Managing Java Memory: A Quick Guide
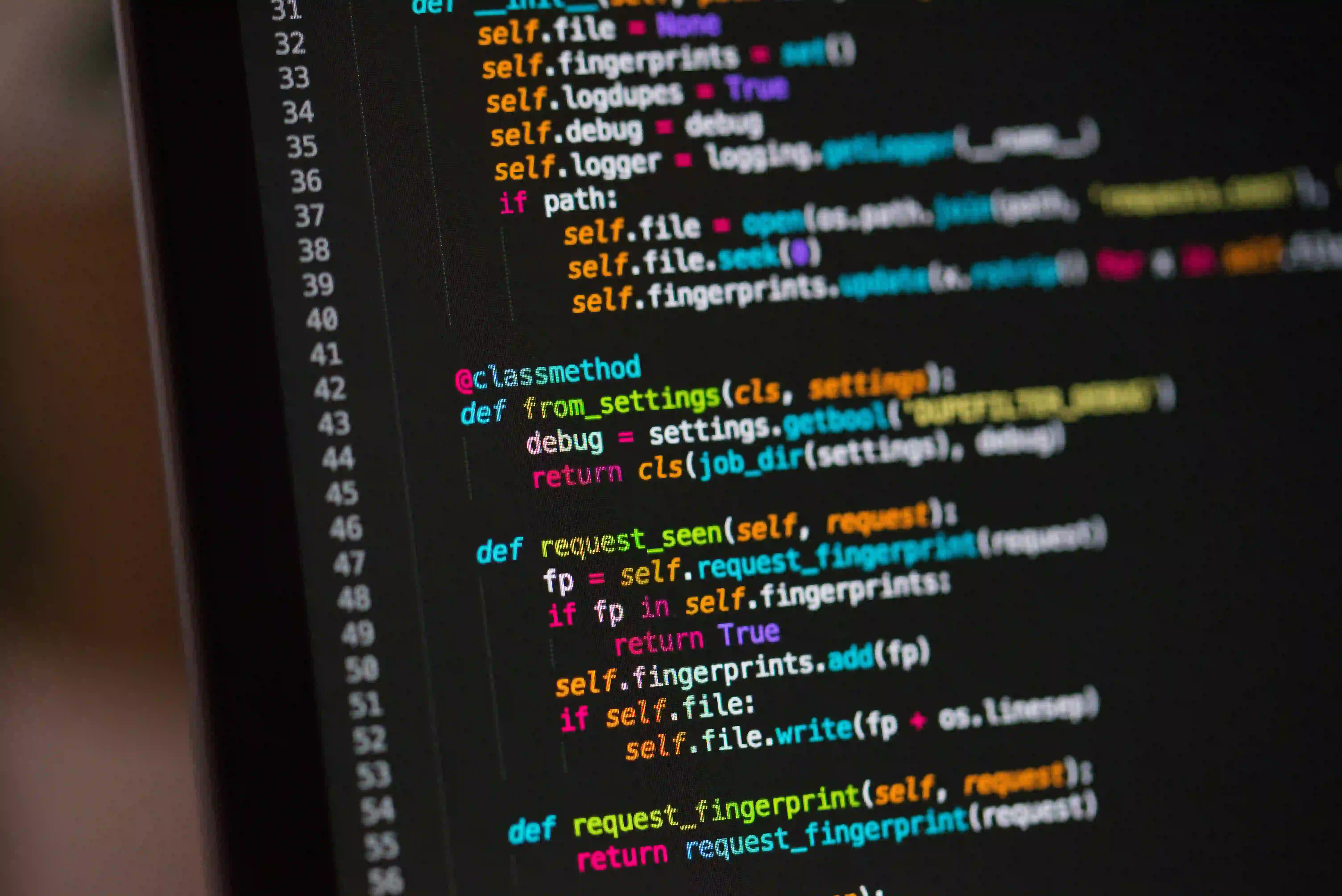
Managing Java Memory: A Quick Guide
As a Java developer, understanding and managing memory is crucial for creating efficient and performant applications. In this guide, we'll dive into the fundamentals of memory management in Java and explore best practices for optimizing memory usage.
Why Memory Management Matters
In Java, memory management is handled by the Java Virtual Machine (JVM). The JVM is responsible for allocating and deallocating memory for Java objects, and it uses different memory areas to store different types of data.
Efficient memory management is essential for several reasons:
- It directly impacts the performance of your application.
- Improper memory management can lead to memory leaks and out-of-memory errors.
- Understanding memory usage can help in optimizing code for better performance.
Understanding Java Memory Model
Before diving into memory management techniques, it's important to understand the Java memory model. The Java memory model consists of the following components:
-
Heap Memory: This is where the objects are stored. The heap is divided into the young generation and the old generation to manage object allocation and garbage collection.
-
Stack Memory: Each thread in a Java application has its own stack, which stores method calls and local variables. Unlike the heap, the stack memory is automatically managed and is not directly under developer control.
-
Permanent Generation (removed in Java 8 and later): The permanent generation was used to store metadata and class definitions. In Java 8 and later, it has been replaced by the
Metaspace
.
Best Practices for Java Memory Management
Use Proper Data Structures and Algorithms
Choosing the right data structures and algorithms can significantly reduce memory usage. For example, using a HashMap
instead of a List
for key-value pair storage can improve memory efficiency.
Limit Object Creation
Frequent object creation and garbage collection can lead to performance overhead. Reusing objects and using object pools can help reduce memory churn and improve performance.
Optimize Collection Usage
When working with collections such as List
or Map
, ensure that you understand their performance characteristics. For example, using the appropriate List
implementation (e.g., ArrayList
vs. LinkedList
) based on the usage patterns can impact memory usage and performance.
Manage Large Objects
For large objects, consider using techniques such as object splitting or lazy loading to minimize memory consumption. These techniques can help in loading only the required data into memory, thereby reducing overall memory usage.
Utilize Memory Profiling Tools
Profiling tools like VisualVM, JProfiler, or YourKit can help in identifying memory hotspots, memory leaks, and inefficient memory usage patterns. By analyzing the memory footprint of your application, you can pinpoint areas for optimization.
Handling Memory Leaks
Memory leaks occur when objects are no longer needed, but they are still referenced and not garbage collected. Using profilers and heap dump analysis tools can help identify and fix memory leaks in your application.
Memory Management in Java Example
Let's consider a simple example of managing memory in Java using a StringBuilder
instead of traditional string concatenation.
// Traditional String Concatenation
String result = "";
for (int i = 0; i < 1000; i++) {
result += "value" + i;
}
// Using StringBuilder for Memory Efficiency
StringBuilder builder = new StringBuilder();
for (int i = 0; i < 1000; i++) {
builder.append("value").append(i);
}
String result = builder.toString();
In the above example, using StringBuilder
is more memory-efficient than traditional string concatenation because it doesn't create a new string object in each iteration of the loop.
Key Takeaways
Effective memory management is essential for building high-performance Java applications. Understanding the Java memory model, employing best practices for memory management, and utilizing memory profiling tools are key strategies for optimizing memory usage in Java applications.
By following these principles and techniques, you can create Java applications that are not only memory-efficient but also deliver superior performance.
To delve deeper into the topic of memory management, you can check out Oracle's documentation on Memory Management in the Java HotSpot Virtual Machine.
Now it's time to apply these memory management techniques in your Java projects and witness the impact on performance and efficiency!