Overfitting in Binary Classification with TensorFlow 2
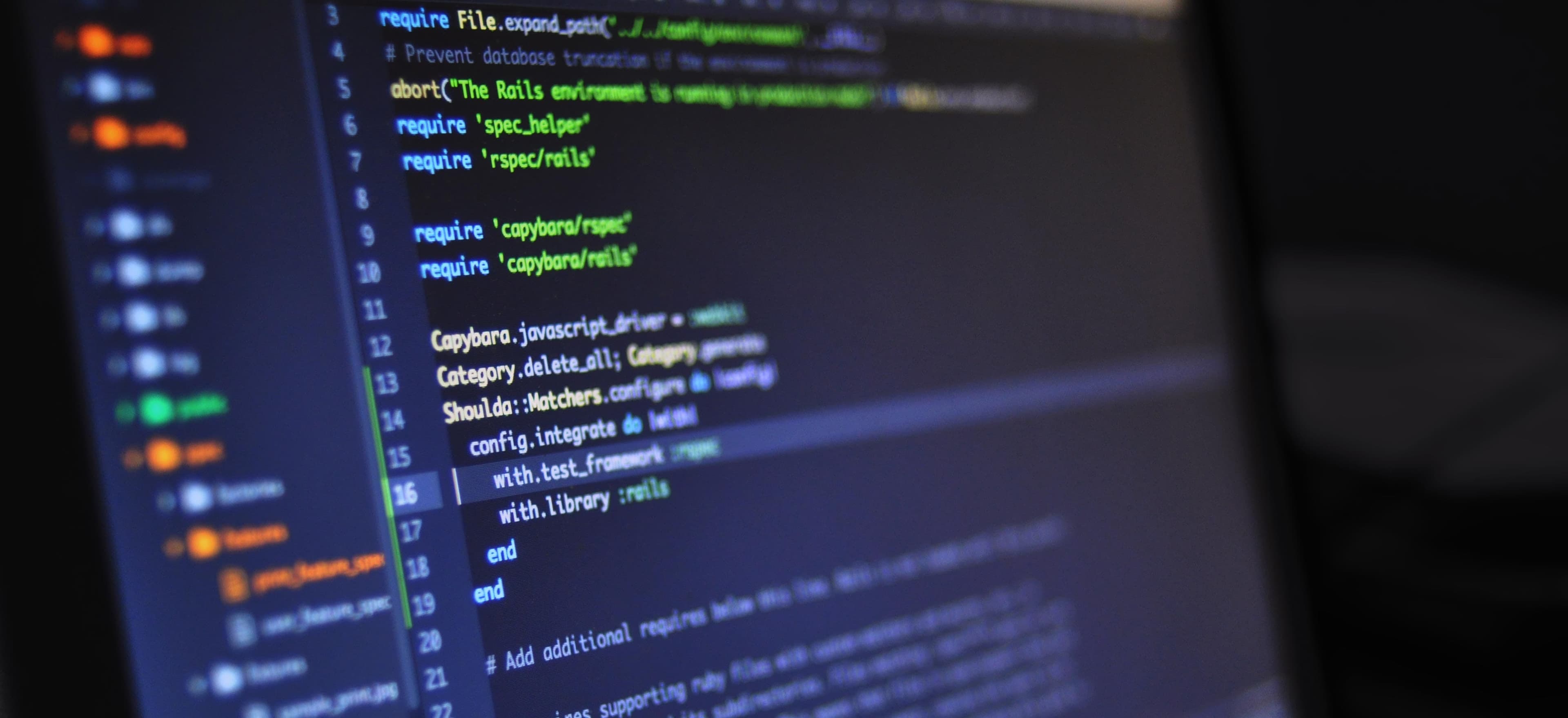
- Published on
Understanding Overfitting in Binary Classification Using TensorFlow 2
When working with machine learning models, one common issue that often arises is overfitting. This is especially true in binary classification problems, where the model's ability to generalize from the training data to new, unseen data is crucial. In this blog post, we will delve into the concept of overfitting in the context of binary classification using TensorFlow 2, a powerful and popular open-source machine learning library.
What is Overfitting?
Overfitting occurs when a machine learning model performs well on the training data but fails to generalize to new, unseen data. In the context of binary classification, this can result in the model making inaccurate predictions when presented with new samples. This phenomenon is often caused by the model learning to identify patterns that are specific to the training data, leading to poor performance on new data.
Data Preparation
To demonstrate overfitting in binary classification, let's start by preparing a synthetic dataset using NumPy. We will create two classes of data points, each belonging to a different category, with some added noise to make the classification problem more challenging.
import numpy as np
import matplotlib.pyplot as plt
# Generating synthetic data
np.random.seed(0)
X = np.random.rand(100, 2)
y = (X[:, 0] + X[:, 1] > 1).astype(int)
# Visualizing the data
plt.scatter(X[y == 0, 0], X[y == 0, 1], label='Class 0')
plt.scatter(X[y == 1, 0], X[y == 1, 1], label='Class 1')
plt.legend()
plt.show()
In the code snippet above, we create a synthetic dataset X
consisting of 100 data points, where each point has two features. The corresponding labels y
are generated based on a simple rule: if the sum of the two features is greater than 1, the label is 1; otherwise, it is 0. We then visualize the dataset to observe the distribution of the two classes.
