Common Pitfalls in Transitioning to Apache Spark
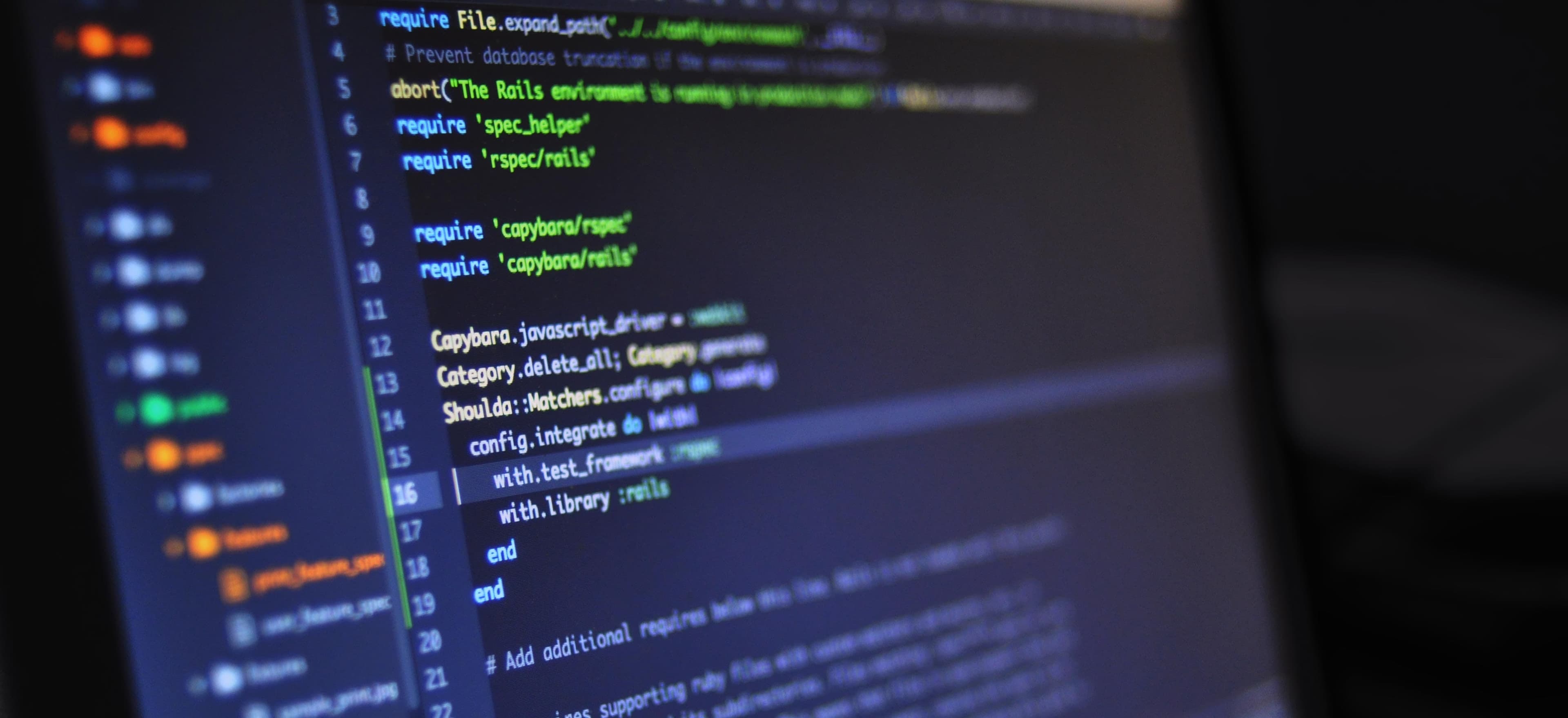
- Published on
Common Pitfalls in Transitioning to Apache Spark
Apache Spark has gained popularity in the world of big data processing due to its speed, ease of use, and versatile capabilities for advanced analytics. However, transitioning to Apache Spark can present several challenges, especially for developers who are accustomed to traditional data processing frameworks. In this article, we will explore some common pitfalls that developers may encounter when transitioning to Apache Spark and discuss strategies to overcome them.
1. Overlooking the DAG Execution Model
One of the common pitfalls when transitioning to Apache Spark is overlooking the Directed Acyclic Graph (DAG) execution model. Unlike traditional data processing frameworks, Apache Spark uses a DAG to represent the logical execution plan of the computation. Each transformation and action in Spark creates a new stage in the DAG, and understanding the DAG execution model is crucial for optimizing the performance of Spark jobs.
