Troubleshooting Navigation Issues in Spring-JSF Integration
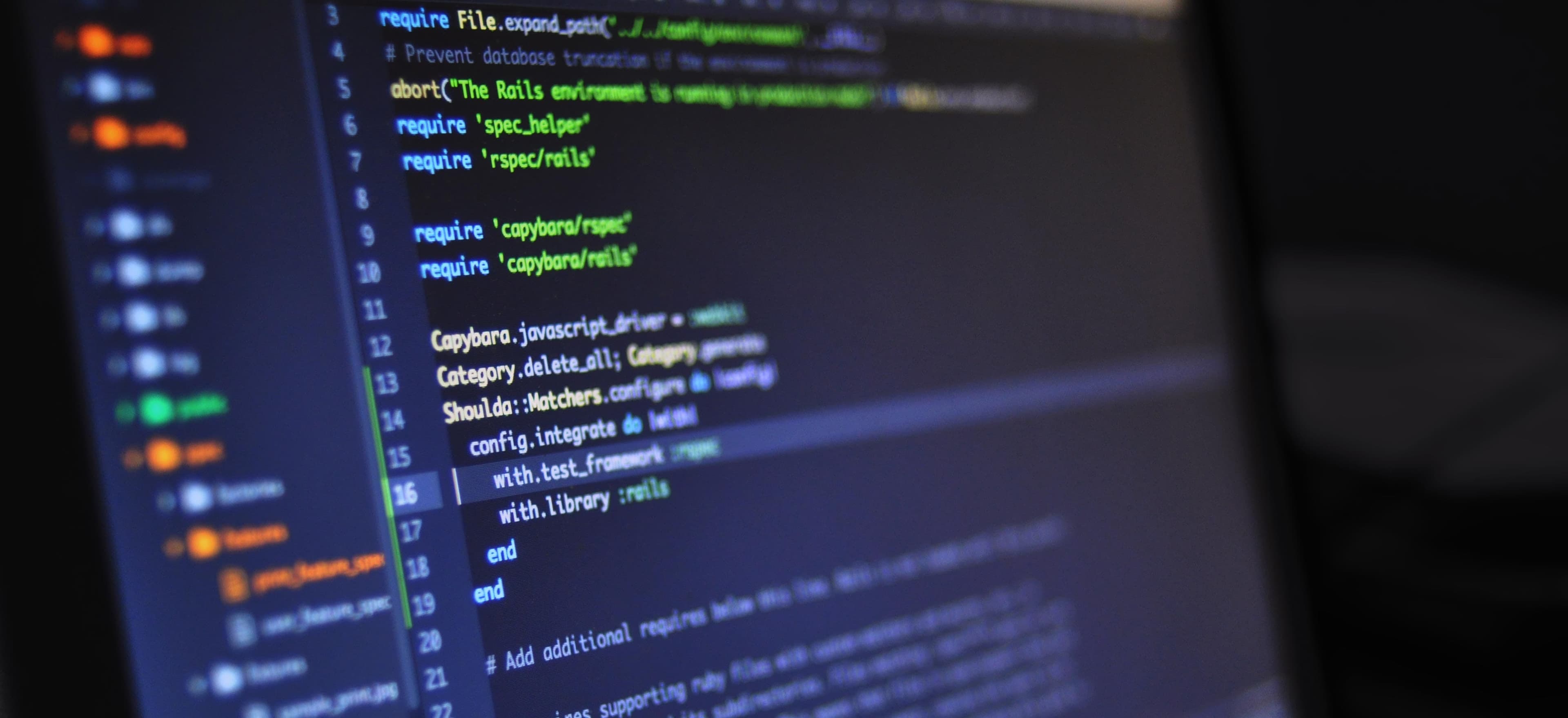
- Published on
Troubleshooting Navigation Issues in Spring-JSF Integration
When working with a Spring-JSF integration, it's common to encounter navigation issues that can be quite frustrating to deal with. Whether you're facing problems with page redirects, view not found errors, or any other navigation-related issues, understanding how to troubleshoot and resolve these issues is crucial for a smooth development process.
In this article, we'll explore common navigation issues in Spring-JSF integration and discuss troubleshooting techniques to resolve them.
Understanding the Problem
Before diving into troubleshooting, it's important to understand the nature of the navigation issue you're facing. Some common navigation issues in Spring-JSF integration include:
-
Page Redirection Problems: When the navigation rules are not properly configured, you might encounter issues with page redirection.
-
View Not Found Errors: This occurs when JSF is unable to locate the specified view.
-
Incorrect Navigation Flow: Issues related to the flow of navigation within the application.
Troubleshooting Navigation Issues
1. Verify Navigation Rules Configuration
The navigation rules in JSF are defined in the faces-config.xml
file or using annotations in managed beans. Ensure that the navigation rules are correctly configured to handle the outcome of the user interactions. Here's an example of navigation rule configuration in faces-config.xml
:
<navigation-rule>
<from-view-id>/start.xhtml</from-view-id>
<navigation-case>
<from-outcome>success</from-outcome>
<to-view-id>/success.xhtml</to-view-id>
</navigation-case>
</navigation-rule>
Why?
It's important to configure the navigation rules accurately because any mismatch here can lead to navigation issues.
2. Check Managed Bean Annotations
If you are using annotations to define navigation rules within managed beans, ensure that the annotations are correctly placed and have the right attributes. Here's an example of a managed bean method with navigation annotations:
@ManagedBean
public class LoginBean {
@ManagedProperty(value="#{userBean}")
private UserBean userBean;
// Action method with navigation rule
@ManagedProperty("#login.xhtml")
public String login() {
if (authenticated) {
return "success";
} else {
return "failure";
}
}
}
Why?
Correctly annotated methods ensure that the navigation flow is properly handled within the managed beans.
3. Use Debugging Tools
Utilize debugging tools provided by Spring and JSF frameworks to trace the navigation flow within the application. This includes logging, breakpoints, and monitoring HTTP requests to understand how the navigation is being handled by the application.
Why?
Debugging tools are essential to identify the specific point of failure in the navigation process.
4. Verify View Mapping
Ensure that the views mapped in the navigation rules exist in the correct location within the project structure. Incorrect view mapping can lead to view not found errors during navigation.
Why?
Correct view mapping is crucial for the navigation flow to work seamlessly.
5. Review URL Patterns Configuration
Check the URL patterns and mappings defined in the web configuration files (web.xml
or Spring's DispatcherServlet
configuration). Incorrect URL patterns can lead to incorrect navigation flow within the application.
Why?
URL patterns play a critical role in directing the user's navigation flow, and any discrepancies can cause navigation issues.
6. Handle Exception Cases
Implement exception handling to gracefully manage navigation failures. This includes handling exceptions such as ViewExpiredException
and NavigationHandler
.
Why?
Exception handling ensures that even in the event of navigation failures, the application can gracefully manage the error and provide a better user experience.
Final Considerations
Troubleshooting navigation issues in Spring-JSF integration requires a systematic approach to identify and resolve the root cause of the problem. By carefully examining the navigation rules, annotations, view mappings, and utilizing debugging tools, developers can effectively address and fix navigation-related issues in their Spring-JSF applications.
Remember, understanding the intricacies of the navigation flow and leveraging the debugging tools provided by the frameworks are essential for efficiently troubleshooting navigation issues in Spring-JSF integration.
For further reading, you can check out the official documentation for Spring Framework and JavaServer Faces to deepen your understanding of navigation in the context of these frameworks.
Happy coding!