Integration problem: JPA and CDI with Camel
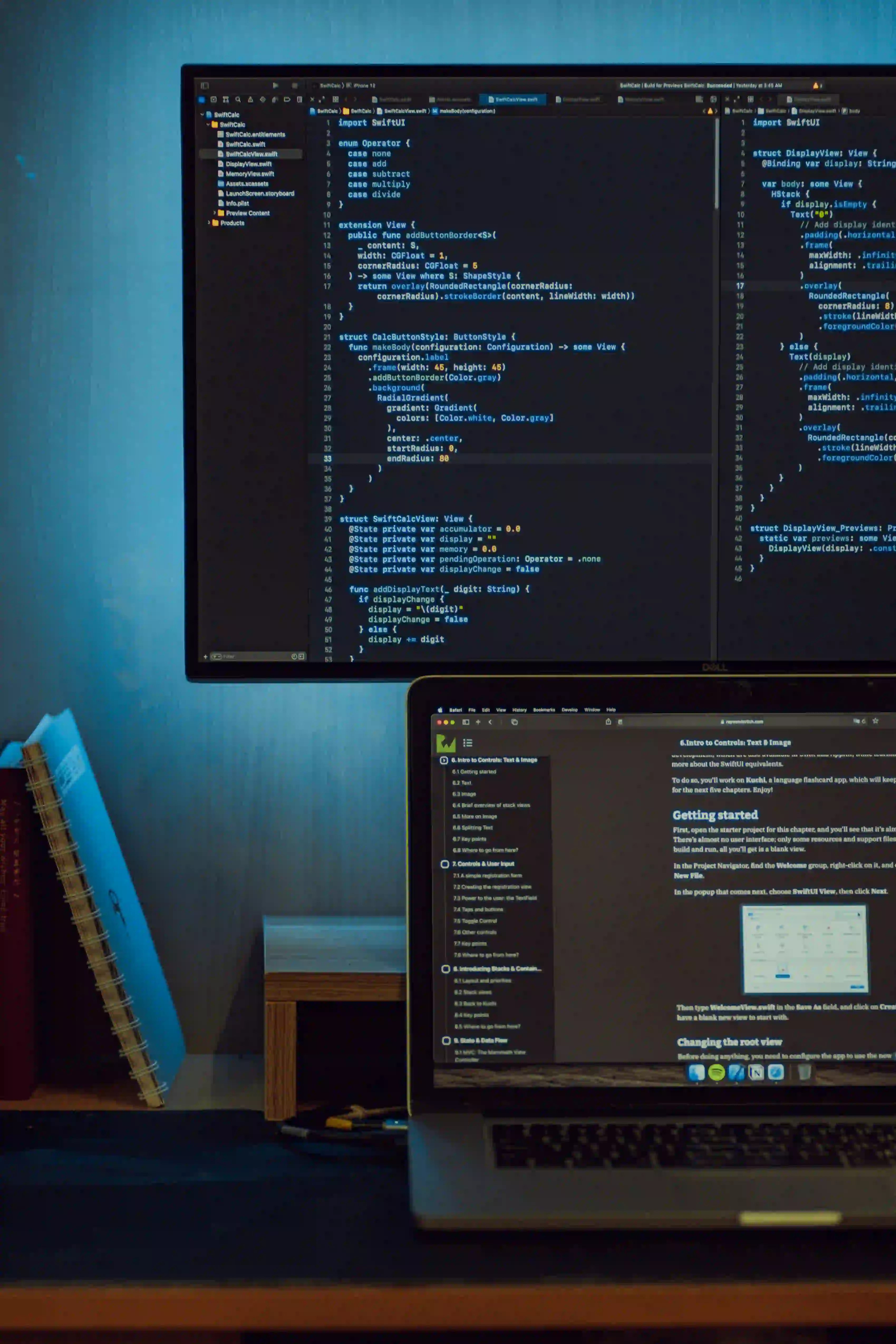
Integrating JPA and CDI with Camel
In enterprise application development, integrating various technologies is a common challenge. One such integration scenario involves combining Java Persistence API (JPA) for database interaction with Contexts and Dependency Injection (CDI) for managing bean lifecycles, along with Apache Camel for routing and mediation.
