Effective Unit Testing for Java Streams
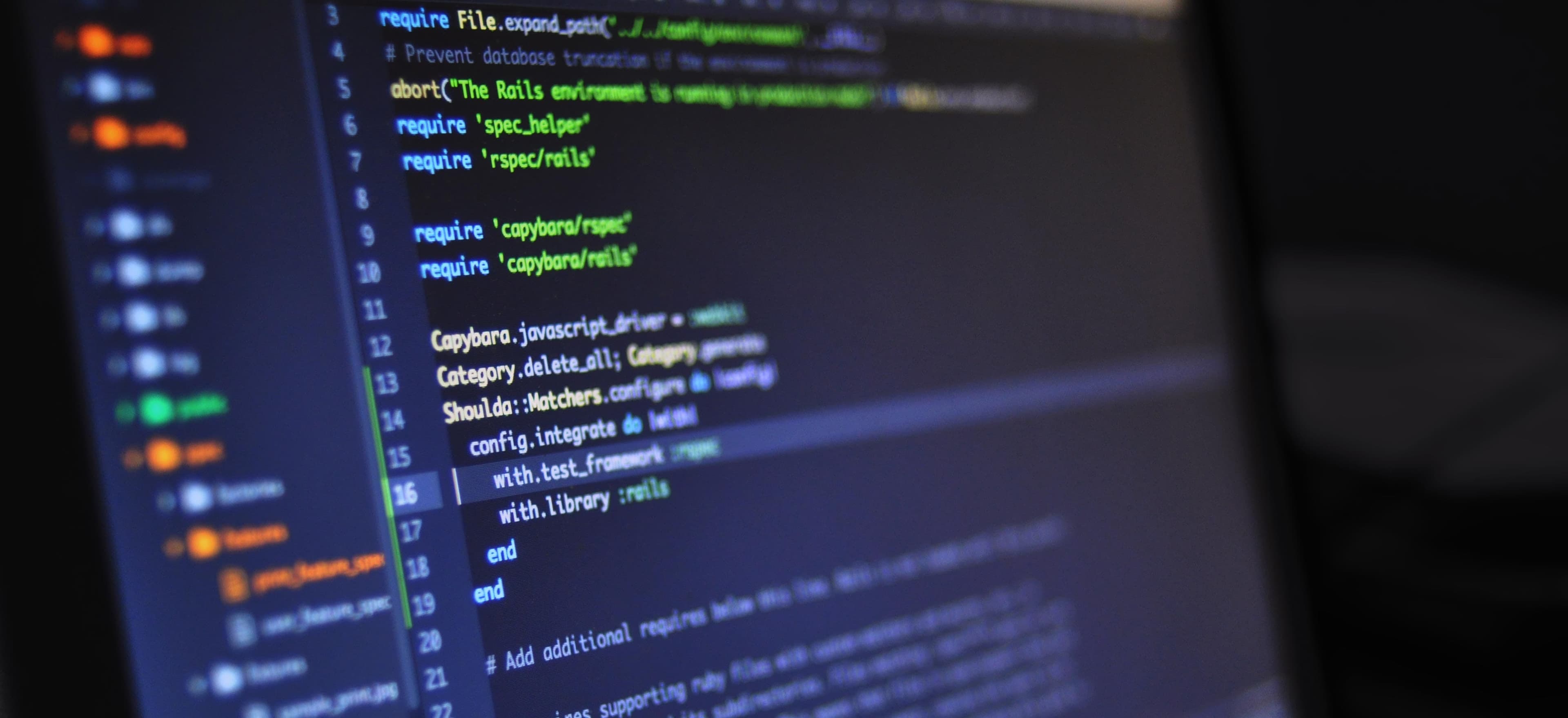
- Published on
Effective Unit Testing for Java Streams
Java 8 introduced the Stream API, which provides a functional approach to processing collections of objects. Streams allow developers to express sophisticated data processing queries concisely. However, testing code that uses streams can be challenging due to the functional nature of streams and the potential for complex transformations and intermediate operations. In this blog post, we'll explore effective strategies for unit testing Java streams to ensure robust and reliable code.
Understanding the Basics of Java Streams
Before diving into unit testing, it's crucial to have a solid understanding of Java streams. Streams are sequences of elements available from a collection or other source of data. They facilitate functional-style operations on collections, such as mapping, filtering, and reducing. Streams promote declarative programming by allowing developers to specify what operations they want to perform on the data, rather than how to perform them.
