Handling File Copy and Movement with Apache Commons IO
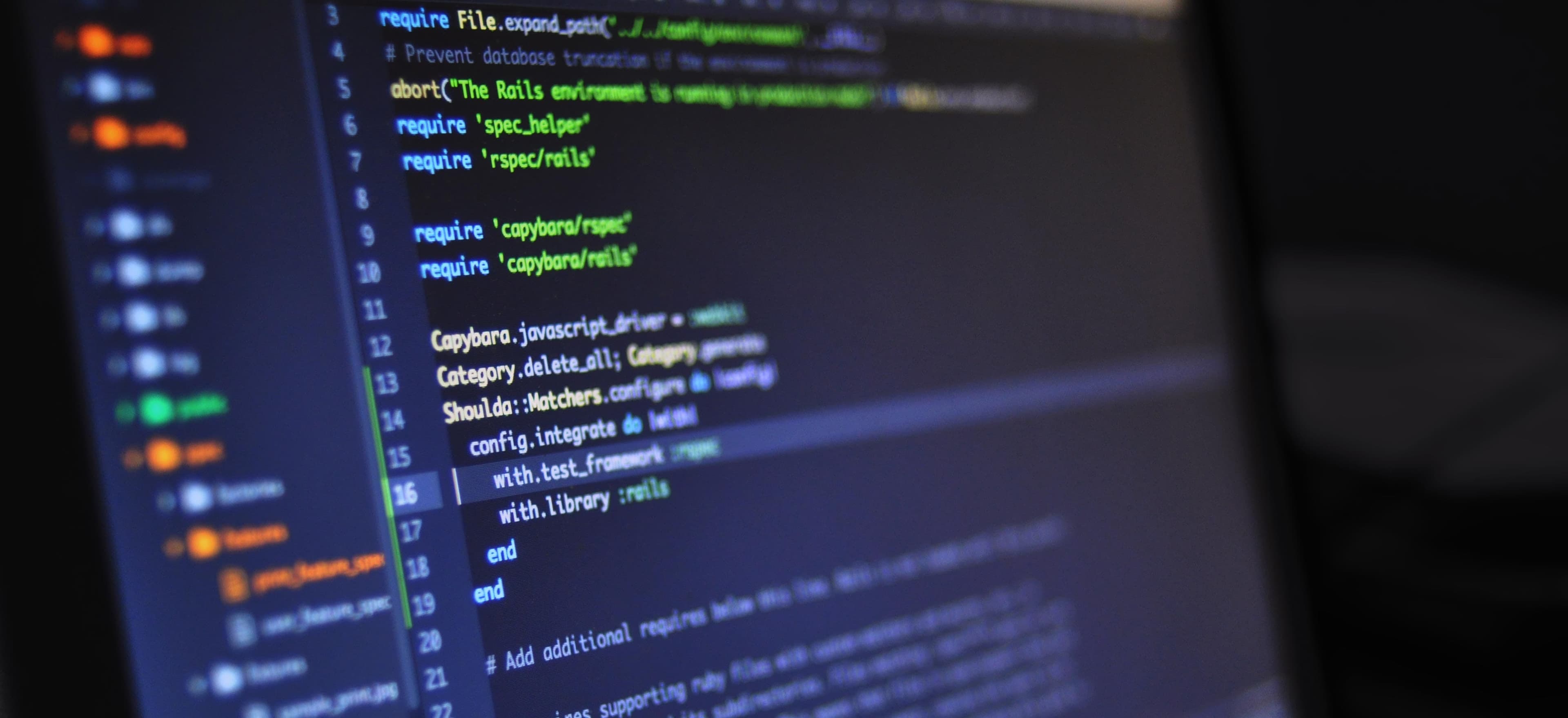
- Published on
Handling File Copy and Movement with Apache Commons IO
When it comes to handling file operations in Java, Apache Commons IO library is a powerful ally. This open-source library provides a plethora of utilities for working with files and file systems, making common tasks such as file copy and movement a breeze.
In this blog post, we will delve into using Apache Commons IO to copy and move files in Java, exploring the various functionalities offered by the library and providing code examples to demonstrate their usage.
Why Use Apache Commons IO for File Operations?
Apache Commons IO simplifies file handling in Java by offering high-level abstractions for common file-related tasks. It provides methods for file copy, deletion, comparison, and manipulation, among others, streamlining the process of working with files and directories.
By using Apache Commons IO, developers can avoid writing repetitive boilerplate code for file operations and instead leverage the efficient and well-tested utilities provided by the library. This not only saves time and effort but also ensures more robust and maintainable code.
Let's dive into the specifics of copying and moving files using Apache Commons IO.
File Copy with FileUtils
The FileUtils
class in Apache Commons IO provides a simple and effective way to copy files in Java. It offers the copyFile
method, which takes the source file and the destination file as arguments and performs the file copy operation.
Here's an example demonstrating how to use copyFile
to copy a file:
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
public class FileCopyExample {
public static void main(String[] args) {
File sourceFile = new File("path/to/source/file.txt");
File destinationFile = new File("path/to/destination/file.txt");
try {
FileUtils.copyFile(sourceFile, destinationFile);
System.out.println("File copied successfully!");
} catch (IOException e) {
System.err.println("Error copying file: " + e.getMessage());
}
}
}
In this example, we import FileUtils
from Apache Commons IO and use its copyFile
method to copy a source file to a destination file. We also handle potential IOException
that may occur during the file copy operation.
File Movement with FileUtils
Similar to file copy, Apache Commons IO provides a straightforward way to move files using the FileUtils
class. The moveFile
method allows us to move a file from one location to another.
Let's take a look at an example showcasing how to move a file using moveFile
:
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
public class FileMoveExample {
public static void main(String[] args) {
File sourceFile = new File("path/to/source/file.txt");
File destinationFile = new File("path/to/destination/file.txt");
try {
FileUtils.moveFile(sourceFile, destinationFile);
System.out.println("File moved successfully!");
} catch (IOException e) {
System.err.println("Error moving file: " + e.getMessage());
}
}
}
In this example, we utilize the moveFile
method from FileUtils
to move a file from a source location to a destination location. As with file copy, we handle any potential IOException
that may arise during the file movement operation.
Handling File Copy and Movement Across File Systems
One of the notable features of Apache Commons IO is its ability to handle file copy and movement across different file systems seamlessly. This means that the library can effectively copy or move files between, for example, the local file system and a network-attached storage (NAS) or a remote file system.
This capability comes in handy when dealing with diverse deployment environments or when transitioning files between various storage systems. Apache Commons IO abstracts away the underlying complexities, providing a uniform interface for file operations regardless of the file systems involved.
Closing the Chapter
Apache Commons IO proves to be a valuable asset for handling file copy and movement in Java. Its intuitive APIs for file manipulation, including the FileUtils
class, simplify common file operations and facilitate seamless interaction with file systems.
By leveraging Apache Commons IO, developers can streamline file handling tasks, enhance code readability, and ensure cross-compatibility across different file systems. Whether it's copying files within the same file system or moving files across diverse storage environments, Apache Commons IO offers a robust and user-friendly solution.
In conclusion, mastering the capabilities of Apache Commons IO empowers Java developers to navigate file operations with ease and efficiency, while maintaining clean and concise code.
For further reading, you can explore the official documentation of Apache Commons IO and deepen your understanding of its extensive features and utilities.
Start integrating Apache Commons IO into your file handling workflows and witness the transformative impact it can have on your Java projects!