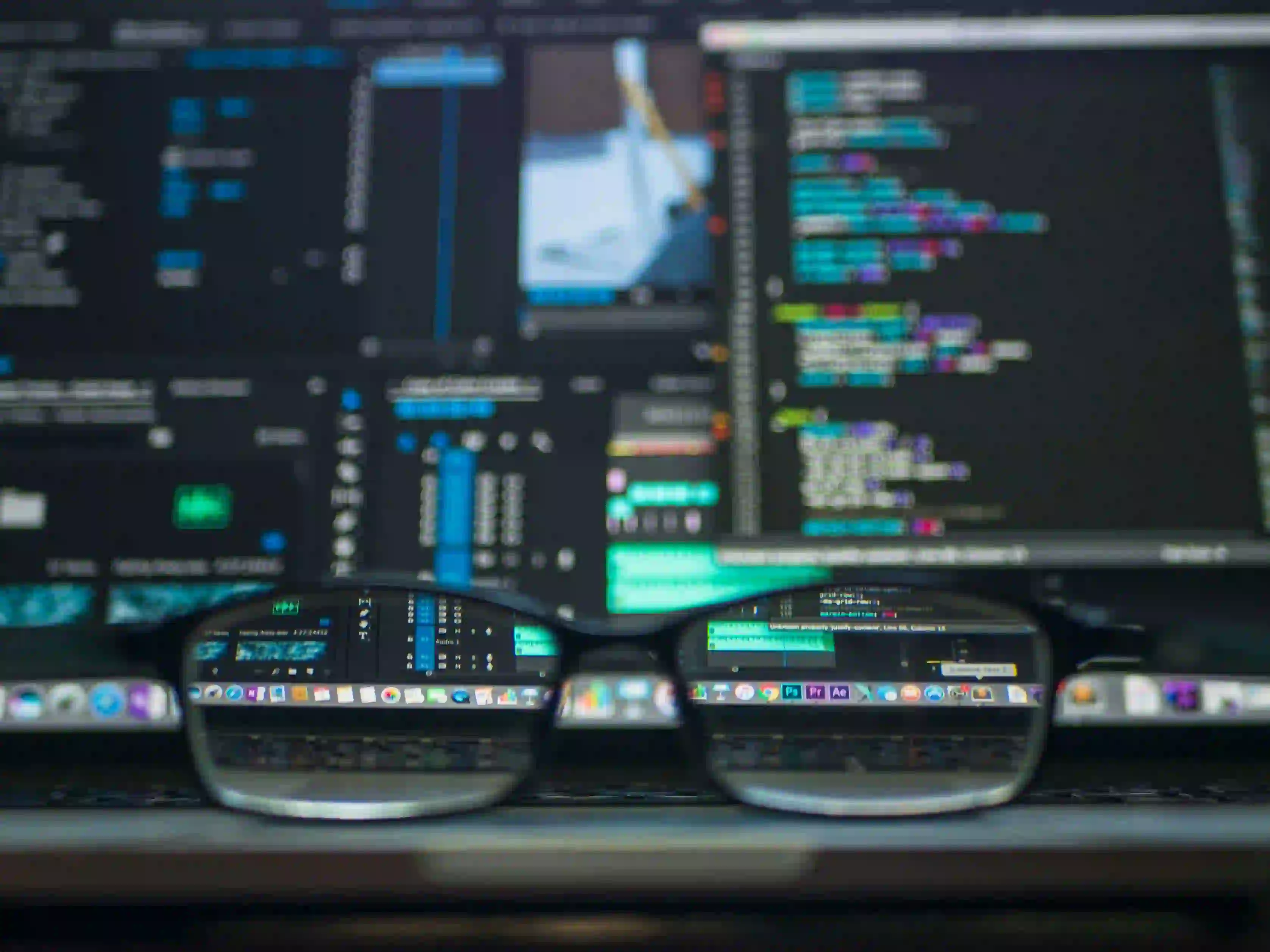
Enforcing Plug-in Policies in JBoss Apiman
In JBoss Apiman, the concept of plug-in policies allows users to define custom logic for managing and securing APIs. However, to maintain a secure and reliable API management system, it is essential to enforce strict policies on the plug-ins that are allowed to be executed within the Apiman runtime. In this blog post, we will explore the process of enforcing plug-in policies in JBoss Apiman and discuss the best practices for ensuring the security and stability of the API management platform.
