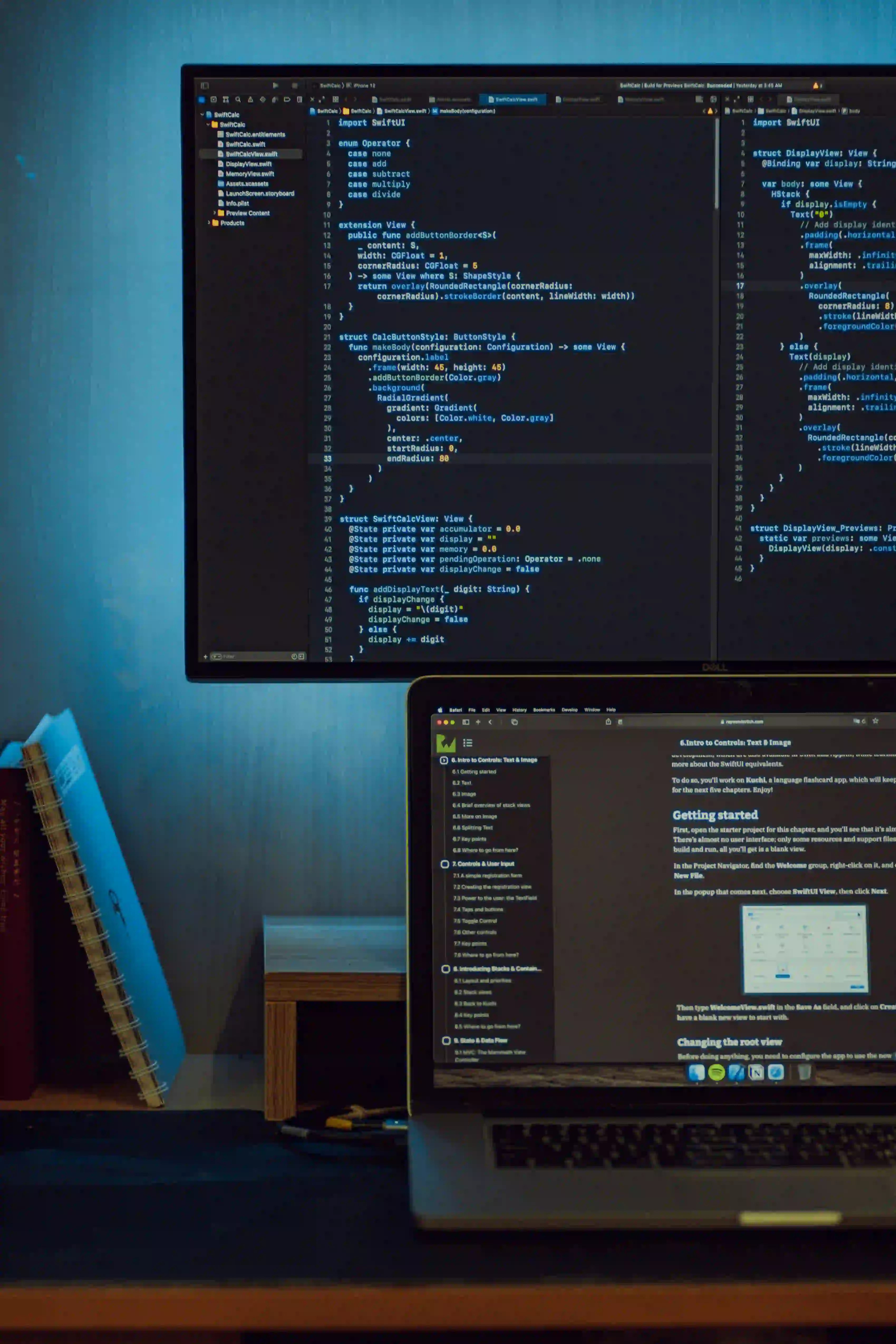
Efficient File Transfer with Maven and Amazon S3
In the world of Java development, efficiently transferring files is a common task. Whether it's deploying artifacts to a remote repository, sharing files within a team, or distributing resources to a production environment, having a robust and reliable file transfer process is crucial.
In this blog post, we'll explore how Maven, a widely used build tool for Java projects, can be leveraged for efficient file transfer using Amazon S3, a popular cloud storage service. We'll delve into the configuration, implementation, and best practices for seamlessly integrating Maven with Amazon S3 for smooth file transfers.
