Effective Exception Handling in Spring REST Services
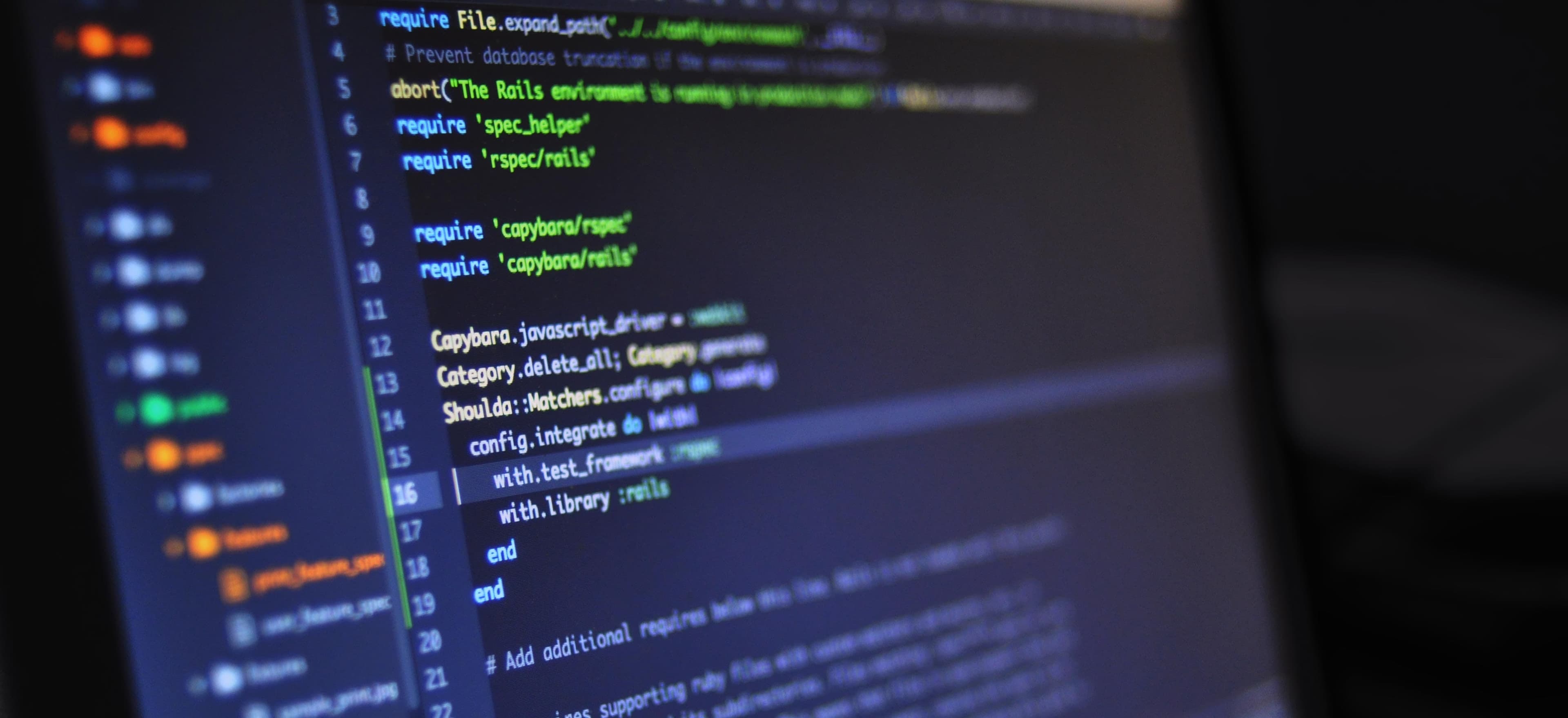
- Published on
Effective Exception Handling in Spring REST Services
Exception handling is a crucial aspect of any robust software application. When it comes to building RESTful services using the Spring framework, proper handling of exceptions becomes even more important. In this post, we'll delve into the best practices and strategies for effective exception handling in Spring REST services.
The Importance of Exception Handling in REST Services
RESTful services are designed to be stateless, which means they don't maintain client state on the server. When an error occurs during the processing of a REST request, it's vital to communicate this error back to the client in a clear and consistent manner. Proper exception handling ensures that the API consumers receive meaningful error messages, status codes, and relevant details to understand and address the issue.
