Troubleshooting HTTPS Configuration for Servlets
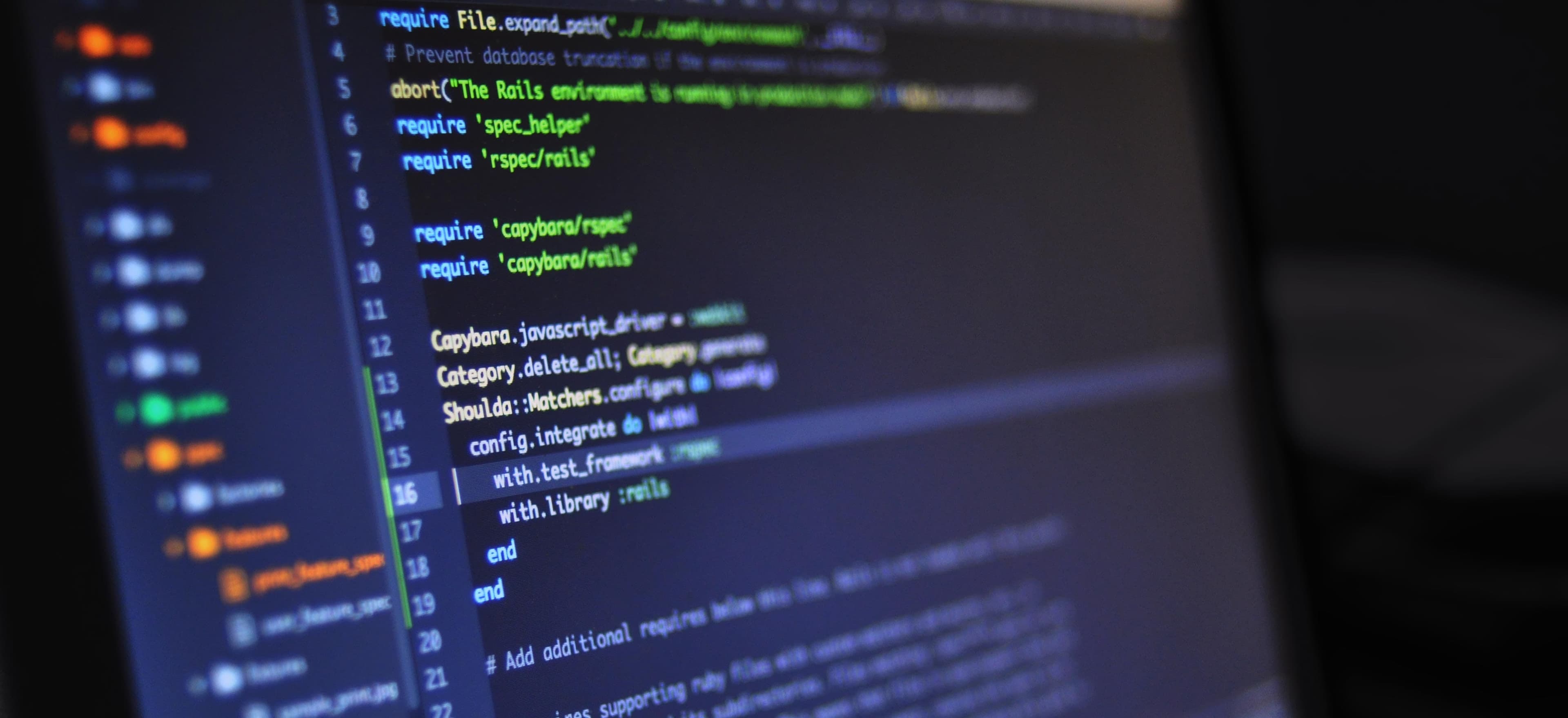
- Published on
Troubleshooting HTTPS Configuration for Servlets
Securing your web applications with HTTPS is crucial for protecting sensitive data. When working with Java servlets, configuring HTTPS is essential to ensure secure communication between the client and the server. However, setting up HTTPS for servlets can sometimes be challenging, and troubleshooting issues that arise is equally important.
