Solving Synchronization Issues in Multithreaded Integration Tests
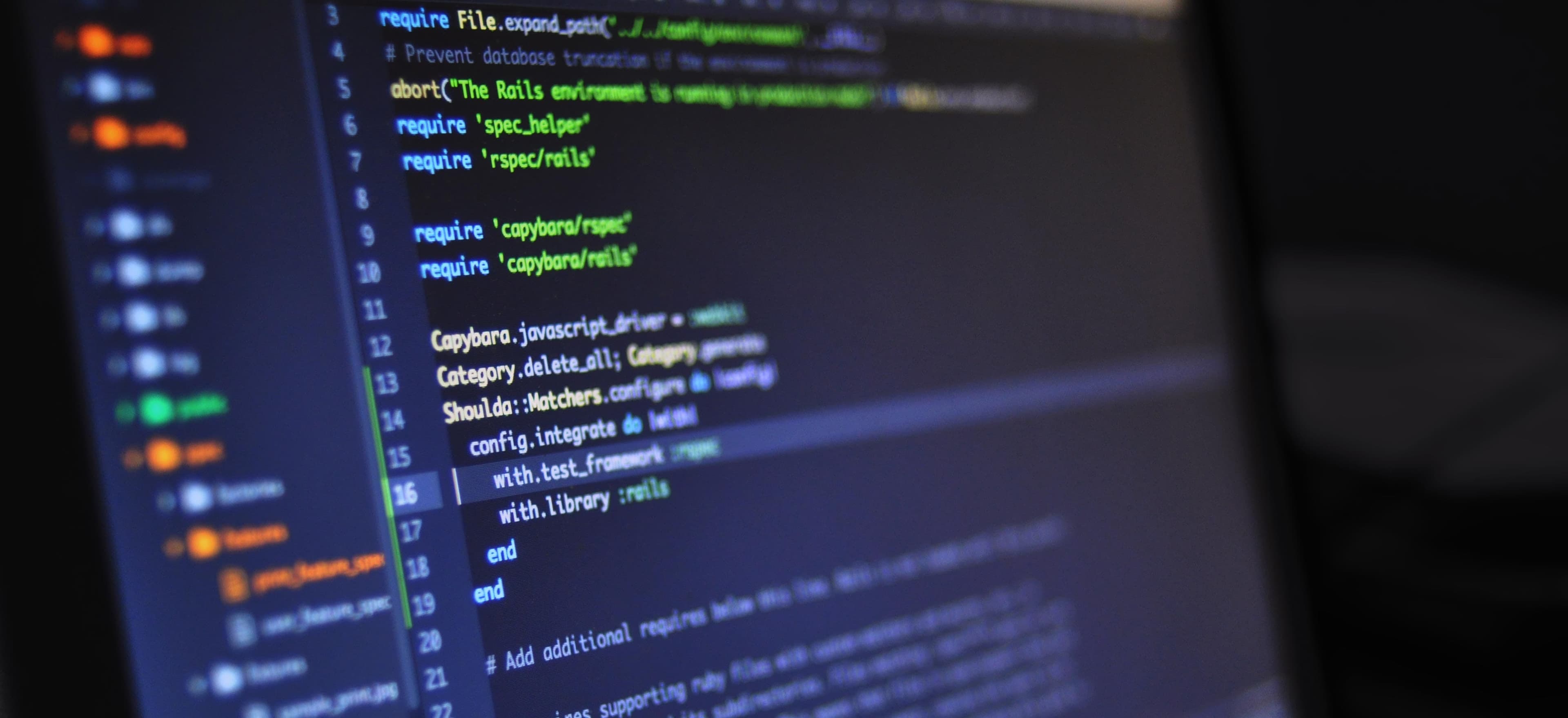
- Published on
Solving Synchronization Issues in Multithreaded Integration Tests
When writing integration tests for multithreaded applications, one of the most common challenges developers face is dealing with synchronization issues. These issues can lead to flaky tests, making it difficult to validate the correctness of the application's behavior under concurrent conditions.
In this article, we'll explore some common synchronization issues that can arise in multithreaded integration tests and discuss strategies for solving them using Java.