Building the Model
Next, we will build a simple neural network using TensorFlow 2 to perform binary classification on the synthetic dataset. We will use this model to demonstrate the concept of overfitting and explore techniques to mitigate it.
import tensorflow as tf
from tensorflow.keras import layers
# Creating a simple neural network
model = tf.keras.Sequential([
layers.Dense(10, activation='relu', input_shape=(2,)),
layers.Dense(1, activation='sigmoid')
])
# Compiling the model
model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Training the model
history = model.fit(X, y, epochs=100, validation_split=0.2, verbose=0)
In the code above, we define a simple neural network with one hidden layer containing 10 neurons and an output layer with a single neuron using the Sequential
API provided by TensorFlow's Keras module. We then compile the model using the Adam optimizer and binary cross-entropy loss, which are commonly used for binary classification tasks. The model is trained on the synthetic dataset for 100 epochs, and we monitor the validation accuracy during training.
Visualizing the Training Process
To understand overfitting better, let's visualize the training and validation accuracy over the course of training.
# Visualizing the training process
plt.plot(history.history['accuracy'], label='Train Accuracy')
plt.plot(history.history['val_accuracy'], label='Validation Accuracy')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
By plotting the training and validation accuracy over the epochs, we can observe the behavior of the model during training. If the model begins to perform significantly better on the training data compared to the validation data, it is an indication of overfitting.
Mitigating Overfitting
To mitigate overfitting, we can employ several techniques, such as regularization, dropout, and early stopping. Let's incorporate these techniques into our model and observe their effects.
Regularization
We can add L2 regularization to the neural network's layers to penalize large weights and prevent overfitting.
# Adding L2 regularization
model_regularized = tf.keras.Sequential([
layers.Dense(10, activation='relu', kernel_regularizer=tf.keras.regularizers.l2(0.001), input_shape=(2,)),
layers.Dense(1, activation='sigmoid')
])
# Compiling the regularized model
model_regularized.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Training the regularized model
history_regularized = model_regularized.fit(X, y, epochs=100, validation_split=0.2, verbose=0)
# Visualizing the training process with regularization
plt.plot(history_regularized.history['accuracy'], label='Train Accuracy (Regularized)')
plt.plot(history_regularized.history['val_accuracy'], label='Validation Accuracy (Regularized)')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
By introducing L2 regularization to the model, we can observe whether the overfitting behavior has been mitigated.
Dropout
Another effective technique to combat overfitting is dropout, which involves randomly setting a fraction of input units to 0 at each update during training, preventing units from co-adapting too much.
# Adding dropout layer
model_dropout = tf.keras.Sequential([
layers.Dense(10, activation='relu', input_shape=(2,)),
layers.Dropout(0.2),
layers.Dense(1, activation='sigmoid')
])
# Compiling the dropout model
model_dropout.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy'])
# Training the dropout model
history_dropout = model_dropout.fit(X, y, epochs=100, validation_split=0.2, verbose=0)
# Visualizing the training process with dropout
plt.plot(history_dropout.history['accuracy'], label='Train Accuracy (Dropout)')
plt.plot(history_dropout.history['val_accuracy'], label='Validation Accuracy (Dropout)')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
By adding a dropout layer to the model, we can assess whether the overfitting behavior has been alleviated.
Early Stopping
Additionally, early stopping can be employed to halt the training process when the validation accuracy ceases to improve, thereby preventing overfitting.
# Implementing early stopping
early_stopping = tf.keras.callbacks.EarlyStopping(monitor='val_accuracy', patience=10)
# Training the model with early stopping
history_early_stopping = model.fit(X, y, epochs=100, validation_split=0.2, callbacks=[early_stopping], verbose=0)
# Visualizing the training process with early stopping
plt.plot(history_early_stopping.history['accuracy'], label='Train Accuracy (Early Stopping)')
plt.plot(history_early_stopping.history['val_accuracy'], label='Validation Accuracy (Early Stopping)')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
The utilization of early stopping can help prevent overfitting by monitoring the validation accuracy and stopping the training process when necessary.
My Closing Thoughts on the Matter
In this blog post, we explored the concept of overfitting in the context of binary classification using TensorFlow 2. Through the use of synthetic data and a simple neural network model, we demonstrated the behavior of an overfitting model and examined several techniques to mitigate it, including regularization, dropout, and early stopping. By incorporating these techniques, we can enhance the model's ability to generalize to new, unseen data and improve its overall performance in binary classification tasks.
Overfitting is a common challenge in machine learning, and understanding how to identify and address it is crucial for building robust and effective models. By applying the techniques discussed in this post and continuing to explore advanced strategies for mitigating overfitting, practitioners can elevate their machine learning capabilities and drive impactful solutions in various domains.
In conclusion, overfitting is a challenge, but with the right tools and techniques, it can be effectively managed, paving the way for more accurate and reliable binary classification models.
Remember, in the world of machine learning, achieving a balance between model complexity and generalization is key. TensorFlow 2 provides a versatile platform for implementing these techniques and exploring further strategies for combating overfitting in binary classification and beyond.
Keywords: overfitting, binary classification, TensorFlow 2, regularization, dropout, early stopping, machine learning, neural network, synthetic data