In this blog post, we will explore the seamless integration of JPA and CDI with Apache Camel to build robust and scalable enterprise applications.
Understanding the Technologies
Java Persistence API (JPA)
JPA is a Java specification for managing relational data in applications. It provides a set of APIs for performing CRUD (Create, Read, Update, Delete) operations on Java objects, which are then mapped to relational database tables.
Contexts and Dependency Injection (CDI)
CDI is a standard for dependency injection in Java EE and Jakarta EE applications. It allows beans to be managed by the container and supports injection, context management, and event firing within the application.
Apache Camel
Apache Camel is a powerful open-source integration framework that provides a rule-based routing and mediation engine. It enables developers to define routing and mediation rules in a variety of domain-specific languages, including Java, XML, and Groovy.
Integrating JPA and CDI with Apache Camel
Setting Up the Maven Dependencies
To begin, let's set up a Maven project and add the necessary dependencies for JPA, CDI, and Apache Camel. We will require the following dependencies in the pom.xml
file:
<dependencies>
<!-- JPA and CDI dependencies -->
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.apache.deltaspike.core</groupId>
<artifactId>deltaspike-core-api</artifactId>
<version>1.9.4</version>
</dependency>
<!-- Apache Camel dependencies -->
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-core</artifactId>
<version>2.24.1</version>
</dependency>
<dependency>
<groupId>org.apache.camel</groupId>
<artifactId>camel-jpa</artifactId>
<version>2.24.1</version>
</dependency>
</dependencies>
Configuring JPA and CDI
With the Maven dependencies in place, let's configure JPA and CDI. We will define a JPA entity class, a persistence unit, and a CDI bean to manage the entity. An example entity class Product
and its corresponding CDI bean ProductManager
are as follows:
Product Entity
@Entity
public class Product {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private Long id;
private String name;
private BigDecimal price;
// Getters and setters
}
ProductManager CDI Bean
@Named
@RequestScoped
public class ProductManager {
@Inject
private EntityManager entityManager;
@Transactional
public void addProduct(Product product) {
entityManager.persist(product);
}
public Product getProductById(Long id) {
return entityManager.find(Product.class, id);
}
// Other business methods
}
Integrating JPA with Apache Camel
Now, let's integrate JPA with Apache Camel to perform database operations within our Camel routes. We will use the JpaComponent
to manage JPA interactions within Camel routes. Below is an example of how to define a Camel route to add a product to the database using JPA:
from("direct:addProduct")
.transacted("PROPAGATION_REQUIRED")
.to("jpa:Product")
.log("Product added with ID: ${body.id}");
In the above route, we use the JpaComponent
to interact with the Product
entity managed by JPA. The transacted("PROPAGATION_REQUIRED")
ensures that the database operation is executed within a transaction boundary.
Leveraging CDI in Camel Routes
Additionally, we can leverage CDI beans within Camel routes to encapsulate business logic. Let's consider a Camel route that retrieves a product from the database using a CDI-managed ProductManager
:
from("direct:getProduct")
.bean(ProductManager.class, "getProductById(${body})")
.log("Retrieved product: ${body.name}, Price: ${body.price}");
In this route, the ProductManager
CDI bean is invoked to retrieve a product based on the provided ID. This demonstrates the seamless integration of CDI with Apache Camel routes.
Closing Remarks
In this blog post, we have explored the seamless integration of JPA and CDI with Apache Camel to build robust and scalable enterprise applications. By combining the power of JPA for database interactions, CDI for bean management, and Apache Camel for routing and mediation, developers can create cohesive and efficient enterprise systems.
Integrating these technologies requires a deep understanding of their capabilities and careful configuration to ensure seamless interactions. Through proper Maven setup, configuration of JPA entities and CDI beans, and integration with Apache Camel routes, developers can create powerful and maintainable enterprise applications.
By effectively integrating JPA, CDI, and Apache Camel, developers can create flexible and highly performant enterprise systems that meet the demands of modern business requirements.
In future posts, we will delve deeper into advanced integration patterns and best practices for leveraging these technologies in enterprise application development.
I hope this post has provided valuable insights into integrating JPA and CDI with Apache Camel. Happy coding!
Related Links: