Optimizing Array Processing for Coding Challenges
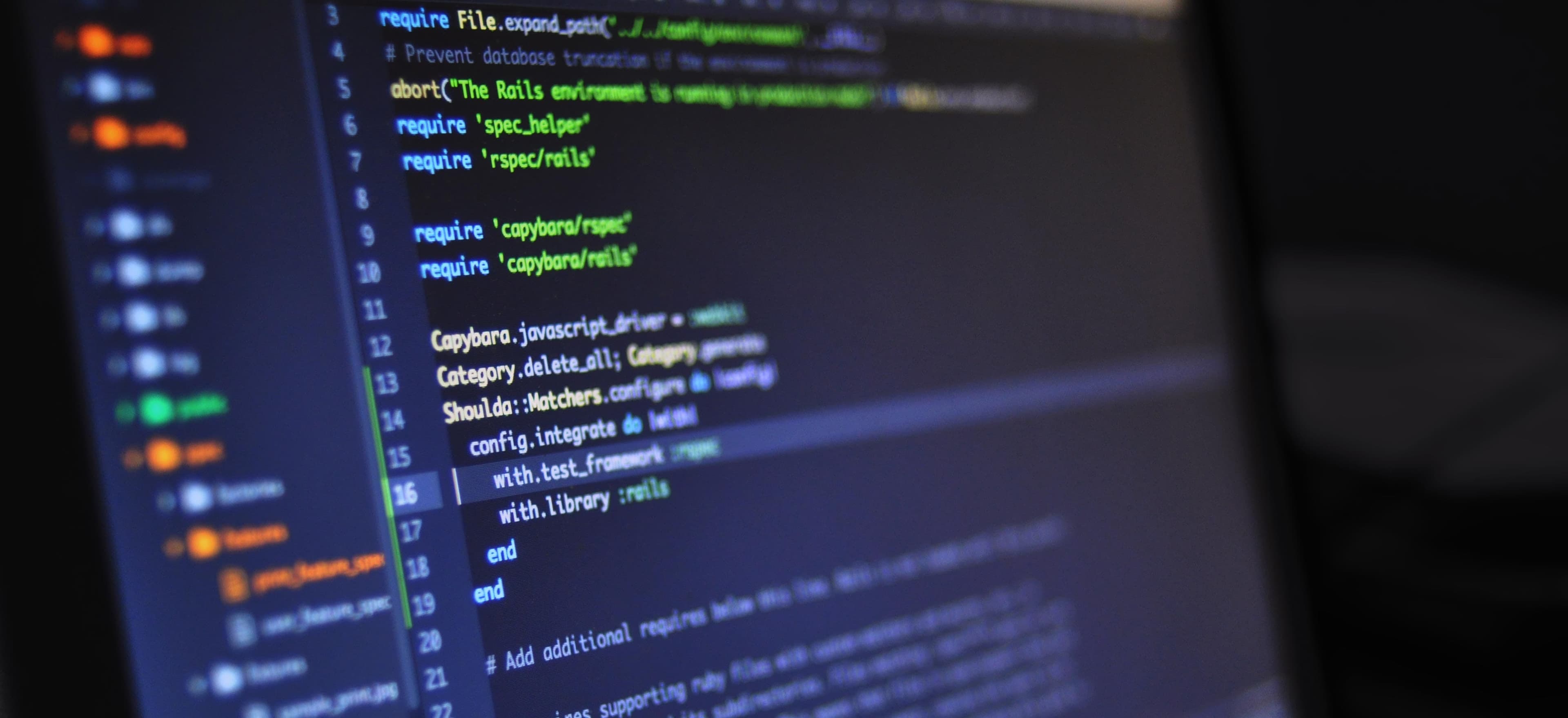
- Published on
Optimizing Array Processing for Coding Challenges
When it comes to solving coding challenges, especially those that involve processing arrays, efficiency and performance are key factors. In this blog post, we'll delve into various techniques and best practices for optimizing array processing in Java to tackle coding challenges with ease and efficiency.
Understanding the Problem
Before diving into code optimization, it's crucial to fully understand the problem at hand. Take the time to analyze the problem statement, input constraints, and expected output. This understanding will guide you in choosing the most efficient array processing approach, preventing over-engineering or underperformance.
Efficient Array Traversal
When dealing with arrays, iterating through their elements is a common operation. While traditional for-loops are effective, Java offers enhanced iterations using the for-each loop and the streams API. Let's look at an example of iterating through an array using both methods:
// Traditional for-loop
int[] arr = {1, 2, 3, 4, 5};
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
// Enhanced for-each loop
for (int num : arr) {
System.out.println(num);
}
// Streams API
Arrays.stream(arr).forEach(System.out::println);
In this example, the for-each loop and streams API provide concise and readable ways to iterate through the array. While the traditional for-loop is perfectly valid, the for-each loop and streams API offer more elegant solutions, especially for complex operations like filtering and mapping.
Why? The for-each loop and streams API simplify the syntax for array traversal and are often more expressive, leading to more maintainable and readable code.
Array Sorting and Searching
Sorting and searching are fundamental operations when working with arrays. Java provides efficient built-in methods for array sorting and searching, such as Arrays.sort()
and Arrays.binarySearch()
.
int[] arr = {3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5};
Arrays.sort(arr); // Sort the array
int index = Arrays.binarySearch(arr, 5); // Search for element 5
System.out.println("Index of 5: " + index);
Why? Utilizing built-in methods like Arrays.sort()
and Arrays.binarySearch()
saves time and guarantees optimal performance for sorting and searching operations on arrays.
Space-Time Trade-offs with Additional Data Structures
In some coding challenges, using additional data structures like HashMaps or Sets can optimize array processing. While these data structures incur space complexity, they can significantly improve time complexity and algorithm readability.
Let's consider an example where we count the frequency of elements in an array using a HashMap:
int[] arr = {1, 2, 3, 4, 3, 2, 1, 2, 3, 4, 5, 2};
Map<Integer, Integer> frequencyMap = new HashMap<>();
for (int num : arr) {
frequencyMap.put(num, frequencyMap.getOrDefault(num, 0) + 1);
}
System.out.println(frequencyMap);
In this example, the HashMap allows us to efficiently count the frequency of each element in the array, improving the algorithm's time complexity compared to nested loops or brute-force approaches.
Why? Using additional data structures can optimize array processing algorithms by trading space complexity for improved time complexity and algorithmic clarity.
Handling Edge Cases
Coding challenges often involve handling edge cases, such as empty arrays or arrays with a single element. It's crucial to consider and explicitly handle these edge cases to prevent runtime errors or incorrect results.
int[] arr = {}; // Empty array
if (arr.length == 0) {
// Handle empty array case
}
int[] singleElementArray = {5}; // Array with a single element
if (singleElementArray.length == 1) {
// Handle single element array case
}
Why? Addressing edge cases in array processing ensures the robustness and correctness of your code, preventing unexpected behaviors in coding challenge solutions.
Wrapping Up
Optimizing array processing for coding challenges in Java involves understanding the problem, choosing efficient traversal methods, leveraging built-in sorting and searching, considering space-time trade-offs with additional data structures, and handling edge cases. By following these best practices, you can tackle coding challenges efficiently and effectively.
In conclusion, efficient array processing is not only about writing code that works but also about writing code that works well. By optimizing array processing, you can enhance the performance of your code and sharpen your problem-solving skills in the world of coding challenges.
Remember, the key is not just to solve the problem, but to solve it optimally.
Start practicing these techniques with coding challenges on platforms like LeetCode and HackerRank to sharpen your skills and become proficient in optimizing array processing for real-world scenarios. Happy coding!