Setting Up Amazon S3
Before we dive into the Maven configuration, let's ensure that you have an active Amazon S3 account and an S3 bucket created. If you haven't done so, head over to the Amazon S3 homepage to create an account and set up your bucket. Make sure to take note of your Access Key and Secret Key, as we'll need them for authentication in the Maven setup.
Maven Configuration
To start using Maven for file transfers to and from Amazon S3, you'll need to include the aws-maven
plugin in your project. Open your project's pom.xml
file and add the following plugin configuration within the <build><plugins>
section:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-deploy-plugin</artifactId>
<version>3.0.0-M1</version>
<configuration>
<altDeploymentRepository>internal.repo::default::s3://your-bucket-name</altDeploymentRepository>
</configuration>
</plugin>
In this configuration, we're leveraging the maven-deploy-plugin
to upload artifacts to our specified Amazon S3 bucket. The <altDeploymentRepository>
element is customized to point to our S3 bucket URL, ensuring that artifacts are deployed to the correct location.
With this setup, Maven will seamlessly transfer artifacts to the specified S3 bucket during the deployment phase. This streamlines the process by eliminating the need for manual uploads and ensuring consistency in artifact storage.
Implementing File Transfer
Now that we have the Maven configuration in place, let's walk through the implementation of file transfer using the configured setup. We'll use a simple Java application to demonstrate the process of uploading a file to our Amazon S3 bucket.
First, ensure that you have the AWS SDK for Java included as a dependency in your project. Add the following dependency to your pom.xml
file within the <dependencies>
section:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>s3</artifactId>
<version>2.17.28</version>
</dependency>
With the dependency added, you can now write a Java class to perform the file upload. Below is an example of how to upload a file to Amazon S3 using the AWS SDK for Java:
import software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider;
import software.amazon.awssdk.regions.Region;
import software.amazon.awssdk.services.s3.S3Client;
import software.amazon.awssdk.services.s3.model.PutObjectRequest;
import java.io.File;
public class S3FileUploader {
public static void main(String[] args) {
String bucketName = "your-bucket-name";
String key = "example-file.txt";
String filePath = "path/to/local/file/example-file.txt";
S3Client s3Client = S3Client.builder()
.region(Region.US_EAST_1)
.credentialsProvider(DefaultCredentialsProvider.create())
.build();
s3Client.putObject(PutObjectRequest.builder()
.bucket(bucketName)
.key(key)
.build(),
new File(filePath));
}
}
In this Java class, we're utilizing the AWS SDK for Java to create an S3 client, specifying the region, credentials, and the file to upload. The putObject
method is then called to initiate the file transfer to the specified S3 bucket.
This implementation showcases how seamlessly Maven-integrated file transfer with Amazon S3 can be leveraged within a Java application, streamlining the process of uploading files to cloud storage.
Best Practices for Efficient File Transfer
When working with Maven and Amazon S3 for file transfer, it's essential to adhere to best practices to ensure efficient and reliable transfers. Here are some key considerations to keep in mind:
-
Optimize Artifact Size: When deploying artifacts to Amazon S3, aim to optimize their size to reduce transfer times and storage costs. Consider techniques such as compression and minimizing unnecessary content.
-
Secure File Transfers: Always prioritize the security of file transfers by utilizing secure access credentials, such as IAM roles or access keys. Additionally, consider enabling encryption for files transferred to S3.
-
Versioning and Lifecycle Policies: Leverage versioning and lifecycle policies within Amazon S3 to manage artifact versions and automate the transition of objects to different storage tiers.
-
Monitoring and Logging: Implement comprehensive monitoring and logging for file transfers to track transfer status, detect issues, and gather insights for optimization.
By adhering to these best practices, you can enhance the efficiency and reliability of file transfers when utilizing Maven and Amazon S3 within your Java projects.
To Wrap Things Up
Efficient file transfer is a crucial aspect of Java development, and leveraging tools like Maven and services like Amazon S3 can greatly streamline the process. With the seamless integration of Maven and Amazon S3, developers can automate artifact deployments, simplify file transfers, and ensure consistent storage of resources.
In this blog post, we've explored the configuration, implementation, and best practices for utilizing Maven with Amazon S3 for efficient file transfer. By following the outlined guidelines and best practices, you can elevate the file transfer process within your Java projects, improving efficiency and reliability.
Now that you have a solid understanding of Maven-integrated file transfer with Amazon S3, consider applying these practices to your projects and experience the streamlined efficiency firsthand. Happy coding!