Optimizing Distributed Data Storage
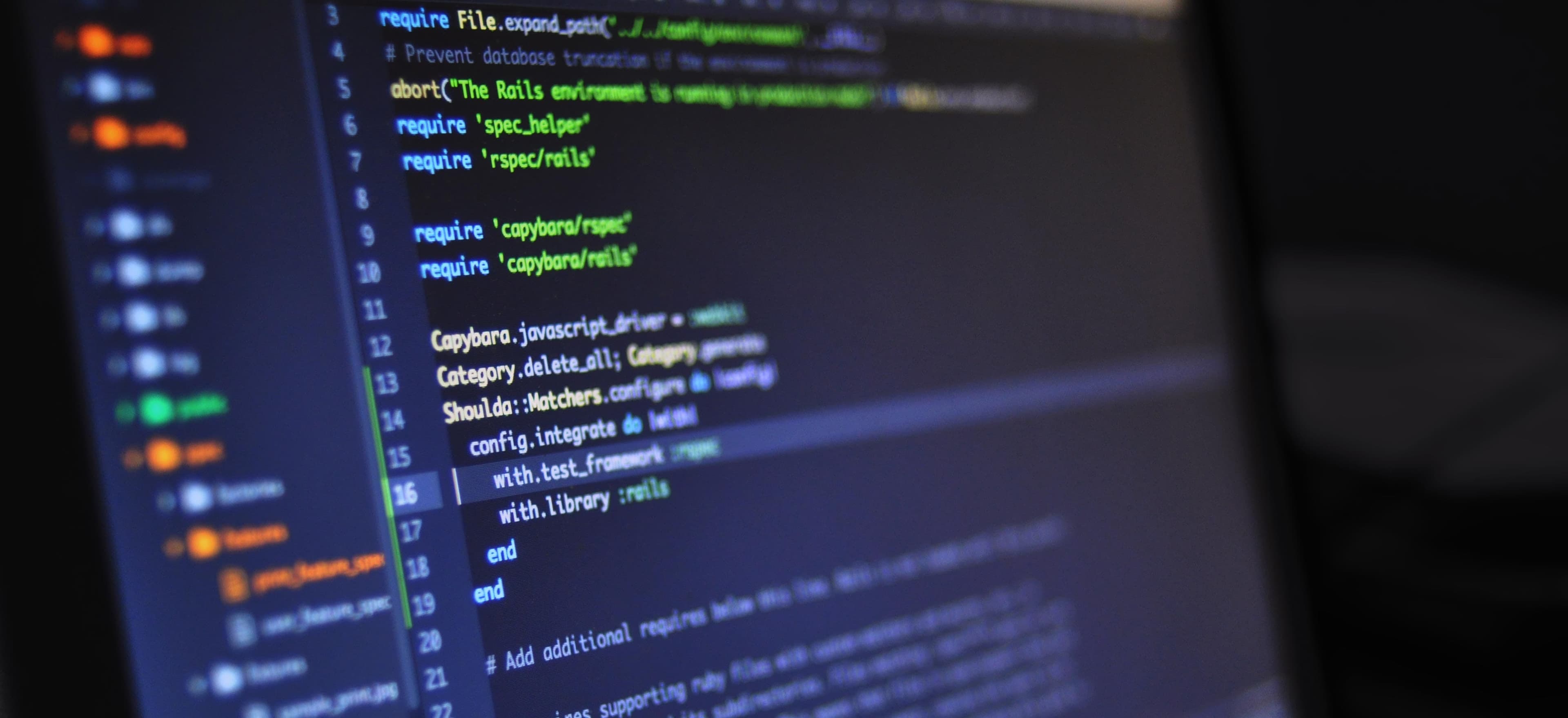
- Published on
Optimizing Distributed Data Storage in Java
In the world of distributed systems, managing and optimizing data storage is a critical factor for achieving efficiency and scalability. Java, being a versatile and widely-used programming language, provides several tools and techniques for optimizing distributed data storage. In this article, we'll explore some best practices and strategies for optimizing distributed data storage in Java applications.
Understanding Distributed Data Storage
Distributed data storage involves storing and managing data across multiple nodes or servers in a network. This approach offers several advantages such as improved fault tolerance, scalability, and performance. However, optimizing distributed data storage requires careful consideration of data distribution, retrieval, and consistency.