In this article, we will explore common problems that developers encounter when configuring HTTPS for servlets and provide solutions to address these issues effectively.
Understanding the Basics
Before diving into troubleshooting, let's review the basic steps for configuring HTTPS in a servlet environment.
Acquiring a Certificate
The first step in setting up HTTPS is acquiring a digital certificate from a trusted Certificate Authority (CA). This certificate is used to authenticate the server to clients and establish a secure connection.
Configuring the Servlet Container
Next, the servlet container, such as Apache Tomcat or Jetty, needs to be configured to support HTTPS. This involves specifying the keystore file that contains the server's private key and digital certificate.
Updating the Servlet Code
Finally, the servlet code must be updated to ensure that it can handle HTTPS requests appropriately. This may involve enforcing HTTPS for certain URLs, redirecting HTTP requests to HTTPS, or handling secure session management.
Common Issues and Solutions
Now, let's delve into some common issues that developers encounter when configuring HTTPS for servlets and explore the solutions for these problems.
Issue 1: Invalid or Self-Signed Certificate
Problem:
When setting up HTTPS, the use of an invalid or self-signed certificate may result in security warnings or errors in web browsers, preventing clients from accessing the application securely.
Solution:
Obtain a valid SSL certificate from a trusted CA. Services like Let's Encrypt provide free SSL/TLS certificates, enabling you to secure your servlets without incurring additional costs.
Issue 2: Keystore Configuration Errors
Problem:
Incorrect configuration of the keystore in the servlet container can lead to HTTPS connections failing to establish.
Solution:
Double-check the keystore configuration in the servlet container's configuration files. Ensure that the keystore file path, password, and alias are correctly specified.
Here's an example of configuring HTTPS in Apache Tomcat's server.xml
:
<Connector
protocol="org.apache.coyote.http11.Http11NioProtocol"
port="8443" maxThreads="200"
scheme="https" secure="true" SSLEnabled="true"
keystoreFile="/path/to/keystore/file"
keystorePass="keystore_password"
clientAuth="false" sslProtocol="TLS"/>
Issue 3: Mixed Content Errors
Problem:
After enabling HTTPS, you may encounter "mixed content" errors, where the web application includes both secure (HTTPS) and non-secure (HTTP) resources.
Solution:
Update the servlet code and any associated web pages to ensure that all resource references, such as images, scripts, and stylesheets, are loaded using HTTPS. This includes using relative URLs or protocol-relative URLs (//
) to reference resources.
Issue 4: Redirect Loops
Problem:
Incorrect redirection configurations may lead to redirect loops, causing the application to become inaccessible.
Solution:
Review the servlet code or web server configuration to ensure that HTTP requests are correctly redirected to HTTPS, and that HTTPS requests are not inadvertently redirected back to HTTP.
Here's an example of redirecting HTTP requests to HTTPS in a servlet:
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
if (!request.isSecure()) {
String redirectUrl = request.getRequestURL().toString().replace("http:", "https:");
response.sendRedirect(redirectUrl);
} else {
// Handle secure requests
}
}
Issue 5: SSL/TLS Protocol Version Compatibility
Problem:
Incompatibility between the SSL/TLS protocol versions supported by the client and server can result in handshake failures or connectivity issues.
Solution:
Ensure that the servlet container is configured to support modern SSL/TLS protocol versions, such as TLS 1.2, and disable older, less secure protocols like SSLv3. Additionally, keep the server's SSL/TLS libraries updated to address any security vulnerabilities.
Key Takeaways
Configuring HTTPS for servlets is essential for ensuring secure communication between clients and servers. By understanding the common issues and their solutions, developers can effectively troubleshoot HTTPS configuration problems in their servlet environments. Remember to prioritize security best practices and stay updated with the latest developments in SSL/TLS protocols to maintain a robust HTTPS configuration for your servlets.