Understanding Plug-in Policies
Plug-in policies in JBoss Apiman are implemented using the Java language. A plug-in policy is essentially a piece of Java code that is executed at various points in the API lifecycle, such as during API creation, request processing, and response processing. These plug-ins can be used to enforce security measures, perform data transformation, apply rate limiting, and more. However, allowing arbitrary plug-ins to be executed within the Apiman runtime can pose significant security risks if not properly controlled.
Enforcing Plug-in Policies
To enforce plug-in policies in JBoss Apiman, we need to implement a custom plug-in registry that controls which plug-ins are allowed to be registered and executed within the Apiman runtime. This can be achieved by extending the default plug-in registry provided by Apiman and adding custom logic to restrict the registration of plug-ins based on certain criteria.
Let's dive into an example of how we can enforce plug-in policies by implementing a custom plug-in registry in Java.
public class CustomPluginRegistry extends DefaultPluginRegistry {
@Override
public void registerPlugin(Plugin plugin) {
// Check if the plugin meets the defined criteria for registration
if (isPluginAllowed(plugin)) {
super.registerPlugin(plugin);
} else {
throw new SecurityException("Unauthorized plugin registration");
}
}
private boolean isPluginAllowed(Plugin plugin) {
// Add custom logic to validate the plugin before allowing registration
// For example, check if the plugin is signed by a trusted authority
// or if it adheres to predefined security standards
// Return true if the plugin is allowed, false otherwise
}
}
In the above code snippet, we have created a custom plug-in registry by extending the DefaultPluginRegistry
class provided by JBoss Apiman. We have overridden the registerPlugin
method to add custom validation logic before allowing the registration of a plug-in. If the plug-in does not meet the specified criteria, a SecurityException
is thrown, preventing the unauthorized registration of the plug-in.
Best Practices for Enforcing Plug-in Policies
When enforcing plug-in policies in JBoss Apiman, it is important to follow best practices to ensure the effectiveness of the policy enforcement mechanism. Here are some best practices to consider:
-
Code Signing: Require that all plug-ins are signed by a trusted authority before they can be registered and executed within the Apiman runtime. This helps prevent the execution of untrusted or tampered plug-ins.
-
Security Standards: Define and enforce security standards for plug-ins, such as requiring adherence to secure coding practices, input validation, and proper error handling.
-
Whitelisting: Maintain a whitelist of approved plug-ins that have been thoroughly vetted for security and compliance. Only allow the registration and execution of plug-ins that are included in the whitelist.
-
Monitoring and Auditing: Implement a robust monitoring and auditing system to track plug-in registrations and executions, enabling the detection of any unauthorized or suspicious activities.
By incorporating these best practices, organizations can establish a proactive and robust approach to enforcing plug-in policies in JBoss Apiman, mitigating the risks associated with untrusted or malicious plug-ins.
Lessons Learned
Enforcing plug-in policies in JBoss Apiman is essential for maintaining a secure and reliable API management platform. By implementing a custom plug-in registry with strict validation criteria, organizations can control the registration and execution of plug-ins within the Apiman runtime, reducing the likelihood of security vulnerabilities and system instability.
In conclusion, the process of enforcing plug-in policies involves implementing a custom plug-in registry with stringent validation logic, adhering to best practices such as code signing, security standards, whitelisting, and monitoring. By following these guidelines, organizations can enhance the security posture of their API management infrastructure and ensure the integrity of their API ecosystem.
For more information on JBoss Apiman plug-in development and security best practices, refer to the official documentation.
Remember, enforcing plug-in policies is a critical aspect of API management and should be approached with careful consideration and a proactive mindset. With the right policies and practices in place, organizations can confidently leverage the power of JBoss Apiman while maintaining the security and reliability of their APIs.