Let's consider an example of using streams to filter a list of numbers greater than 10:
List<Integer> numbers = Arrays.asList(5, 12, 3, 18, 9);
List<Integer> filteredNumbers = numbers.stream()
.filter(num -> num > 10)
.collect(Collectors.toList());
In this example, we use the filter
operation to create a new stream containing only elements that satisfy the given predicate. The collect
operation then converts the filtered stream into a list.
Challenges of Unit Testing Java Streams
When it comes to unit testing code that uses Java streams, several challenges arise:
1. Functional nature
Streams encourage a functional programming style, which can make it difficult to isolate and test individual stream operations.
2. Intermediate operations
Streams often involve a series of intermediate and terminal operations. Testing a particular intermediate operation independently of others can be challenging.
3. Complex transformations
Stream operations, such as mapping and reducing, can lead to complex transformations of data, making it harder to write meaningful and concise test cases.
Given these challenges, it's essential to adopt effective strategies for unit testing Java stream operations to ensure code correctness and maintainability.
Strategies for Effective Unit Testing
Strategy 1: Isolate Stream Operations
When testing code that involves stream operations, it's essential to isolate each operation for individual testing. This can be achieved by breaking down complex stream pipelines into smaller, testable components.
Let's consider an example where we want to test a method that processes a list of strings using a stream:
public String concatenateStrings(List<String> strings) {
return strings.stream()
.map(String::toUpperCase)
.collect(Collectors.joining(" "));
}
To effectively test the concatenateStrings
method, we can break down the stream operations into separate testable methods for mapping and joining:
public String mapToUppercase(List<String> strings) {
return strings.stream()
.map(String::toUpperCase)
.collect(Collectors.joining(" "));
}
public String joinStrings(List<String> strings, String delimiter) {
return strings.stream()
.collect(Collectors.joining(delimiter));
}
By isolating the stream operations into separate methods, we can test each operation independently, ensuring that they produce the expected results.
Strategy 2: Use Mocked Data
In unit testing, using realistic but controlled data is crucial for validating the behavior of stream operations. By using mocked data, we can create predictable scenarios and cover edge cases that might not be present in actual production data.
Consider the following example of testing a method that filters a list of employees based on their department:
public List<Employee> filterByDepartment(List<Employee> employees, String department) {
return employees.stream()
.filter(employee -> employee.getDepartment().equals(department))
.collect(Collectors.toList());
}
In the corresponding unit test, we can use mocked employee data to cover various department scenarios and edge cases, ensuring the filter operation behaves as expected.
Strategy 3: Assert Intermediary and Terminal States
When testing methods that involve stream operations, it's crucial to assert the intermediary and terminal states of the stream. Verifying the intermediate states of a stream ensures that the transformation or filtering is performed correctly, while asserting the terminal state validates the final result of the stream operations.
@Test
public void testFilterByDepartment() {
List<Employee> employees = Arrays.asList(
new Employee("John", "Engineering"),
new Employee("Jane", "Sales"),
new Employee("Doe", "Engineering")
);
List<Employee> filteredEmployees = filterByDepartment(employees, "Engineering");
assertEquals(2, filteredEmployees.size());
assertTrue(filteredEmployees.stream().allMatch(employee -> employee.getDepartment().equals("Engineering")));
}
In this example, we test the filterByDepartment
method by asserting the size and department property of the filtered employees, covering both the intermediary and terminal states of the stream operation.
Strategy 4: Test Exceptional Cases
It's vital to test the handling of exceptional cases when working with stream operations. This includes scenarios where the stream may be empty, or when certain operations may throw exceptions.
public Optional<String> findLongestString(List<String> strings) {
return strings.stream()
.max(Comparator.comparingInt(String::length));
}
When unit testing the findLongestString
method, we should include tests for empty input lists or scenarios where the input list contains null elements to ensure the method behaves as expected in exceptional cases.
Lessons Learned
Unit testing Java streams can be challenging due to their functional nature and complex operations. By adopting effective strategies such as isolating stream operations, using mocked data, asserting intermediary and terminal states, and testing exceptional cases, developers can ensure the reliability and correctness of code that uses Java streams. Embracing these strategies not only leads to robust and maintainable code but also enhances the overall quality of software development.
In conclusion, mastering unit testing for Java streams is essential for delivering high-quality and reliable software, and it requires a deep understanding of stream operations and effective testing methodologies. By following the strategies outlined in this post, developers can tackle the challenges of testing Java streams with confidence and precision, leading to more robust and maintainable codebases.