Example:
// Example of creating a simple Spark DAG
JavaRDD<String> lines = sc.textFile("hdfs://...");
JavaRDD<Integer> lineLengths = lines.map(s -> s.length());
int totalLength = lineLengths.reduce((a, b) -> a + b);
In this example, the map
and reduce
operations create distinct stages in the DAG, and developers need to be mindful of the DAG structure when designing Spark workflows for efficient execution.
2. Neglecting Data Partitioning
Another common pitfall is neglecting data partitioning, which can significantly impact the performance of Apache Spark jobs. In Spark, data partitioning controls the distribution of data across the cluster, and appropriate partitioning is essential for achieving parallelism and minimizing data shuffling during transformations.
Developers should carefully consider the partitioning strategy based on the nature of the data and the operations to be performed, ensuring that data is evenly distributed across the executors to avoid skewed workloads.
Example:
// Example of explicit data partitioning in Spark
JavaRDD<String> data = sc.textFile("hdfs://...").repartition(10);
In this example, the repartition
method is used to explicitly control the number of partitions in the RDD, allowing developers to optimize the data distribution for parallel processing.
3. Underestimating Memory Management
Memory management is a critical aspect of Apache Spark, and underestimating the importance of efficient memory usage can lead to performance issues and job failures. Spark relies heavily on in-memory processing for intermediate data storage and caching, and developers need to carefully manage memory allocation to prevent out-of-memory errors and excessive garbage collection.
Understanding the memory hierarchy in Spark, including the use of memory fractions, storage levels, and off-heap memory, is essential for optimizing memory utilization and improving job performance.
Example:
// Example of setting memory configuration in Spark
SparkConf conf = new SparkConf().setAppName("MyApp")
.set("spark.executor.memory", "4g")
.set("spark.storage.memoryFraction", "0.5");
In this example, the memory configuration is explicitly set to allocate 4GB for executor memory and reserve 50% of the memory for storage, demonstrating the importance of fine-tuning memory management in Spark applications.
4. Ignoring Data Skew and Hotspotting
Data skew and hotspotting can have detrimental effects on the performance of Apache Spark jobs, yet they are often overlooked during the transition. Skewed data distribution or hotspotting occurs when certain keys or partitions have significantly more data than others, leading to unequal processing times across the cluster and potential job failures.
Developers should identify and address data skew issues through techniques such as data repartitioning, bucketing, or using specialized join strategies to mitigate the impact of skewed data distribution.
Example:
// Example of using bucketing to mitigate data skew
Dataset<Row> df = spark.read().format("parquet").load("path/to/data");
df.write().bucketBy(100, "col_name").saveAsTable("bucketed_table");
In this example, bucketing the data based on a specific column can help distribute the data evenly across partitions, reducing the impact of data skew during processing.
5. Lack of Understanding of Lazy Evaluation
Apache Spark employs lazy evaluation, where transformations are not executed immediately, but rather deferred until an action is triggered. Developers transitioning to Spark often overlook this aspect, leading to inefficient code design and unnecessary computation.
Understanding lazy evaluation is essential for composing efficient Spark workflows, as it enables optimizations such as pipelining and fusion of operations to minimize unnecessary data materialization and improve performance.
Example:
// Example of lazy evaluation in Spark
Dataset<Row> df = spark.read().format("csv").load("path/to/data");
Dataset<Row> filteredData = df.filter(col("age").gt(18));
// The 'filter' transformation is lazily evaluated and not executed immediately
filteredData.show();
In this example, the filter
transformation is deferred until the show
action is invoked, showcasing the lazy evaluation behavior of Spark.
To Wrap Things Up
In conclusion, transitioning to Apache Spark offers immense potential for scalable and high-performance data processing, but it requires developers to be mindful of potential pitfalls and best practices for effective utilization. By avoiding common pitfalls such as overlooking the DAG execution model, neglecting data partitioning, underestimating memory management, ignoring data skew, and lacking understanding of lazy evaluation, developers can ensure smooth and efficient adoption of Apache Spark in their data workflows.
As developers delve into the realm of Apache Spark, a deep understanding of its underlying principles and best practices will empower them to harness the full potential of this powerful big data framework.
Incorporating strategies to overcome common pitfalls in transitioning to Apache Spark can lead to optimized performance and streamlined data processing, paving the way for successful integration of Spark into modern data ecosystems.
For further exploration of Apache Spark best practices and optimization techniques, refer to the official Apache Spark documentation and community resources to stay updated with the latest advancements and insights in the world of big data processing.