Custom Exception Handling in Spring
In a Spring-based REST service, the central mechanism for handling exceptions is the @ControllerAdvice
annotation. This annotation allows us to consolidate our exception handling logic into one global component. By creating a class annotated with @ControllerAdvice
, we can define methods to handle specific exceptions and apply custom error responses across the application.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public ResponseEntity<String> handleResourceNotFoundException(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("Resource not found");
}
@ExceptionHandler(BadRequestException.class)
@ResponseStatus(HttpStatus.BAD_REQUEST)
public ResponseEntity<String> handleBadRequestException(BadRequestException ex) {
return ResponseEntity.status(HttpStatus.BAD_REQUEST).body("Bad request");
}
// More exception handling methods can be added here
}
In the above example, the GlobalExceptionHandler
class defines methods to handle ResourceNotFoundException
and BadRequestException
. When these exceptions are thrown within the application, the corresponding handler methods will return custom HTTP status codes and error messages.
Global Error Handling Configuration
To further enhance exception handling, and to ensure that exceptions are processed consistently, we can extend the ResponseEntityExceptionHandler
class. By doing so, we can reuse common exception handling logic across different types of exceptions.
@ControllerAdvice
public class GlobalExceptionHandler extends ResponseEntityExceptionHandler {
@Override
protected ResponseEntity<Object> handleMethodArgumentNotValid(MethodArgumentNotValidException ex,
HttpHeaders headers,
HttpStatus status,
WebRequest request) {
// Custom logic for handling validation errors
}
@Override
protected ResponseEntity<Object> handleHttpMessageNotReadable(HttpMessageNotReadableException ex,
HttpHeaders headers,
HttpStatus status,
WebRequest request) {
// Custom logic for handling malformed JSON requests
}
// More overridden methods to handle specific exceptions
}
By extending ResponseEntityExceptionHandler
and overriding its methods, we can define consistent error handling logic for various types of exceptions, such as method argument validation errors and unreadable JSON requests.
Exception Handling for Specific Controllers
While global exception handling is powerful and convenient, there are scenarios where we need to apply custom exception handling logic specific to a particular controller or endpoint. In such cases, we can utilize the @ExceptionHandler
annotation within the controller class to handle exceptions locally.
@RestController
public class UserController {
@Autowired
private UserService userService;
@ExceptionHandler(UserNotFoundException.class)
@ResponseStatus(HttpStatus.NOT_FOUND)
public ResponseEntity<String> handleUserNotFoundException(UserNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("User not found");
}
@GetMapping("/users/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.getUserById(id);
if (user == null) {
throw new UserNotFoundException();
}
return ResponseEntity.ok(user);
}
// Other handler methods and controller endpoints
}
In the UserController
class, the handleUserNotFoundException
method handles the UserNotFoundException
locally for the specific controller. This ensures that when the exception is thrown within the UserController
, it will be handled with the custom logic defined in the local handler method.
Standardizing Error Responses
Consistency is key when it comes to communicating errors in a RESTful service. To standardize error responses across the application, we can create a custom ErrorResponse
class to encapsulate error details such as status code, error message, timestamp, and any additional information.
public class ErrorResponse {
private int status;
private String message;
private String timestamp;
// Getters, setters, and constructor
}
Then, we can modify our exception handling methods to return instances of ErrorResponse
instead of raw strings or predefined HTTP responses. By doing so, we ensure that all error responses adhere to a consistent structure, making it easier for API consumers to interpret and handle errors.
In Conclusion, Here is What Matters
In a Spring-based REST service, effective exception handling is crucial for providing a reliable and user-friendly API experience. Utilizing @ControllerAdvice
for global exception handling, extending ResponseEntityExceptionHandler
for consistent error processing, and leveraging @ExceptionHandler
at the controller level enables us to build a robust exception handling strategy. Standardizing error responses further enhances the clarity and coherence of the API's error communication. By implementing these best practices, we can ensure that our Spring REST services deliver informative and consistent error responses, ultimately enhancing the overall reliability and usability of our APIs.
Exception handling in Spring REST services is an essential aspect of ensuring the reliability and usability of the API. Proper exception handling can significantly improve user experience and simplify debugging and troubleshooting processes. By following best practices and leveraging the powerful features of Spring, developers can create robust and reliable RESTful services that effectively communicate errors to their consumers.