Choosing the Right Data Storage Technology
Selecting the appropriate data storage technology is crucial for optimizing distributed data storage. In Java, there are several popular choices such as Apache Cassandra, Redis, Apache HBase, and Apache Kafka. Each technology has its unique strengths and use cases, so it's essential to evaluate factors such as data model, consistency requirements, and scalability characteristics before making a decision.
Example: When designing a system that requires high write throughput and linear scalability, Apache Cassandra is a suitable choice due to its distributed architecture and tunable consistency levels.
Data Partitioning and Sharding
Efficient data partitioning and sharding are fundamental for optimizing distributed data storage. By distributing data across multiple nodes based on certain criteria such as key ranges, hash values, or specific attributes, it's possible to achieve better load distribution and improved query performance.
Example: In a social media platform where user data needs to be distributed evenly, a consistent hashing algorithm can be applied to shard user data across multiple database nodes, ensuring balanced data distribution and efficient retrieval.
Leveraging In-Memory Data Grids
In-memory data grids such as Hazelcast and Apache Ignite provide distributed, in-memory key-value stores that can significantly improve data access latency and throughput. These solutions are beneficial for caching frequently accessed data, executing complex queries, and maintaining data consistency across distributed nodes.
// Example of utilizing Hazelcast for distributed caching
Config config = new Config();
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance(config);
IMap<String, String> distributedMap = hazelcastInstance.getMap("distributedMap");
distributedMap.put("key", "value");
In this example, Hazelcast is utilized to create a distributed map for caching key-value pairs, offering fast and efficient access to the data.
Asynchronous Data Replication
In distributed systems, asynchronous data replication can enhance performance and fault tolerance by allowing nodes to continue processing without waiting for data to be replicated across all replicas. Java provides robust support for asynchronous programming through features such as CompletableFuture and ExecutorService, which can be leveraged to implement efficient asynchronous data replication strategies.
// Example of asynchronous data replication using CompletableFuture
CompletableFuture<Void> replicationTask = CompletableFuture.runAsync(() -> {
// Asynchronous data replication logic here
}, executorService);
By using CompletableFuture and ExecutorService, data replication tasks can be executed asynchronously, improving overall system responsiveness and scalability.
Consistency and Conflict Resolution
Ensuring data consistency is a critical aspect of optimizing distributed data storage. In a distributed environment, achieving strong consistency can be challenging due to network partitions and node failures. Techniques such as version vector clocks, conflict-free replicated data types (CRDTs), and consensus algorithms like Raft and Paxos play a vital role in maintaining data consistency and resolving conflicts.
Example: When designing a collaborative editing application, employing CRDTs for representing shared data structures can facilitate conflict-free concurrent editing by multiple users, ensuring eventual consistency without central coordination.
Monitoring and Performance Tuning
Monitoring and performance tuning are ongoing activities essential for optimizing distributed data storage in Java applications. Utilizing tools like Prometheus, Grafana, and Micrometer enables efficient monitoring of key performance metrics such as throughput, latency, and resource utilization. With these insights, targeted performance optimizations can be applied, such as index optimizations, query restructuring, and resource allocation adjustments.
Example: By using Micrometer to instrument data access operations, developers gain visibility into the performance of distributed data storage, enabling them to identify bottlenecks and fine-tune the system for improved efficiency.
Key Takeaways
Optimizing distributed data storage in Java involves a combination of selecting the right technology, implementing efficient data distribution strategies, leveraging in-memory caching, asynchronous replication, ensuring data consistency, and continuously monitoring and tuning performance. By following these best practices and utilizing the powerful tools and libraries available in the Java ecosystem, developers can build scalable and efficient distributed data storage solutions.
In conclusion, optimizing distributed data storage in Java is a multifaceted undertaking that demands careful consideration of distributed system principles, performance optimization techniques, and the utilization of appropriate tools and technologies.
By adopting a holistic approach that encompasses data distribution, storage technologies, asynchronous processing, consistency mechanisms, and performance monitoring, developers can build robust and efficient distributed data storage solutions that meet the demands of modern, scalable applications.
References:
Remember, optimizing distributed data storage is an ongoing process that requires continuous refinement according to evolving application requirements and usage patterns. With a solid understanding of the principles and tools available, developers can navigate the complexities of distributed data storage in Java with confidence and efficiency.