Understanding the Problem
Multithreaded integration tests involve simulating real-world scenarios where multiple threads interact with the application concurrently. This can uncover synchronization issues that may not be apparent in single-threaded tests. These issues can manifest as race conditions, deadlock, or inconsistent state due to concurrent modifications.
Let's consider a scenario where multiple threads are updating a shared resource concurrently. Without proper synchronization, these updates can interfere with each other, leading to unexpected behavior and test failures.
public class SharedResource {
private int value;
public void increment() {
value++;
}
public int getValue() {
return value;
}
}
In the above example, if multiple threads concurrently invoke the increment
method, the shared value
may not be updated correctly, leading to synchronization issues.
Using Synchronization Constructs
1. Using synchronized
Keyword
One way to address synchronization issues is by using the synchronized
keyword to control access to critical sections of code. By synchronizing the methods or code blocks that modify shared resources, we can ensure that only one thread can access them at a time.
public class SharedResource {
private int value;
public synchronized void increment() {
value++;
}
public synchronized int getValue() {
return value;
}
}
In this modified example, the increment
and getValue
methods are synchronized, preventing concurrent access and ensuring thread safety. While this approach can be effective, it may lead to contention and reduced performance in highly concurrent scenarios.
2. Using Locks
Another approach is to use explicit Lock
objects to manage synchronization. Unlike the implicit locking provided by the synchronized
keyword, Lock
objects offer more flexibility and control over the locking mechanism.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class SharedResource {
private int value;
private Lock lock = new ReentrantLock();
public void increment() {
lock.lock();
try {
value++;
} finally {
lock.unlock();
}
}
public int getValue() {
lock.lock();
try {
return value;
} finally {
lock.unlock();
}
}
}
In this example, we use a ReentrantLock
to guard the critical sections, providing a more granular approach to synchronization. The try-finally
block ensures that the lock is always released, even if an exception occurs.
Dealing with Asynchronous Operations
In multithreaded integration tests, we often need to deal with asynchronous operations, such as making network requests or processing callbacks. Managing the synchronization of such operations is crucial for ensuring the correctness of the test outcomes.
3. Using CountDownLatch
CountDownLatch
is a synchronization aid that allows one or more threads to wait until a set of operations being performed in other threads completes. This can be useful for coordinating asynchronous tasks in integration tests.
import java.util.concurrent.CountDownLatch;
public class AsynchronousOperation {
private CountDownLatch latch = new CountDownLatch(1);
private boolean result;
public void performAsynchronousTask() {
// Simulate an asynchronous task
new Thread(() -> {
// Perform the task
// Set the result
result = true;
latch.countDown();
}).start();
}
public boolean waitForResult() throws InterruptedException {
latch.await();
return result;
}
}
In this example, the performAsynchronousTask
method simulates an asynchronous operation and uses a CountDownLatch
to wait until the task completes. The waitForResult
method blocks until the latch is counted down, ensuring that the result is available before proceeding with the test.
4. Using CompletableFuture
Java 8 introduced the CompletableFuture
class, which provides a powerful way to work with asynchronous computations. It allows chaining asynchronous operations and handling their outcomes using fluent functional APIs.
import java.util.concurrent.CompletableFuture;
public class AsynchronousOperation {
public CompletableFuture<Boolean> performAsynchronousTask() {
// Simulate an asynchronous task
return CompletableFuture.supplyAsync(() -> {
// Perform the task
return true;
});
}
}
In this example, the performAsynchronousTask
method returns a CompletableFuture
that represents the result of the asynchronous task. This makes it easy to perform further operations, such as applying transformations or handling errors, in a composable manner.
Closing the Chapter
Writing multithreaded integration tests can be challenging, especially when dealing with synchronization issues. By using appropriate synchronization constructs and managing asynchronous operations effectively, we can ensure the reliability and consistency of our tests.
In this article, we've covered some common strategies for solving synchronization issues in multithreaded integration tests using Java. Understanding these techniques and applying them thoughtfully can lead to more robust and reliable tests for multithreaded applications.
References:
- Java Concurrency in Practice
- Java Documentation on Locks
- Java Documentation on CompletableFuture